在Python中,列表和元组是两种常见的数据结构,它们都可以存储一系列的元素。要选取列表或元组中的某个特定元素,可以使用索引、切片、条件筛选等方法。 索引和切片是最直接的方法,通过指定位置或范围来选取元素。条件筛选则可以通过循环或列表解析来实现。这篇文章将详细介绍如何使用这些方法来选取列表和元组中的特定元素,并提供一些使用技巧和注意事项。
一、列表和元组的基础知识
在深入讨论如何选取列表和元组中的特定元素之前,首先需要了解它们的基础知识。列表和元组都是序列类型的数据结构,它们的主要区别在于列表是可变的,而元组是不可变的。
列表
列表使用方括号[]
表示,可以包含任意类型的元素,并且可以通过索引、切片等方法进行修改。
my_list = [1, 2, 3, 4, 5]
元组
元组使用小括号()
表示,和列表类似,但元组中的元素一旦定义就不能修改。
my_tuple = (1, 2, 3, 4, 5)
二、使用索引选取特定元素
索引是最直接的方法,通过指定元素在列表或元组中的位置,可以快速选取特定的元素。索引从0开始,负数索引表示从末尾开始计数。
列表中的索引选取
my_list = [10, 20, 30, 40, 50]
print(my_list[2]) # 输出:30
print(my_list[-1]) # 输出:50
元组中的索引选取
my_tuple = (10, 20, 30, 40, 50)
print(my_tuple[2]) # 输出:30
print(my_tuple[-1]) # 输出:50
三、使用切片选取范围内的元素
切片可以选取列表或元组中的一部分元素,通过指定起始和结束位置,可以选取一个子序列。切片的语法为[start:end:step]
,其中start
是起始索引,end
是结束索引(不包括),step
是步长。
列表中的切片选取
my_list = [10, 20, 30, 40, 50]
print(my_list[1:4]) # 输出:[20, 30, 40]
print(my_list[:3]) # 输出:[10, 20, 30]
print(my_list[::2]) # 输出:[10, 30, 50]
元组中的切片选取
my_tuple = (10, 20, 30, 40, 50)
print(my_tuple[1:4]) # 输出:(20, 30, 40)
print(my_tuple[:3]) # 输出:(10, 20, 30)
print(my_tuple[::2]) # 输出:(10, 30, 50)
四、条件筛选选取特定元素
通过条件筛选,可以选取满足特定条件的元素。条件筛选通常使用循环或列表解析来实现。
列表中的条件筛选
使用循环筛选:
my_list = [10, 20, 30, 40, 50]
filtered_list = []
for item in my_list:
if item > 25:
filtered_list.append(item)
print(filtered_list) # 输出:[30, 40, 50]
使用列表解析筛选:
my_list = [10, 20, 30, 40, 50]
filtered_list = [item for item in my_list if item > 25]
print(filtered_list) # 输出:[30, 40, 50]
元组中的条件筛选
由于元组是不可变的,筛选结果通常会存储在一个新的列表或元组中。
使用循环筛选:
my_tuple = (10, 20, 30, 40, 50)
filtered_list = []
for item in my_tuple:
if item > 25:
filtered_list.append(item)
print(filtered_list) # 输出:[30, 40, 50]
使用列表解析筛选:
my_tuple = (10, 20, 30, 40, 50)
filtered_list = [item for item in my_tuple if item > 25]
print(filtered_list) # 输出:[30, 40, 50]
五、使用内置函数选取特定元素
Python 提供了一些内置函数,可以方便地选取列表和元组中的特定元素。
使用min()
和max()
选取最小和最大元素
my_list = [10, 20, 30, 40, 50]
print(min(my_list)) # 输出:10
print(max(my_list)) # 输出:50
my_tuple = (10, 20, 30, 40, 50)
print(min(my_tuple)) # 输出:10
print(max(my_tuple)) # 输出:50
使用index()
查找特定元素的位置
my_list = [10, 20, 30, 40, 50]
print(my_list.index(30)) # 输出:2
my_tuple = (10, 20, 30, 40, 50)
print(my_tuple.index(30)) # 输出:2
使用count()
统计特定元素的出现次数
my_list = [10, 20, 30, 40, 50, 30]
print(my_list.count(30)) # 输出:2
my_tuple = (10, 20, 30, 40, 50, 30)
print(my_tuple.count(30)) # 输出:2
六、使用filter()
函数进行筛选
filter()
函数可以用于筛选满足特定条件的元素。它接受一个函数和一个可迭代对象作为参数,返回一个迭代器,其中包含所有满足条件的元素。
列表中的filter()
筛选
my_list = [10, 20, 30, 40, 50]
filtered_list = list(filter(lambda x: x > 25, my_list))
print(filtered_list) # 输出:[30, 40, 50]
元组中的filter()
筛选
my_tuple = (10, 20, 30, 40, 50)
filtered_tuple = tuple(filter(lambda x: x > 25, my_tuple))
print(filtered_tuple) # 输出:(30, 40, 50)
七、使用map()
函数进行元素转换
map()
函数可以用于对列表或元组中的每个元素应用一个函数,并返回一个迭代器,其中包含所有转换后的元素。
列表中的map()
转换
my_list = [10, 20, 30, 40, 50]
mapped_list = list(map(lambda x: x * 2, my_list))
print(mapped_list) # 输出:[20, 40, 60, 80, 100]
元组中的map()
转换
my_tuple = (10, 20, 30, 40, 50)
mapped_tuple = tuple(map(lambda x: x * 2, my_tuple))
print(mapped_tuple) # 输出:(20, 40, 60, 80, 100)
八、使用reduce()
函数进行元素聚合
reduce()
函数可以用于对列表或元组中的元素进行聚合操作。它接受一个函数和一个可迭代对象作为参数,并返回一个单一的聚合值。
列表中的reduce()
聚合
from functools import reduce
my_list = [10, 20, 30, 40, 50]
sum_value = reduce(lambda x, y: x + y, my_list)
print(sum_value) # 输出:150
元组中的reduce()
聚合
from functools import reduce
my_tuple = (10, 20, 30, 40, 50)
sum_value = reduce(lambda x, y: x + y, my_tuple)
print(sum_value) # 输出:150
九、使用zip()
函数进行元素配对
zip()
函数可以用于将多个列表或元组中的元素配对,并返回一个迭代器,其中包含所有配对后的元素。
列表中的zip()
配对
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
zipped_list = list(zip(list1, list2))
print(zipped_list) # 输出:[(1, 'a'), (2, 'b'), (3, 'c')]
元组中的zip()
配对
tuple1 = (1, 2, 3)
tuple2 = ('a', 'b', 'c')
zipped_tuple = tuple(zip(tuple1, tuple2))
print(zipped_tuple) # 输出:((1, 'a'), (2, 'b'), (3, 'c'))
十、使用列表和元组的组合方法
在实际应用中,列表和元组常常需要组合使用。通过灵活运用各种方法,可以实现更复杂的数据处理任务。
列表和元组的组合操作
my_list = [1, 2, 3]
my_tuple = (4, 5, 6)
combined_list = my_list + list(my_tuple)
print(combined_list) # 输出:[1, 2, 3, 4, 5, 6]
列表和元组的嵌套操作
nested_list = [1, 2, (3, 4), [5, 6]]
print(nested_list[2][1]) # 输出:4
print(nested_list[3][0]) # 输出:5
总结
选取Python列表和元组中的特定元素可以通过多种方法实现,包括索引、切片、条件筛选、内置函数、filter()
、map()
、reduce()
、zip()
等。每种方法都有其适用的场景和优势。通过掌握这些方法,可以更高效地处理和操作列表和元组中的数据。无论是简单的索引选取,还是复杂的条件筛选和聚合操作,都可以在实际编程中得到广泛应用。希望本篇文章能帮助读者深入理解和灵活运用Python列表和元组的选取技巧。
相关问答FAQs:
如何在Python中从列表中选择特定元素?
在Python中,可以通过索引来选择列表中的特定元素。索引从0开始,因此第一个元素的索引为0,第二个元素的索引为1,以此类推。如果想要选择多个元素,可以使用切片,例如my_list[1:4]
将返回从索引1到索引3的元素。如果需要选择特定条件的元素,可以使用列表推导式,例如[x for x in my_list if x > 10]
。
如何从元组中提取特定值?
元组的操作与列表类似,使用索引可以方便地获取元组中的特定元素。例如,使用my_tuple[2]
将获取元组中第三个元素。由于元组是不可变的,如果需要提取多个元素,可以使用切片,如my_tuple[1:3]
。然而,要修改元组的内容,必须创建一个新的元组。
在Python中如何有效地遍历列表和元组?
遍历列表和元组可以使用for
循环。例如,for item in my_list:
会遍历列表中的每个元素。对于元组也相同,使用for item in my_tuple:
进行遍历。若需要同时获取索引,可以使用enumerate
函数,如for index, item in enumerate(my_list):
,这样不仅可以获取元素,还可以同时获取其索引。
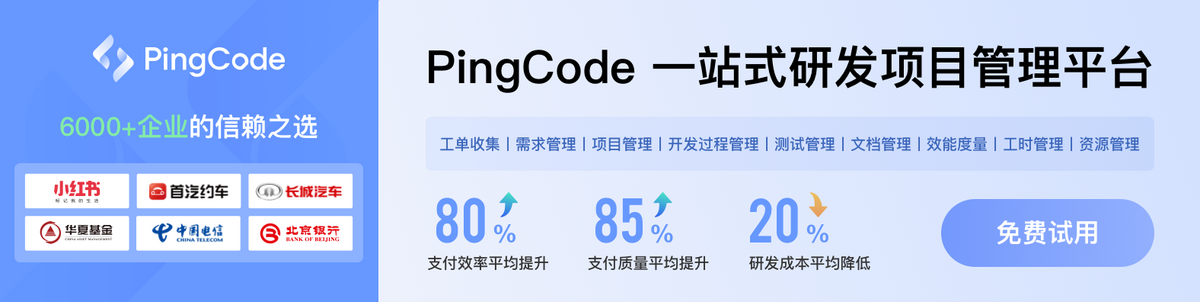