在Python中去掉行尾的换行符,可以使用strip()、rstrip()、replace()方法。 这些方法各有其适用的场景和特点,其中最常用的是 rstrip()
方法,因为它只移除字符串末尾的空白字符,包括换行符。下面将详细介绍这几种方法及其使用场景。
一、使用strip()方法
strip()
方法可以移除字符串首尾的空白字符,包括空格、制表符以及换行符。这个方法适用于需要同时去除字符串开头和结尾空白字符的情况。
string_with_newline = "Hello, World!\n"
cleaned_string = string_with_newline.strip()
print(cleaned_string) # 输出: "Hello, World!"
在这个例子中,strip()
方法移除了字符串首尾的换行符和其他空白字符。如果字符串中间有空白字符,它们将不会被移除。
二、使用rstrip()方法
rstrip()
方法只移除字符串末尾的空白字符,因此它是专门用于去掉行尾换行符的理想选择。
string_with_newline = "Hello, World!\n"
cleaned_string = string_with_newline.rstrip()
print(cleaned_string) # 输出: "Hello, World!"
在这个例子中,rstrip()
方法仅移除了字符串末尾的换行符,而保留了字符串开头和中间的所有字符。
三、使用replace()方法
replace()
方法用于替换字符串中的子字符串。虽然它不如 strip()
和 rstrip()
方法直接,但也可以用于移除行尾的换行符。
string_with_newline = "Hello, World!\n"
cleaned_string = string_with_newline.replace("\n", "")
print(cleaned_string) # 输出: "Hello, World!"
在这个例子中,replace()
方法将所有的换行符替换为空字符串,从而移除了行尾的换行符。这种方法适用于需要移除字符串中所有换行符的情况。
四、综合使用场景
1、处理多行字符串
在处理包含多行的字符串时,可能需要逐行移除换行符。可以结合 splitlines()
方法和 strip()
或 rstrip()
方法来实现。
multi_line_string = """Hello, World!
This is a test.
Python is great!\n"""
lines = multi_line_string.splitlines()
cleaned_lines = [line.rstrip() for line in lines]
cleaned_string = "\n".join(cleaned_lines)
print(cleaned_string)
这个例子展示了如何逐行处理多行字符串,并移除每行末尾的换行符。
2、处理文件读取
在处理文件读取时,通常需要移除每行末尾的换行符。可以结合 readlines()
方法和 strip()
或 rstrip()
方法来实现。
with open("example.txt", "r") as file:
lines = file.readlines()
cleaned_lines = [line.rstrip() for line in lines]
for line in cleaned_lines:
print(line)
这个例子展示了如何在读取文件时,移除每行末尾的换行符,并逐行输出处理后的内容。
五、总结
在Python中去掉行尾的换行符有多种方法,包括 strip()
、rstrip()
和 replace()
方法。选择适合的方法取决于具体的应用场景。rstrip()
方法是最常用的,因为它专门用于移除字符串末尾的空白字符。 在处理多行字符串或文件读取时,可以结合其他方法进行灵活处理。希望这篇文章能帮助你更好地理解和使用这些方法。
相关问答FAQs:
如何在Python中去掉字符串末尾的换行符?
在Python中,可以使用rstrip()
方法去掉字符串末尾的换行符。这种方法不仅可以去掉换行符,还可以去掉其他空白字符。例如,my_string.rstrip()
将返回一个去除了所有尾部空白字符的字符串。
使用strip()和rstrip()的区别是什么?strip()
方法会去掉字符串两端的空白字符,而rstrip()
仅去掉字符串末尾的空白字符。如果您只需要删除行尾的换行符,rstrip()
是更合适的选择。例如,my_string.strip()
会去掉字符串首尾的换行符和空格。
在读取文件时如何处理行尾的换行符?
在读取文件时,通常会遇到每行末尾都有换行符的情况。可以在读取文件时使用strip()
或rstrip()
方法来处理每一行。例如,使用for line in file: line = line.rstrip()
可以确保在处理每一行时去掉行尾的换行符,这样可以使后续操作更加简洁。
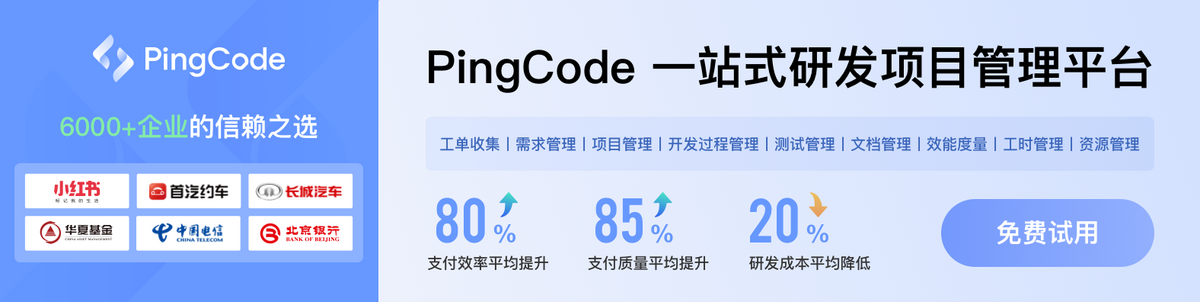