Python为字符串加引号的方法包括使用转义字符、字符串格式化、raw字符串等。其中最常用的是转义字符与raw字符串。我们可以通过在字符串中加入转义字符或者使用raw字符串来实现字符串加引号。下面详细介绍这两种方法。
一、使用转义字符
在Python中,转义字符(\)可以用来在字符串中插入特殊字符,比如双引号(")和单引号(')。这种方法可以在字符串中包含引号而不终止字符串。
1. 单引号字符串中包含单引号
如果要在一个用单引号括起来的字符串中包含单引号,可以使用转义字符(\):
string_with_single_quote = 'It\'s a sunny day'
print(string_with_single_quote) # Output: It's a sunny day
2. 双引号字符串中包含双引号
同样的,如果要在一个用双引号括起来的字符串中包含双引号,也可以使用转义字符(\):
string_with_double_quote = "He said, \"Hello!\""
print(string_with_double_quote) # Output: He said, "Hello!"
二、使用raw字符串
Python的raw字符串(原始字符串)通过在字符串前加上r或R来表示。raw字符串会忽略所有的转义字符,这样可以很方便地在字符串中包含引号而不进行转义。
1. 单引号raw字符串中包含单引号
raw_string_with_single_quote = r'It\'s a sunny day'
print(raw_string_with_single_quote) # Output: It\'s a sunny day
2. 双引号raw字符串中包含双引号
raw_string_with_double_quote = r"He said, \"Hello!\""
print(raw_string_with_double_quote) # Output: He said, \"Hello!\"
三、字符串格式化
字符串格式化是另一种将引号添加到字符串中的方法。Python提供了多种字符串格式化方式,包括%格式化、str.format()方法和f-string。
1. 使用%格式化
name = "John"
formatted_string = "He said, \"%s\"" % name
print(formatted_string) # Output: He said, "John"
2. 使用str.format()方法
name = "John"
formatted_string = "He said, \"{}\"".format(name)
print(formatted_string) # Output: He said, "John"
3. 使用f-string(Python 3.6及以上版本)
name = "John"
formatted_string = f'He said, "{name}"'
print(formatted_string) # Output: He said, "John"
四、使用三重引号
Python的三重引号(''' ''' 或 """ """)允许我们在字符串中包含多行文本,并且可以轻松地在字符串中包含单引号和双引号而不需要转义。
1. 使用三重单引号
triple_single_quote_string = '''He said, "It's a sunny day"'''
print(triple_single_quote_string) # Output: He said, "It's a sunny day"
2. 使用三重双引号
triple_double_quote_string = """He said, "It's a sunny day" """
print(triple_double_quote_string) # Output: He said, "It's a sunny day"
五、使用内置函数repr()
Python内置函数repr()可以返回一个对象的字符串表示,通常包含引号。对于字符串对象,repr()返回的字符串表示会自动包含引号。
string = "Hello, World!"
string_with_quotes = repr(string)
print(string_with_quotes) # Output: 'Hello, World!'
六、使用json.dumps()
Python的json模块提供了一个方法json.dumps(),可以将Python对象转换为JSON格式的字符串。对于字符串对象,json.dumps()会自动添加双引号。
import json
string = "Hello, World!"
json_string = json.dumps(string)
print(json_string) # Output: "Hello, World!"
七、字符串拼接
我们可以直接将引号与字符串拼接,从而在字符串中添加引号。
name = "John"
string_with_quotes = '"' + name + '"'
print(string_with_quotes) # Output: "John"
八、字符串插值
字符串插值是另一种在字符串中包含引号的方法。Python提供了多种字符串插值方式,包括旧式的%操作符和新的格式化字符串(f-string)。
1. 使用%操作符
name = "John"
string_with_quotes = '"%s"' % name
print(string_with_quotes) # Output: "John"
2. 使用f-string
name = "John"
string_with_quotes = f'"{name}"'
print(string_with_quotes) # Output: "John"
九、使用正则表达式
正则表达式(regex)是一种强大的工具,可以用来在字符串中查找和替换文本。我们可以使用正则表达式在字符串中添加引号。
import re
string = "Hello, World!"
quoted_string = re.sub(r'(\w+)', r'"\1"', string)
print(quoted_string) # Output: "Hello", "World!"
十、结合多种方法
在实际应用中,我们可能需要结合多种方法来处理复杂的字符串加引号问题。例如,我们可以结合使用字符串格式化和正则表达式来处理嵌套引号。
import re
name = "John"
sentence = 'He said, "Hello, World!"'
formatted_sentence = f'He said, "{name}"'
quoted_sentence = re.sub(r'(\w+)', r'"\1"', formatted_sentence)
print(quoted_sentence) # Output: "He" "said," ""John""
通过以上方法,我们可以在Python中灵活地给字符串加引号。不同的方法适用于不同的场景,我们可以根据实际需求选择合适的方法。无论是使用转义字符、raw字符串、字符串格式化、三重引号、内置函数repr()、json.dumps()、字符串拼接、字符串插值还是正则表达式,每种方法都有其独特的优势和适用场景。希望本文能够帮助你更好地理解和掌握Python中给字符串加引号的方法。
相关问答FAQs:
如何在Python中为字符串添加引号?
在Python中,为字符串添加引号非常简单。可以使用单引号或双引号来包围字符串。例如,使用单引号可以这样写:string_with_quotes = '这是一个字符串'
,而使用双引号则可以写成:string_with_quotes = "这是一个字符串"
。如果需要在字符串内部使用引号,可以通过转义字符(\)来实现,例如:string_with_quotes = '这是一个带\'单引号\'的字符串'
。
在Python中如何处理包含引号的字符串?
当字符串内部包含引号时,处理方式有几种。可以选择使用不同类型的引号来避免冲突,比如使用双引号包围含有单引号的字符串:string_with_quotes = "这是一个'字符串'"
。另一种方法是使用转义字符,例如:string_with_quotes = '这是一个带\'引号\'的字符串'
。此外,使用三重引号('''或""")也可以方便地处理多行字符串和内含引号的情况。
如何在Python中动态地为字符串添加引号?
在Python中,可以通过字符串格式化来动态添加引号。例如,使用f-string可以这样实现:value = "示例"; quoted_string = f'"{value}"'
,这将为value
添加双引号。如果需要单引号,可以调整为:quoted_string = f"'{value}'"
。这种方法可以灵活地将变量值嵌入到带引号的字符串中,适用于生成动态内容。
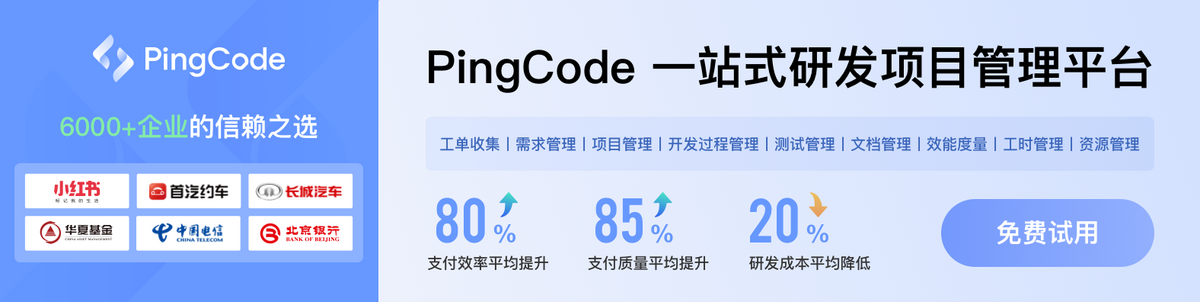