如何用Python做圆柱体教程
在Python中制作圆柱体涉及使用一些图形库和数学计算,如定义圆柱体的几何参数、使用matplotlib库绘制3D图形、计算圆柱体表面和体积。本文将详细介绍如何使用Python绘制和计算圆柱体相关的几何属性。
一、定义圆柱体的几何参数
在开始绘制圆柱体之前,我们需要定义其基本几何参数,如半径、高度、分段数。这些参数将帮助我们在绘制时确定圆柱体的形状和大小。
-
半径和高度
圆柱体的半径和高度是最基本的两个参数。半径决定了圆柱体的底面大小,而高度决定了圆柱体的长度。
radius = 5 # 圆柱体的半径
height = 10 # 圆柱体的高度
-
分段数
为了更精细地绘制圆柱体,我们通常会将其分成多个小段。分段数越多,绘制出来的圆柱体越平滑。
segments = 100 # 分段数
二、使用matplotlib库绘制3D图形
Python中的matplotlib库非常适合绘制各种2D和3D图形。我们可以使用它的mpl_toolkits.mplot3d
模块来绘制3D圆柱体。
-
导入必要的库
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d.art3d import Poly3DCollection
-
生成圆柱体的顶面和底面
我们可以使用numpy生成顶面和底面上的点,然后连接这些点形成圆柱体的表面。
theta = np.linspace(0, 2 * np.pi, segments)
x = radius * np.cos(theta)
y = radius * np.sin(theta)
z_top = np.ones_like(x) * height
z_bottom = np.zeros_like(x)
-
创建3D图形对象
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
-
绘制顶面和底面
ax.plot(x, y, z_top, color='b')
ax.plot(x, y, z_bottom, color='r')
-
连接顶面和底面
我们需要连接顶面和底面的对应点,以形成圆柱体的侧面。
for i in range(segments):
ax.plot([x[i], x[i]], [y[i], y[i]], [z_bottom[i], z_top[i]], color='g')
-
显示图形
plt.show()
三、计算圆柱体表面和体积
除了绘制圆柱体,我们还可以使用Python计算其表面积和体积。
-
计算表面积
圆柱体的表面积包括两个圆形的底面和一个矩形的侧面。公式如下:
base_area = 2 * np.pi * radius2
side_area = 2 * np.pi * radius * height
surface_area = base_area + side_area
print(f"圆柱体的表面积: {surface_area}")
-
计算体积
圆柱体的体积公式为:
volume = np.pi * radius2 * height
print(f"圆柱体的体积: {volume}")
四、通过Python进行圆柱体的更多操作
我们可以通过Python进行更多与圆柱体相关的操作,比如模拟物理现象、进行更复杂的几何计算等。
-
模拟圆柱体滚动
通过计算圆柱体的运动轨迹,我们可以模拟其在斜坡上的滚动。
# 假设斜坡角度为30度,重力加速度为9.8m/s^2
slope_angle = np.radians(30)
g = 9.8
计算圆柱体的加速度
acceleration = g * np.sin(slope_angle)
模拟圆柱体的滚动
time = np.linspace(0, 10, 100)
displacement = 0.5 * acceleration * time2
plt.plot(time, displacement)
plt.xlabel('时间 (s)')
plt.ylabel('位移 (m)')
plt.title('圆柱体在斜坡上的滚动')
plt.show()
-
绘制带有纹理的圆柱体
我们可以为圆柱体添加纹理,使其看起来更加真实。
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
使用meshgrid生成网格点
theta = np.linspace(0, 2 * np.pi, segments)
z = np.linspace(0, height, segments)
theta, z = np.meshgrid(theta, z)
计算对应的x和y值
x = radius * np.cos(theta)
y = radius * np.sin(theta)
绘制带有纹理的圆柱体
ax.plot_surface(x, y, z, rstride=5, cstride=5, color='c')
plt.show()
五、圆柱体在实际中的应用
-
工程设计
在工程设计中,圆柱体常用于设计管道、储罐等设备。通过Python,我们可以模拟和优化这些设备的设计。
-
3D打印
使用Python生成圆柱体的3D模型,可以用于3D打印。我们可以将生成的模型导出为STL文件,然后使用3D打印机进行打印。
from stl import mesh
定义顶面和底面上的点
points = np.array([[radius, 0, 0],
[radius * np.cos(np.pi / 3), radius * np.sin(np.pi / 3), 0],
[radius * np.cos(2 * np.pi / 3), radius * np.sin(2 * np.pi / 3), 0],
[radius * np.cos(np.pi), radius * np.sin(np.pi), 0],
[radius * np.cos(4 * np.pi / 3), radius * np.sin(4 * np.pi / 3), 0],
[radius * np.cos(5 * np.pi / 3), radius * np.sin(5 * np.pi / 3), 0],
[radius, 0, height],
[radius * np.cos(np.pi / 3), radius * np.sin(np.pi / 3), height],
[radius * np.cos(2 * np.pi / 3), radius * np.sin(2 * np.pi / 3), height],
[radius * np.cos(np.pi), radius * np.sin(np.pi), height],
[radius * np.cos(4 * np.pi / 3), radius * np.sin(4 * np.pi / 3), height],
[radius * np.cos(5 * np.pi / 3), radius * np.sin(5 * np.pi / 3), height]])
定义三角形面
faces = np.array([[0, 1, 6],
[1, 7, 6],
[1, 2, 7],
[2, 8, 7],
[2, 3, 8],
[3, 9, 8],
[3, 4, 9],
[4, 10, 9],
[4, 5, 10],
[5, 11, 10],
[5, 0, 11],
[0, 6, 11]])
创建网格
cylinder = mesh.Mesh(np.zeros(faces.shape[0], dtype=mesh.Mesh.dtype))
for i, face in enumerate(faces):
for j in range(3):
cylinder.vectors[i][j] = points[face[j], :]
保存为STL文件
cylinder.save('cylinder.stl')
-
科学计算
在科学计算中,圆柱体模型常用于模拟流体流动、热传导等现象。通过Python,我们可以对这些现象进行数值模拟和分析。
六、总结
通过本文的介绍,我们学习了如何使用Python定义圆柱体的几何参数,绘制3D圆柱体,计算其表面积和体积,并进行更多的相关操作。Python强大的计算和绘图能力,使得我们能够轻松地进行各种与圆柱体相关的工程设计和科学计算。希望本文对您有所帮助,让您在Python的世界中畅游无阻。
相关问答FAQs:
如何使用Python计算圆柱体的体积和表面积?
使用Python计算圆柱体的体积和表面积非常简单。您可以使用以下公式:
- 体积公式:V = π * r² * h,其中r是圆柱的半径,h是高度。
- 表面积公式:A = 2 * π * r * (r + h)。
可以使用Python的math库来实现这些计算。示例代码如下:
import math
def cylinder_volume(radius, height):
return math.pi * (radius ** 2) * height
def cylinder_surface_area(radius, height):
return 2 * math.pi * radius * (radius + height)
# 示例
r = 5
h = 10
print("体积:", cylinder_volume(r, h))
print("表面积:", cylinder_surface_area(r, h))
在Python中如何可视化圆柱体?
要在Python中可视化圆柱体,可以使用Matplotlib库。通过创建三维图形,您可以展示圆柱的形状和大小。以下是一个简单的示例代码:
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
def plot_cylinder(radius, height):
z = np.linspace(0, height, 100)
theta = np.linspace(0, 2 * np.pi, 100)
theta_grid, z_grid = np.meshgrid(theta, z)
x_grid = radius * np.cos(theta_grid)
y_grid = radius * np.sin(theta_grid)
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot_surface(x_grid, y_grid, z_grid, alpha=0.5)
plt.show()
# 示例
plot_cylinder(5, 10)
使用Python处理圆柱体相关的数据时,有哪些常用的库?
在处理圆柱体相关数据时,常用的Python库包括:
- NumPy:用于进行数值计算和数组操作,适合处理大量数据。
- Matplotlib:用于数据可视化,能够绘制圆柱体及其他几何形状。
- Pandas:适合数据分析和处理,能够轻松管理与圆柱体相关的各种数据。
- SciPy:提供了许多科学计算功能,可以用于更复杂的数学问题和计算。
使用这些库,可以更高效地进行圆柱体的计算和分析。
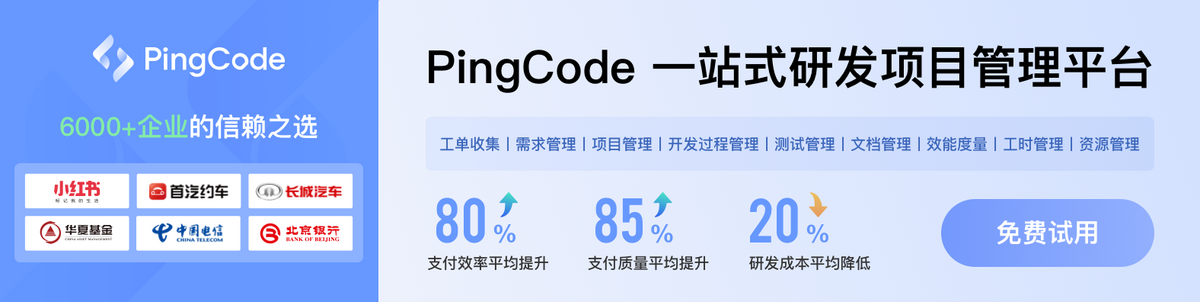