Python线程执行完了如何再次执行,使用线程池、循环创建线程、检查线程状态
在Python中,可以通过多种方式实现线程的重复执行。使用线程池、循环创建线程、检查线程状态是其中几种有效的方法。下面将详细介绍这几种方法,并提供相关的代码示例。
一、使用线程池
线程池是一种线程管理机制,可以通过线程池来管理和重复执行线程任务。Python的concurrent.futures
模块提供了一个方便的线程池实现,即ThreadPoolExecutor
。
示例代码:
import concurrent.futures
import time
def worker():
print("Thread is running")
time.sleep(2)
print("Thread is done")
if __name__ == "__main__":
with concurrent.futures.ThreadPoolExecutor(max_workers=2) as executor:
while True:
future = executor.submit(worker)
time.sleep(5) # Wait for 5 seconds before re-executing the thread
在这个示例中,ThreadPoolExecutor
被用来创建一个线程池,通过submit
方法提交worker
函数来执行任务。通过循环和延时,可以实现线程的重复执行。
二、循环创建线程
另一种方法是通过循环创建线程来实现线程的重复执行。这种方法适合于简单的线程管理需求。
示例代码:
import threading
import time
def worker():
print("Thread is running")
time.sleep(2)
print("Thread is done")
if __name__ == "__main__":
while True:
thread = threading.Thread(target=worker)
thread.start()
thread.join() # Wait for the thread to complete before creating a new one
time.sleep(5) # Wait for 5 seconds before creating a new thread
在这个示例中,通过threading.Thread
创建新的线程,并使用join
方法等待线程完成。然后通过延时实现线程的重复执行。
三、检查线程状态
通过检查线程的状态,可以在线程执行完毕后再次执行线程。可以使用threading.Event
来实现线程间的同步和状态检查。
示例代码:
import threading
import time
def worker(event):
while True:
event.wait() # Wait until the event is set
print("Thread is running")
time.sleep(2)
print("Thread is done")
event.clear() # Clear the event to indicate the thread is done
if __name__ == "__main__":
event = threading.Event()
thread = threading.Thread(target=worker, args=(event,))
thread.start()
while True:
time.sleep(5) # Wait for 5 seconds before setting the event
event.set() # Set the event to trigger the thread
在这个示例中,通过threading.Event
实现线程间的同步。主线程通过设置和清除事件来控制工作线程的执行。
四、使用定时器
定时器是一种用于在特定时间间隔后执行某个函数的机制。Python的threading.Timer
类提供了一个简单的定时器实现。
示例代码:
import threading
import time
def worker():
print("Thread is running")
time.sleep(2)
print("Thread is done")
timer = threading.Timer(5, worker)
timer.start()
if __name__ == "__main__":
timer = threading.Timer(5, worker)
timer.start()
在这个示例中,通过threading.Timer
创建一个定时器,在指定的时间间隔后执行worker
函数。在worker
函数内部再次创建定时器实现重复执行。
五、使用多进程
除了线程之外,Python还支持多进程编程。通过多进程可以实现类似于线程的并发执行,并且多进程在某些情况下比线程具有更高的性能和稳定性。
示例代码:
import multiprocessing
import time
def worker():
print("Process is running")
time.sleep(2)
print("Process is done")
if __name__ == "__main__":
while True:
process = multiprocessing.Process(target=worker)
process.start()
process.join() # Wait for the process to complete before creating a new one
time.sleep(5) # Wait for 5 seconds before creating a new process
在这个示例中,通过multiprocessing.Process
创建新的进程,并使用join
方法等待进程完成。然后通过延时实现进程的重复执行。
六、使用队列
使用队列可以实现线程任务的调度和管理。通过队列可以将任务添加到队列中,然后由线程从队列中获取任务进行执行。
示例代码:
import threading
import queue
import time
def worker(q):
while True:
task = q.get()
if task is None:
break
print("Thread is running")
time.sleep(2)
print("Thread is done")
q.task_done()
if __name__ == "__main__":
q = queue.Queue()
thread = threading.Thread(target=worker, args=(q,))
thread.start()
while True:
q.put("task")
time.sleep(5) # Wait for 5 seconds before adding a new task to the queue
在这个示例中,通过queue.Queue
创建任务队列,将任务添加到队列中,然后由工作线程从队列中获取任务进行执行。
七、使用协程
协程是一种轻量级的并发实现方式,适用于IO密集型任务。Python的asyncio
模块提供了协程的支持。
示例代码:
import asyncio
async def worker():
while True:
print("Coroutine is running")
await asyncio.sleep(2)
print("Coroutine is done")
await asyncio.sleep(5) # Wait for 5 seconds before re-executing the coroutine
if __name__ == "__main__":
asyncio.run(worker())
在这个示例中,通过asyncio
模块创建和管理协程,实现类似于线程的并发执行。
总结
在Python中,可以通过多种方式实现线程的重复执行,包括使用线程池、循环创建线程、检查线程状态、使用定时器、使用多进程、使用队列、使用协程等。每种方法都有其适用的场景和优缺点。选择合适的方法可以提高代码的性能和稳定性。
相关问答FAQs:
如何在Python中重启已完成的线程?
在Python中,线程一旦完成其任务后便不能被直接重启。要再次执行相同的任务,您需要创建一个新的线程实例。可以通过定义一个函数来封装要执行的代码,并在需要时使用threading.Thread
类实例化新的线程。
如何处理Python线程中的异常?
在Python线程中,如果出现异常,线程会终止并且不会返回到主线程。为了确保线程的稳定性,建议在目标函数内使用try-except
块来捕获并处理任何可能出现的异常。这种做法能够有效防止线程因未处理的异常而意外结束。
在Python中如何管理多个线程的执行?
管理多个线程通常可以使用threading
模块中的Thread
类,结合join()
方法来确保主程序等待所有线程执行完成。此外,使用threading.Lock
可以避免多个线程之间的资源竞争,确保线程安全。通过设计合理的线程调度和资源管理策略,可以有效提高程序的性能和稳定性。
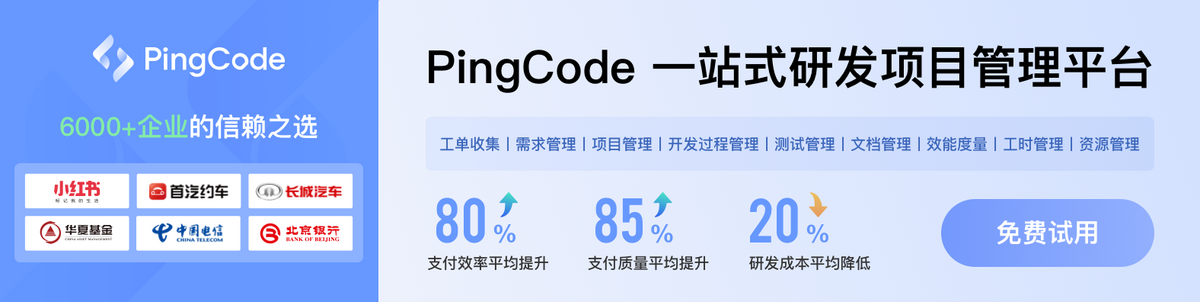