在 Python 中使用 Tkinter 实现按下按钮跳转,可以通过创建多个窗口或更新当前窗口的内容来实现。、使用 Tkinter 的 Button
小部件绑定事件处理函数来执行跳转逻辑。在本文中,我们将详细解释如何使用这些方法来实现按钮跳转。
首先,我们需要了解如何使用 Tkinter 库创建一个简单的 GUI 应用程序,并在其中添加按钮。然后,我们将探讨如何使用不同的方法在按下按钮时进行跳转。这些方法包括:创建新窗口、隐藏和显示窗口内容以及切换帧。
一、创建 Tkinter 基本窗口
在开始实现按钮跳转之前,我们需要创建一个基本的 Tkinter 窗口。以下是一个简单的 Tkinter 窗口的例子:
import tkinter as tk
def main():
root = tk.Tk()
root.title("Tkinter Button Jump Example")
root.geometry("300x200")
label = tk.Label(root, text="Welcome to the main window")
label.pack(pady=20)
button = tk.Button(root, text="Go to next window", command=lambda: go_to_next_window(root))
button.pack(pady=20)
root.mainloop()
def go_to_next_window(current_window):
current_window.destroy()
next_window()
def next_window():
new_window = tk.Tk()
new_window.title("Next Window")
new_window.geometry("300x200")
label = tk.Label(new_window, text="You are now in the next window")
label.pack(pady=20)
close_button = tk.Button(new_window, text="Close", command=new_window.destroy)
close_button.pack(pady=20)
new_window.mainloop()
if __name__ == "__main__":
main()
在这个例子中,我们创建了一个基本的 Tkinter 窗口,其中包含一个标签和一个按钮。当按下按钮时,它会调用 go_to_next_window
函数,销毁当前窗口并打开一个新窗口。
二、创建新窗口实现跳转
在 Tkinter 中,一个常见的方法是创建一个新窗口来实现跳转。以下是一个更详细的示例:
import tkinter as tk
class App:
def __init__(self, root):
self.root = root
self.root.title("Tkinter Button Jump Example")
self.root.geometry("300x200")
self.label = tk.Label(self.root, text="Welcome to the main window")
self.label.pack(pady=20)
self.button = tk.Button(self.root, text="Go to next window", command=self.go_to_next_window)
self.button.pack(pady=20)
def go_to_next_window(self):
new_window = tk.Toplevel(self.root)
new_window.title("Next Window")
new_window.geometry("300x200")
label = tk.Label(new_window, text="You are now in the next window")
label.pack(pady=20)
close_button = tk.Button(new_window, text="Close", command=new_window.destroy)
close_button.pack(pady=20)
if __name__ == "__main__":
root = tk.Tk()
app = App(root)
root.mainloop()
在这个示例中,我们使用 tk.Toplevel
创建一个新窗口,而不是销毁当前窗口。这允许我们同时拥有多个窗口,并且每个窗口都有自己的内容和按钮。
三、隐藏和显示窗口内容
另一种方法是隐藏和显示窗口内容,而不是创建新窗口。以下是一个示例:
import tkinter as tk
class App:
def __init__(self, root):
self.root = root
self.root.title("Tkinter Button Jump Example")
self.root.geometry("300x200")
self.main_frame = tk.Frame(self.root)
self.next_frame = tk.Frame(self.root)
self.label = tk.Label(self.main_frame, text="Welcome to the main window")
self.label.pack(pady=20)
self.button = tk.Button(self.main_frame, text="Go to next window", command=self.show_next_frame)
self.button.pack(pady=20)
self.main_frame.pack(fill="both", expand=True)
self.label2 = tk.Label(self.next_frame, text="You are now in the next window")
self.label2.pack(pady=20)
self.back_button = tk.Button(self.next_frame, text="Back to main window", command=self.show_main_frame)
self.back_button.pack(pady=20)
def show_next_frame(self):
self.main_frame.pack_forget()
self.next_frame.pack(fill="both", expand=True)
def show_main_frame(self):
self.next_frame.pack_forget()
self.main_frame.pack(fill="both", expand=True)
if __name__ == "__main__":
root = tk.Tk()
app = App(root)
root.mainloop()
在这个示例中,我们创建了两个帧(main_frame
和 next_frame
),并使用 pack_forget
和 pack
方法来隐藏和显示帧的内容。这种方法适用于需要在同一窗口内切换不同内容的情况。
四、使用 Frame 切换
最后,我们可以使用多个 Frame
小部件来实现窗口内容的切换。这种方法与隐藏和显示窗口内容类似,但更适合于需要管理多个页面的应用程序。以下是一个示例:
import tkinter as tk
class App:
def __init__(self, root):
self.root = root
self.root.title("Tkinter Button Jump Example")
self.root.geometry("300x200")
self.frames = {}
for F in (MainFrame, NextFrame):
page_name = F.__name__
frame = F(parent=self.root, controller=self)
self.frames[page_name] = frame
frame.grid(row=0, column=0, sticky="nsew")
self.show_frame("MainFrame")
def show_frame(self, page_name):
frame = self.frames[page_name]
frame.tkraise()
class MainFrame(tk.Frame):
def __init__(self, parent, controller):
tk.Frame.__init__(self, parent)
self.controller = controller
label = tk.Label(self, text="Welcome to the main window")
label.pack(pady=20)
button = tk.Button(self, text="Go to next window", command=lambda: controller.show_frame("NextFrame"))
button.pack(pady=20)
class NextFrame(tk.Frame):
def __init__(self, parent, controller):
tk.Frame.__init__(self, parent)
self.controller = controller
label = tk.Label(self, text="You are now in the next window")
label.pack(pady=20)
button = tk.Button(self, text="Back to main window", command=lambda: controller.show_frame("MainFrame"))
button.pack(pady=20)
if __name__ == "__main__":
root = tk.Tk()
app = App(root)
root.mainloop()
在这个示例中,我们定义了两个帧类(MainFrame
和 NextFrame
),并使用 controller
来切换帧。每个帧类都包含自己的内容和按钮,按钮的 command
参数用于调用 controller.show_frame
方法来切换帧。
总结
在本文中,我们介绍了如何使用 Tkinter 库实现按下按钮跳转的方法。我们探讨了创建新窗口、隐藏和显示窗口内容以及使用多个 Frame
小部件来实现窗口内容的切换。希望这些方法能够帮助您在开发 Tkinter 应用程序时实现所需的功能。无论是创建新窗口还是在同一窗口内切换内容,这些方法都能够提供灵活的解决方案。
相关问答FAQs:
如何在Python Tkinter中创建按钮并实现事件处理?
在Python Tkinter中,可以通过创建一个按钮并使用command
参数来绑定一个事件处理函数。当按钮被按下时,指定的函数将被调用,实现相应的功能。以下是一个简单的示例代码:
import tkinter as tk
def on_button_click():
print("按钮被点击!")
root = tk.Tk()
button = tk.Button(root, text="点击我", command=on_button_click)
button.pack()
root.mainloop()
在这个例子中,点击按钮将会在控制台输出“按钮被点击!”的信息。
如何在按钮点击时切换到新窗口?
要在按钮点击时打开一个新窗口,可以在事件处理函数中创建一个新的Toplevel
窗口。以下是实现这一功能的代码示例:
import tkinter as tk
def open_new_window():
new_window = tk.Toplevel(root)
new_window.title("新窗口")
label = tk.Label(new_window, text="这是一个新窗口")
label.pack()
root = tk.Tk()
button = tk.Button(root, text="打开新窗口", command=open_new_window)
button.pack()
root.mainloop()
当用户点击“打开新窗口”按钮时,将会弹出一个包含标签的新窗口。
如何在按钮按下后更改主窗口的内容?
可以通过在按钮的事件处理函数中直接修改主窗口中的控件属性来改变内容。以下是一个示例,展示了如何在按钮被点击时更改标签的文本:
import tkinter as tk
def change_label_text():
label.config(text="文本已更改")
root = tk.Tk()
label = tk.Label(root, text="原始文本")
label.pack()
button = tk.Button(root, text="改变文本", command=change_label_text)
button.pack()
root.mainloop()
点击“改变文本”按钮后,标签的文本将被更新为“文本已更改”。这种方式可以灵活地更新界面元素,提升用户体验。
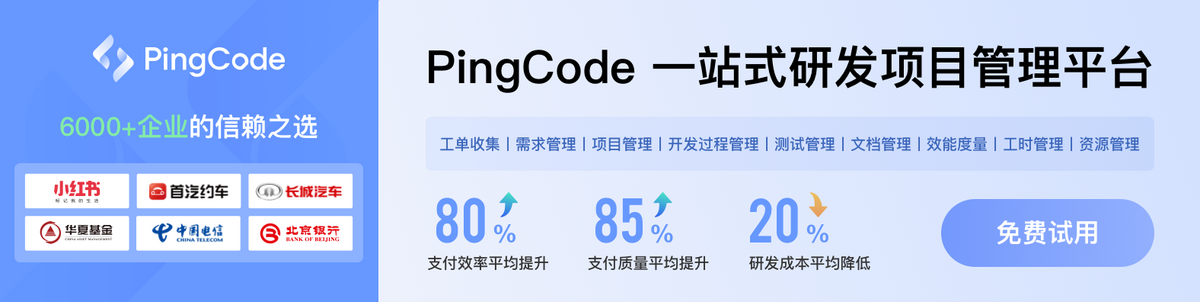