如何用Python写一个邮箱
用Python编写一个邮箱的核心要点包括:SMTP协议、IMAP协议、邮件格式、收发邮件、处理附件、错误处理。在这些要点中,SMTP协议是实现邮件发送的关键。SMTP,即简单邮件传输协议,是一种用于电子邮件传输的协议,通常用于在服务器之间发送电子邮件。通过SMTP协议,我们可以将邮件从客户端发送到服务器,再由服务器将邮件转发到收件人的服务器上。
下面将详细描述如何使用Python实现一个简单的邮箱功能,并逐步解释实现过程中的每个步骤。
一、SMTP协议发送邮件
SMTP协议(Simple Mail Transfer Protocol)是用来传送邮件的协议。Python中可以通过smtplib模块来使用SMTP协议发送邮件。
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def send_email(smtp_server, port, sender_email, sender_password, receiver_email, subject, body):
msg = MIMEMultipart()
msg['From'] = sender_email
msg['To'] = receiver_email
msg['Subject'] = subject
msg.attach(MIMEText(body, 'plain'))
with smtplib.SMTP(smtp_server, port) as server:
server.starttls()
server.login(sender_email, sender_password)
server.sendmail(sender_email, receiver_email, msg.as_string())
smtp_server = 'smtp.example.com'
port = 587
sender_email = 'your_email@example.com'
sender_password = 'your_password'
receiver_email = 'receiver_email@example.com'
subject = 'Test Email'
body = 'This is a test email sent from Python.'
send_email(smtp_server, port, sender_email, sender_password, receiver_email, subject, body)
二、IMAP协议接收邮件
IMAP协议(Internet Message Access Protocol)用于从服务器读取邮件。Python中可以通过imaplib模块来使用IMAP协议接收邮件。
import imaplib
import email
def receive_email(imap_server, email_user, email_pass):
mail = imaplib.IMAP4_SSL(imap_server)
mail.login(email_user, email_pass)
mail.select('inbox')
status, data = mail.search(None, 'ALL')
mail_ids = data[0].split()
for mail_id in mail_ids:
status, data = mail.fetch(mail_id, '(RFC822)')
msg = email.message_from_bytes(data[0][1])
email_from = msg['from']
email_subject = msg['subject']
email_body = msg.get_payload(decode=True)
print(f'From: {email_from}\nSubject: {email_subject}\n\n{email_body.decode("utf-8")}')
imap_server = 'imap.example.com'
email_user = 'your_email@example.com'
email_pass = 'your_password'
receive_email(imap_server, email_user, email_pass)
三、邮件格式处理
邮件格式通常包括文本、HTML和附件等内容。使用Python的email模块可以方便地处理这些格式。下面的示例展示了如何发送包含HTML内容和附件的邮件。
from email.mime.base import MIMEBase
from email import encoders
def send_email_with_attachment(smtp_server, port, sender_email, sender_password, receiver_email, subject, body, attachment_path):
msg = MIMEMultipart()
msg['From'] = sender_email
msg['To'] = receiver_email
msg['Subject'] = subject
msg.attach(MIMEText(body, 'html'))
with open(attachment_path, 'rb') as attachment:
part = MIMEBase('application', 'octet-stream')
part.set_payload(attachment.read())
encoders.encode_base64(part)
part.add_header('Content-Disposition', f'attachment; filename={attachment_path}')
msg.attach(part)
with smtplib.SMTP(smtp_server, port) as server:
server.starttls()
server.login(sender_email, sender_password)
server.sendmail(sender_email, receiver_email, msg.as_string())
attachment_path = 'path_to_attachment'
send_email_with_attachment(smtp_server, port, sender_email, sender_password, receiver_email, subject, body, attachment_path)
四、处理附件
处理附件包括解析接收到的邮件中的附件并保存到本地。以下示例展示了如何在接收邮件时处理附件。
def save_attachment(msg, download_folder="/path/to/download"):
for part in msg.walk():
if part.get_content_maintype() == 'multipart':
continue
if part.get('Content-Disposition') is None:
continue
filename = part.get_filename()
if bool(filename):
filepath = os.path.join(download_folder, filename)
with open(filepath, 'wb') as f:
f.write(part.get_payload(decode=True))
def receive_email_with_attachment(imap_server, email_user, email_pass, download_folder):
mail = imaplib.IMAP4_SSL(imap_server)
mail.login(email_user, email_pass)
mail.select('inbox')
status, data = mail.search(None, 'ALL')
mail_ids = data[0].split()
for mail_id in mail_ids:
status, data = mail.fetch(mail_id, '(RFC822)')
msg = email.message_from_bytes(data[0][1])
save_attachment(msg, download_folder)
receive_email_with_attachment(imap_server, email_user, email_pass, '/path/to/download')
五、错误处理
在实际应用中,错误处理是必不可少的。通过捕获和处理异常,可以提高程序的健壮性和用户体验。
def send_email_with_error_handling(smtp_server, port, sender_email, sender_password, receiver_email, subject, body):
try:
msg = MIMEMultipart()
msg['From'] = sender_email
msg['To'] = receiver_email
msg['Subject'] = subject
msg.attach(MIMEText(body, 'plain'))
with smtplib.SMTP(smtp_server, port) as server:
server.starttls()
server.login(sender_email, sender_password)
server.sendmail(sender_email, receiver_email, msg.as_string())
print("Email sent successfully.")
except smtplib.SMTPException as e:
print(f"Error: unable to send email. Exception: {e}")
send_email_with_error_handling(smtp_server, port, sender_email, sender_password, receiver_email, subject, body)
总结
通过上述内容,我们详细介绍了如何用Python实现一个基本的邮箱功能,包括发送邮件、接收邮件、处理邮件格式和附件以及进行错误处理。SMTP协议是实现邮件发送的关键,通过smtplib模块,我们可以方便地实现邮件发送功能。同样地,通过imaplib模块和email模块,我们可以实现接收邮件和处理邮件内容的功能。在实际应用中,良好的错误处理和日志记录也是非常重要的,可以提高程序的健壮性和用户体验。
相关问答FAQs:
如何用Python发送电子邮件?
使用Python发送电子邮件通常会用到smtplib
库。你可以通过连接到SMTP服务器,配置发件人、收件人、主题和邮件内容来发送邮件。以下是一个简单的示例代码:
import smtplib
from email.mime.text import MIMEText
# 邮件内容
subject = "测试邮件"
body = "这是一封测试邮件。"
msg = MIMEText(body)
msg['Subject'] = subject
msg['From'] = "你的邮箱@example.com"
msg['To'] = "收件人邮箱@example.com"
# 发送邮件
with smtplib.SMTP('smtp.example.com', 587) as server:
server.starttls()
server.login("你的邮箱@example.com", "你的密码")
server.send_message(msg)
确保你将示例中的邮箱和SMTP服务器地址替换为你的实际信息。
使用Python发送邮件时需要注意哪些安全性问题?
在发送邮件时,重要的是确保你的密码和其他敏感信息的安全。建议使用环境变量存储邮箱密码,避免将其硬编码在代码中。此外,许多邮件服务提供商(如Gmail)会要求启用“允许不够安全的应用”,或使用应用专用密码来增强安全性。
可以用Python发送带有附件的邮件吗?
绝对可以。使用email
模块中的MIMEBase
和encoders
可以轻松实现附件功能。以下是一个基本示例,展示如何添加附件:
from email.mime.base import MIMEBase
from email import encoders
# 创建邮件对象
msg = MIMEMultipart()
msg['From'] = "你的邮箱@example.com"
msg['To'] = "收件人邮箱@example.com"
msg['Subject'] = "带有附件的邮件"
# 邮件正文
msg.attach(MIMEText("这是带有附件的邮件。"))
# 添加附件
filename = "附件.txt"
with open(filename, "rb") as attachment:
part = MIMEBase("application", "octet-stream")
part.set_payload(attachment.read())
encoders.encode_base64(part)
part.add_header("Content-Disposition", f"attachment; filename={filename}")
msg.attach(part)
# 发送邮件代码与上述相同
通过这些代码示例和注意事项,你将能够使用Python高效地发送电子邮件。
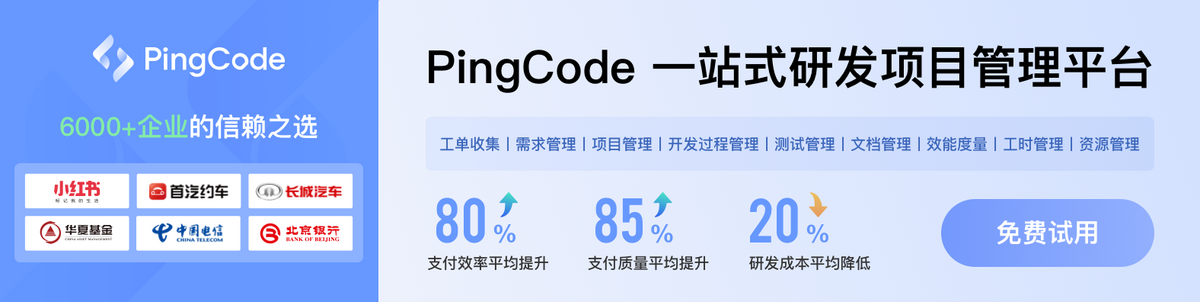