Python如何强制解压压缩包?使用shutil
模块、使用zipfile
模块、处理文件路径、处理压缩包内文件名冲突。其中,使用shutil
模块是最常见的方法之一,因为它提供了简单且易于使用的接口来处理文件和目录的操作。
使用shutil
模块来强制解压压缩包是非常方便的。shutil
模块是Python标准库的一部分,它包含了许多用于文件和目录操作的高效函数。我们可以使用shutil.unpack_archive()
函数来解压压缩包。这个函数支持多种压缩格式,包括zip、tar、gztar、bztar和xztar。通过指定目标目录,我们可以确保解压后的文件不会覆盖已有文件,或者在需要时覆盖已有文件。
一、使用shutil模块
shutil
模块提供了一个简单的方法来解压各种格式的压缩包。以下是使用shutil
模块解压压缩包的详细步骤:
1、解压zip格式的压缩包
import shutil
def extract_zip(file_path, extract_path):
try:
shutil.unpack_archive(file_path, extract_path, 'zip')
print(f"Successfully extracted {file_path} to {extract_path}")
except Exception as e:
print(f"Failed to extract {file_path}. Reason: {e}")
zip_file_path = 'example.zip'
extract_to_path = './extracted_files'
extract_zip(zip_file_path, extract_to_path)
2、解压tar格式的压缩包
import shutil
def extract_tar(file_path, extract_path):
try:
shutil.unpack_archive(file_path, extract_path, 'tar')
print(f"Successfully extracted {file_path} to {extract_path}")
except Exception as e:
print(f"Failed to extract {file_path}. Reason: {e}")
tar_file_path = 'example.tar'
extract_to_path = './extracted_files'
extract_tar(tar_file_path, extract_to_path)
二、使用zipfile模块
zipfile
模块是Python标准库的一部分,专门用于处理zip格式的压缩文件。以下是使用zipfile
模块解压压缩包的详细步骤:
1、解压zip文件
import zipfile
def extract_zip(file_path, extract_path):
with zipfile.ZipFile(file_path, 'r') as zip_ref:
zip_ref.extractall(extract_path)
print(f"Successfully extracted {file_path} to {extract_path}")
zip_file_path = 'example.zip'
extract_to_path = './extracted_files'
extract_zip(zip_file_path, extract_to_path)
2、处理文件路径
在解压压缩包时,处理文件路径是一个重要步骤。我们需要确保解压后的文件路径正确且不会覆盖已有文件。以下是处理文件路径的示例:
import os
import zipfile
def extract_zip(file_path, extract_path):
with zipfile.ZipFile(file_path, 'r') as zip_ref:
for member in zip_ref.namelist():
filename = os.path.basename(member)
source = zip_ref.open(member)
target = open(os.path.join(extract_path, filename), "wb")
with source, target:
shutil.copyfileobj(source, target)
print(f"Successfully extracted {file_path} to {extract_path}")
zip_file_path = 'example.zip'
extract_to_path = './extracted_files'
extract_zip(zip_file_path, extract_to_path)
三、处理压缩包内文件名冲突
在解压压缩包时,可能会遇到文件名冲突的情况。为了避免覆盖已有文件,我们可以在解压之前检查目标目录中是否存在同名文件,并根据需要进行重命名或跳过。以下是处理文件名冲突的示例:
import os
import zipfile
def extract_zip(file_path, extract_path):
with zipfile.ZipFile(file_path, 'r') as zip_ref:
for member in zip_ref.namelist():
filename = os.path.basename(member)
target_path = os.path.join(extract_path, filename)
if os.path.exists(target_path):
base, ext = os.path.splitext(filename)
counter = 1
while os.path.exists(target_path):
target_path = os.path.join(extract_path, f"{base}_{counter}{ext}")
counter += 1
source = zip_ref.open(member)
target = open(target_path, "wb")
with source, target:
shutil.copyfileobj(source, target)
print(f"Successfully extracted {file_path} to {extract_path}")
zip_file_path = 'example.zip'
extract_to_path = './extracted_files'
extract_zip(zip_file_path, extract_to_path)
四、处理大文件压缩包
在解压大文件压缩包时,我们可能需要处理内存和性能问题。以下是处理大文件压缩包的示例:
import os
import zipfile
def extract_large_zip(file_path, extract_path):
with zipfile.ZipFile(file_path, 'r') as zip_ref:
for member in zip_ref.namelist():
filename = os.path.basename(member)
target_path = os.path.join(extract_path, filename)
if os.path.exists(target_path):
base, ext = os.path.splitext(filename)
counter = 1
while os.path.exists(target_path):
target_path = os.path.join(extract_path, f"{base}_{counter}{ext}")
counter += 1
with zip_ref.open(member) as source, open(target_path, "wb") as target:
shutil.copyfileobj(source, target, 1024*1024) # 1MB buffer size
print(f"Successfully extracted {file_path} to {extract_path}")
zip_file_path = 'large_example.zip'
extract_to_path = './extracted_files'
extract_large_zip(zip_file_path, extract_to_path)
五、使用第三方库
除了标准库,Python还有许多第三方库可以用于解压压缩包。例如,pyunpack
和patoolib
库可以处理多种格式的压缩包。以下是使用pyunpack
库解压压缩包的示例:
1、安装pyunpack和patool
pip install pyunpack patool
2、解压压缩包
from pyunpack import Archive
def extract_archive(file_path, extract_path):
try:
Archive(file_path).extractall(extract_path)
print(f"Successfully extracted {file_path} to {extract_path}")
except Exception as e:
print(f"Failed to extract {file_path}. Reason: {e}")
archive_file_path = 'example.rar'
extract_to_path = './extracted_files'
extract_archive(archive_file_path, extract_to_path)
六、总结
在这篇文章中,我们探讨了多种使用Python解压压缩包的方法,包括使用shutil
模块、zipfile
模块、处理文件路径、处理文件名冲突、处理大文件压缩包以及使用第三方库。每种方法都有其优点和适用场景,开发者可以根据具体需求选择合适的方法来解压压缩包。希望这些示例代码和详细步骤能够帮助你在实际项目中顺利解压压缩包。
相关问答FAQs:
如何在Python中处理不同格式的压缩文件?
在Python中,可以使用内置的zipfile
模块处理ZIP格式的压缩文件,使用tarfile
模块处理TAR格式的压缩文件。如果需要解压缩其他格式的文件,比如RAR文件,可以考虑使用第三方库,如rarfile
。确保在处理之前安装相关的库,并根据文件的格式选择合适的解压方式。
遇到“文件损坏”或“解压失败”时该如何处理?
如果在解压过程中遇到文件损坏的提示,首先可以尝试使用压缩软件修复文件,或重新下载压缩包。如果确认文件完好无损,可以检查是否使用了正确的解压方法。确保使用的解压工具支持该文件类型,或者尝试更新Python版本和相关库,以获得更好的兼容性。
如何选择合适的库来解压缩文件?
选择合适的库通常取决于压缩文件的格式和项目的需求。对于常见的ZIP和TAR文件,Python的标准库zipfile
和tarfile
已经足够。如果需要处理更复杂的格式,例如7z或RAR,可以考虑使用py7zr
或rarfile
等第三方库。在选择时,也要考虑库的维护情况和社区支持,以确保在使用过程中遇到问题时能够得到解决。
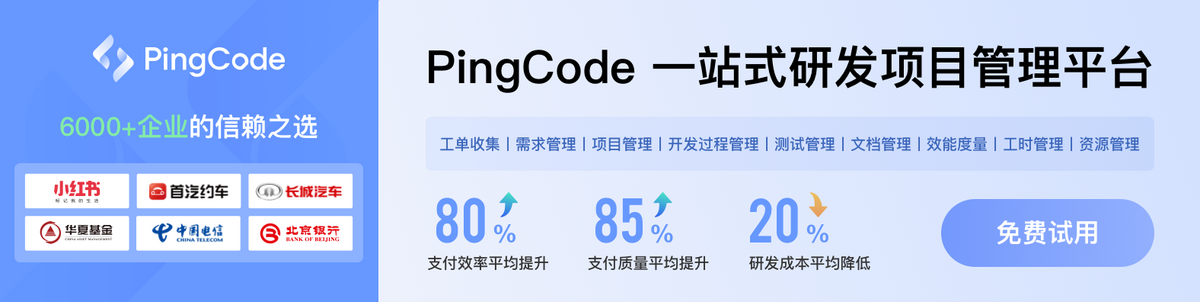