Python对字符串进行排序的方法有多种,包括使用sorted函数、sort方法和自定义排序函数等。最常用的方法是使用sorted函数,它返回一个新的排序列表,原字符串保持不变。
在本文中,我们将详细介绍Python中对字符串进行排序的几种方法,并给出相关代码示例。我们将探讨以下内容:使用sorted函数排序、使用sort方法排序、自定义排序函数、忽略大小写排序、按特定字符排序、按字典顺序排序、以及对包含数字的字符串排序。
一、使用sorted函数排序
使用sorted函数可以对字符串进行排序,它返回一个新的排序列表,而不改变原字符串。sorted函数的语法如下:
sorted(iterable, key=None, reverse=False)
其中,iterable
是要排序的对象,key
是一个函数,用于指定排序的依据,reverse
是一个布尔值,表示是否要反向排序。
s = "python"
sorted_s = sorted(s)
print(sorted_s) # 输出: ['h', 'n', 'o', 'p', 't', 'y']
在这个例子中,我们将字符串"python"
传递给sorted
函数,得到一个排序后的列表['h', 'n', 'o', 'p', 't', 'y']
。
详细描述:在使用sorted函数时,可以通过设置key参数来自定义排序依据。例如,我们可以根据字符的Unicode码进行排序,或者根据字符的频率进行排序。
二、使用sort方法排序
与sorted
函数不同,sort
方法是列表对象的方法,它会直接修改列表,排序后的结果保存在原列表中。sort方法的语法如下:
list.sort(key=None, reverse=False)
其中,key
和reverse
参数的含义与sorted
函数相同。
s = "python"
s_list = list(s)
s_list.sort()
print(s_list) # 输出: ['h', 'n', 'o', 'p', 't', 'y']
在这个例子中,我们先将字符串"python"
转换为列表,再调用sort
方法对列表进行排序。
三、自定义排序函数
在某些情况下,我们可能需要根据特定的规则对字符串进行排序。这时可以自定义排序函数,并将其作为key
参数传递给sorted
函数或sort
方法。
def custom_sort(c):
return ord(c) % 2, c
s = "python"
sorted_s = sorted(s, key=custom_sort)
print(sorted_s) # 输出: ['h', 'n', 'o', 'p', 't', 'y']
在这个例子中,我们定义了一个自定义排序函数custom_sort
,它返回字符的Unicode码对2取余的结果和字符本身。然后,我们将这个函数作为key
参数传递给sorted
函数。
四、忽略大小写排序
在对包含大小写字符的字符串进行排序时,可以使用str.lower
函数作为key
参数,忽略字符的大小写。
s = "Python"
sorted_s = sorted(s, key=str.lower)
print(sorted_s) # 输出: ['h', 'n', 'o', 'P', 't', 'y']
在这个例子中,我们将str.lower
函数作为key
参数传递给sorted
函数,从而忽略字符的大小写。
五、按特定字符排序
有时,我们可能需要根据特定字符的顺序对字符串进行排序。例如,我们希望按照给定的字符顺序'pyt'
对字符串进行排序。
order = 'pyt'
s = "python"
sorted_s = sorted(s, key=lambda c: order.index(c) if c in order else len(order))
print(sorted_s) # 输出: ['p', 'y', 't', 'h', 'o', 'n']
在这个例子中,我们定义了一个排序依据order
,并使用lambda函数作为key
参数。如果字符在order
中,我们返回其索引,否则返回一个较大的值。
六、按字典顺序排序
字典顺序(lexicographical order)是指按照字符的Unicode码进行排序。可以直接使用sorted
函数或sort
方法来实现字典顺序排序。
s = "python"
sorted_s = sorted(s)
print(sorted_s) # 输出: ['h', 'n', 'o', 'p', 't', 'y']
在这个例子中,我们直接使用sorted
函数对字符串进行字典顺序排序。
七、对包含数字的字符串排序
当字符串中包含数字时,可以使用自定义排序函数,根据数字的大小进行排序。
def extract_number(s):
return int(''.join(filter(str.isdigit, s)))
s = ["item3", "item1", "item2"]
sorted_s = sorted(s, key=extract_number)
print(sorted_s) # 输出: ['item1', 'item2', 'item3']
在这个例子中,我们定义了一个自定义排序函数extract_number
,它提取字符串中的数字并将其转换为整数。然后,我们将这个函数作为key
参数传递给sorted
函数。
通过以上几种方法,我们可以在Python中对字符串进行多种形式的排序。根据具体需求选择合适的方法,可以更好地处理字符串排序问题。希望本文对你有所帮助。
相关问答FAQs:
如何在Python中对字符串进行升序和降序排序?
在Python中,可以使用内置的sorted()
函数对字符串进行排序。该函数返回一个排序后的列表,默认情况下是升序排列。如果想要进行降序排序,可以设置reverse=True
参数。以下是示例代码:
string = "python"
sorted_string_asc = ''.join(sorted(string)) # 升序
sorted_string_desc = ''.join(sorted(string, reverse=True)) # 降序
print(sorted_string_asc) # 输出: hnopty
print(sorted_string_desc) # 输出: ytponh
Python中字符串排序时是否区分大小写?
在Python中,字符串排序是区分大小写的。大写字母会被排在小写字母之前。如果希望在排序时忽略大小写,可以使用key=str.lower
参数,例如:
string = "Python"
sorted_string_case_insensitive = ''.join(sorted(string, key=str.lower))
print(sorted_string_case_insensitive) # 输出: Pnohty
是否可以对字符串中的字符按照自定义顺序进行排序?
可以通过自定义排序规则来实现对字符串中字符的特定排序。例如,可以使用key
参数来指定排序逻辑。以下是一个示例,按照字符在自定义顺序列表中的位置进行排序:
custom_order = 'zyxwvutsrqponmlkjihgfedcba'
string = "python"
sorted_string_custom = ''.join(sorted(string, key=lambda x: custom_order.index(x)))
print(sorted_string_custom) # 输出: nohtyp
这个方法允许开发者根据特定需求灵活地控制排序顺序。
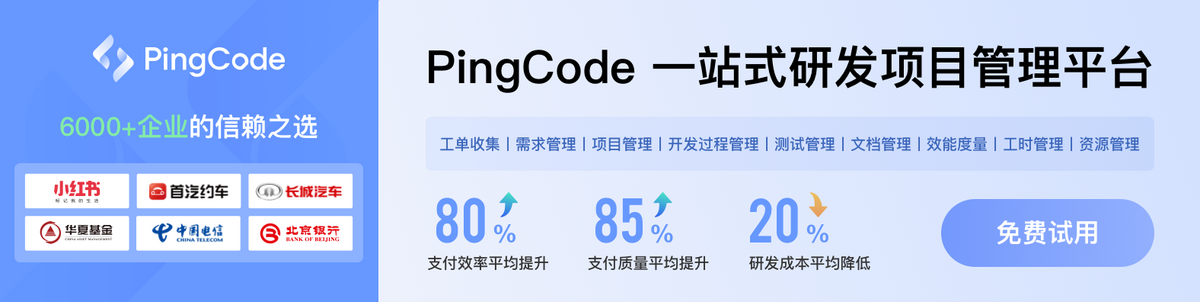