如何让Python设置使用时间
使用Python设置使用时间的主要方法有:使用time模块、使用datetime模块、使用schedule模块、结合线程处理。这些方法各有优劣,适用于不同的需求场景。其中,使用time模块是最基础的方法,可以完成简单的时间操作。接下来,我们详细介绍如何使用time模块来设置使用时间。
使用time模块的方法:time模块是Python内置的标准库,提供了多种时间相关的函数,可以进行时间延迟、获取当前时间戳等操作。time.sleep()函数可以让程序暂停执行指定的秒数,非常适合用来控制程序的执行时间。
一、TIME模块
time模块是Python中处理时间的基础模块。它提供了多种时间操作方法,包括获取当前时间、格式化时间、暂停程序等。以下是使用time模块的一些常见方法:
1.1 获取当前时间戳
使用time模块,可以获取当前时间的时间戳。时间戳是从1970年1月1日00:00:00 UTC到当前时间的秒数。
import time
current_time = time.time()
print(f"当前时间戳: {current_time}")
1.2 格式化时间
time模块还提供了将时间戳转换为可读格式的方法。例如,可以使用time.strftime()函数将时间戳转换为指定格式的时间字符串。
import time
current_time = time.time()
formatted_time = time.strftime("%Y-%m-%d %H:%M:%S", time.localtime(current_time))
print(f"格式化时间: {formatted_time}")
1.3 暂停程序
time.sleep()函数可以使程序暂停执行指定的秒数。这在需要控制程序执行时间时非常有用。
import time
print("程序开始...")
time.sleep(5) # 暂停5秒
print("程序结束。")
二、DATETIME模块
datetime模块是Python中处理日期和时间的高级模块。相比time模块,datetime模块提供了更多的功能和更友好的接口。以下是使用datetime模块的一些常见方法:
2.1 获取当前日期和时间
使用datetime模块,可以获取当前的日期和时间。
from datetime import datetime
current_datetime = datetime.now()
print(f"当前日期和时间: {current_datetime}")
2.2 格式化日期和时间
datetime模块还提供了将日期和时间格式化为指定字符串的方法。例如,可以使用strftime()函数将日期和时间格式化为指定格式的字符串。
from datetime import datetime
current_datetime = datetime.now()
formatted_datetime = current_datetime.strftime("%Y-%m-%d %H:%M:%S")
print(f"格式化日期和时间: {formatted_datetime}")
2.3 日期和时间的加减
datetime模块还支持日期和时间的加减操作。例如,可以使用timedelta对象来表示时间间隔,并进行日期和时间的加减运算。
from datetime import datetime, timedelta
current_datetime = datetime.now()
one_week_later = current_datetime + timedelta(weeks=1)
print(f"一周后的日期和时间: {one_week_later}")
三、SCHEDULE模块
schedule模块是一个轻量级的任务调度库,适用于需要定时执行任务的场景。以下是使用schedule模块的一些常见方法:
3.1 安装schedule模块
在使用schedule模块之前,需要先安装它。可以使用pip进行安装:
pip install schedule
3.2 定时任务
使用schedule模块,可以轻松地设置定时任务。例如,每隔5秒执行一次任务:
import schedule
import time
def job():
print("定时任务执行中...")
schedule.every(5).seconds.do(job)
while True:
schedule.run_pending()
time.sleep(1)
3.3 定时任务的多种频率
schedule模块支持多种频率的定时任务,例如每天、每小时、每分钟等。
import schedule
import time
def job():
print("每天的定时任务执行中...")
schedule.every().day.at("10:30").do(job)
while True:
schedule.run_pending()
time.sleep(1)
四、结合线程处理
在某些情况下,可能需要在设置使用时间的同时执行其他任务。此时,可以使用Python的线程模块threading来实现多任务处理。
4.1 创建线程
可以使用threading模块创建和启动线程。例如,创建一个新的线程来执行定时任务:
import threading
import time
def task():
while True:
print("定时任务执行中...")
time.sleep(5)
thread = threading.Thread(target=task)
thread.start()
主线程继续执行其他任务
for i in range(10):
print(f"主线程任务 {i}")
time.sleep(1)
4.2 线程同步
在多线程环境中,可能需要进行线程同步。可以使用threading模块中的Lock对象来实现线程同步。
import threading
import time
lock = threading.Lock()
def task():
while True:
with lock:
print("定时任务执行中...")
time.sleep(5)
thread = threading.Thread(target=task)
thread.start()
主线程继续执行其他任务
for i in range(10):
with lock:
print(f"主线程任务 {i}")
time.sleep(1)
五、综合应用
在实际应用中,可以结合使用上述方法,根据具体需求选择合适的时间设置方法。以下是一个综合应用示例:
5.1 定时发送邮件
假设需要每隔一小时发送一封邮件,可以使用schedule模块和threading模块结合实现。
import schedule
import time
import threading
import smtplib
from email.mime.text import MIMEText
def send_email():
msg = MIMEText("这是定时邮件内容", "plain", "utf-8")
msg["From"] = "your_email@example.com"
msg["To"] = "recipient@example.com"
msg["Subject"] = "定时邮件"
try:
server = smtplib.SMTP("smtp.example.com", 587)
server.starttls()
server.login("your_email@example.com", "your_password")
server.sendmail("your_email@example.com", ["recipient@example.com"], msg.as_string())
server.quit()
print("邮件发送成功")
except Exception as e:
print(f"邮件发送失败: {e}")
def job():
print("定时任务执行中...")
send_email()
schedule.every().hour.do(job)
def run_scheduler():
while True:
schedule.run_pending()
time.sleep(1)
thread = threading.Thread(target=run_scheduler)
thread.start()
主线程继续执行其他任务
for i in range(10):
print(f"主线程任务 {i}")
time.sleep(1)
在这个示例中,使用schedule模块设置了一个每小时执行一次的定时任务,并在任务中调用send_email()函数发送邮件。同时,使用threading模块创建了一个新线程来运行定时任务调度器,主线程则继续执行其他任务。
总结起来,使用Python设置使用时间的方法有很多,可以根据具体需求选择合适的方法。使用time模块是最基础的方法,适用于简单的时间操作;使用datetime模块提供了更高级的日期和时间处理功能;使用schedule模块可以方便地设置定时任务;结合线程处理可以实现多任务并发执行。在实际应用中,可以灵活组合这些方法,以满足不同的需求。
相关问答FAQs:
如何在Python中设置程序的运行时间?
在Python中,您可以使用time
模块来控制程序的运行时间。通过time.sleep(seconds)
函数,可以使程序暂停指定的秒数。此外,您还可以使用datetime
模块获取当前时间,并根据需要进行计算,以实现更复杂的时间控制。
Python如何定时执行某个任务?
可以使用schedule
库来定时执行任务。首先,您需要安装该库(使用pip install schedule
),然后通过定义任务函数和设置时间间隔来实现定时任务。例如,可以通过schedule.every().day.at("10:30").do(job)
来每天在10:30执行某个函数。
如何在Python中处理时间格式化?
Python的datetime
模块提供了强大的时间格式化功能。通过strftime
方法,您可以将日期时间对象格式化为字符串,例如:now.strftime("%Y-%m-%d %H:%M:%S")
,这将输出当前的日期和时间。了解不同的格式化代码,可以帮助您根据需求生成所需的时间字符串。
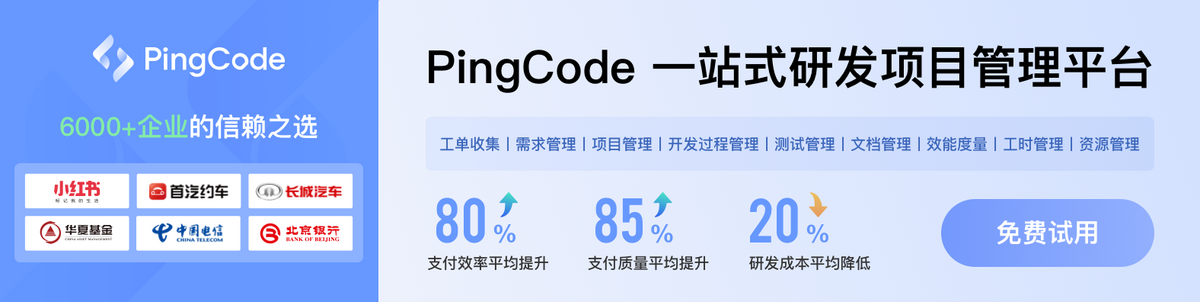