如何与Python一起使用
使用Python可以通过其广泛的库和工具实现自动化、数据分析、机器学习、Web开发等多种功能、Python的简单易学性使其成为初学者和专业开发者的首选语言、Python的社区支持和丰富的文档资源为用户提供了强大的支持。其中,Python的广泛库支持是其最大的优势之一,接下来我们将详细介绍这一点。
Python拥有一个庞大的标准库,这使得开发者可以更快速地实现各种功能。例如,numpy
和pandas
库在数据分析和科学计算中非常常用;scikit-learn
和tensorflow
库在机器学习和深度学习领域应用广泛;flask
和django
库为Web开发提供了强大的支持。通过这些库,Python可以处理从简单的脚本到复杂的应用程序开发。
一、安装和配置Python环境
1. 安装Python解释器
在使用Python之前,首先需要安装Python解释器。可以从Python官方网站(https://www.python.org/)下载最新版本的Python安装包。安装过程中,确保选中“Add Python to PATH”选项,这样可以在命令行中直接使用Python命令。
2. 配置虚拟环境
虚拟环境是Python中非常重要的概念,它允许在不同项目中使用不同的依赖包版本,避免库版本冲突。可以使用venv
模块创建虚拟环境:
python -m venv myenv
然后激活虚拟环境:
- Windows:
myenv\Scripts\activate
- macOS和Linux:
source myenv/bin/activate
在虚拟环境中,可以使用pip
命令安装需要的库:
pip install numpy pandas
二、数据分析与科学计算
1. 使用NumPy进行数值计算
NumPy是Python中进行数值计算的基础库,它提供了高效的多维数组对象和丰富的数学函数。可以使用NumPy进行数组操作、线性代数计算、随机数生成等。
import numpy as np
创建一个一维数组
arr = np.array([1, 2, 3, 4, 5])
print(arr)
创建一个二维数组
arr2 = np.array([[1, 2, 3], [4, 5, 6]])
print(arr2)
数组操作
print(arr + 10)
print(arr2.T) # 转置
2. 使用Pandas进行数据处理
Pandas是Python中进行数据处理和分析的强大工具,提供了DataFrame数据结构,可以方便地进行数据清洗、数据转换、数据汇总等操作。
import pandas as pd
创建一个DataFrame
data = {
'name': ['Alice', 'Bob', 'Charlie'],
'age': [25, 30, 35],
'city': ['New York', 'San Francisco', 'Los Angeles']
}
df = pd.DataFrame(data)
print(df)
数据选择
print(df['name'])
print(df[['name', 'age']])
数据过滤
print(df[df['age'] > 30])
数据汇总
print(df['age'].mean())
三、机器学习与深度学习
1. 使用Scikit-learn进行机器学习
Scikit-learn是Python中最常用的机器学习库,提供了各种机器学习算法和工具,可以方便地进行数据预处理、模型训练、模型评估等。
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
生成随机数据
X = np.random.rand(100, 1)
y = 3 * X.squeeze() + 2 + np.random.randn(100)
数据划分
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
模型训练
model = LinearRegression()
model.fit(X_train, y_train)
模型预测
y_pred = model.predict(X_test)
模型评估
print(f'Mean Squared Error: {mean_squared_error(y_test, y_pred)}')
2. 使用TensorFlow进行深度学习
TensorFlow是一个开源的深度学习框架,可以用于构建和训练复杂的深度神经网络。TensorFlow提供了高效的计算图和自动微分功能,支持GPU加速。
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
生成随机数据
X = np.random.rand(100, 1)
y = 3 * X.squeeze() + 2 + np.random.randn(100)
构建模型
model = Sequential([
Dense(1, input_shape=(1,), activation='linear')
])
编译模型
model.compile(optimizer='adam', loss='mse')
训练模型
model.fit(X, y, epochs=100, verbose=0)
模型预测
y_pred = model.predict(X)
print(y_pred)
四、Web开发
1. 使用Flask开发Web应用
Flask是一个轻量级的Web框架,适用于构建小型Web应用和API。Flask提供了简洁的路由和模板功能,可以快速开发和部署Web应用。
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/')
def home():
return "Hello, Flask!"
@app.route('/api', methods=['POST'])
def api():
data = request.json
return jsonify({"message": f"Hello, {data['name']}!"})
if __name__ == '__main__':
app.run(debug=True)
2. 使用Django开发Web应用
Django是一个功能强大的Web框架,适用于构建复杂的Web应用。Django提供了丰富的功能,包括ORM、表单、认证等,可以快速构建和部署企业级Web应用。
django-admin startproject mysite
cd mysite
python manage.py startapp myapp
在myapp/views.py
中定义视图:
from django.http import HttpResponse
def home(request):
return HttpResponse("Hello, Django!")
在mysite/urls.py
中配置路由:
from django.contrib import admin
from django.urls import path
from myapp import views
urlpatterns = [
path('admin/', admin.site.urls),
path('', views.home),
]
使用命令启动开发服务器:
python manage.py runserver
五、自动化脚本
1. 使用Python进行文件操作
Python提供了丰富的文件操作功能,可以方便地读取、写入、删除文件以及操作目录结构。
import os
读取文件
with open('example.txt', 'r') as file:
content = file.read()
print(content)
写入文件
with open('output.txt', 'w') as file:
file.write("Hello, World!")
删除文件
os.remove('output.txt')
创建目录
os.makedirs('example_dir')
删除目录
os.rmdir('example_dir')
2. 使用Python进行网络请求
Python的requests
库是进行HTTP请求的强大工具,可以方便地进行GET、POST等操作,适用于网页抓取、API调用等场景。
import requests
发送GET请求
response = requests.get('https://api.github.com')
print(response.status_code)
print(response.json())
发送POST请求
data = {'name': 'John', 'age': 30}
response = requests.post('https://httpbin.org/post', json=data)
print(response.status_code)
print(response.json())
六、数据可视化
1. 使用Matplotlib进行数据可视化
Matplotlib是Python中最常用的数据可视化库,可以创建各种图表,如折线图、柱状图、散点图等。
import matplotlib.pyplot as plt
创建数据
x = np.linspace(0, 10, 100)
y = np.sin(x)
绘制折线图
plt.plot(x, y)
plt.xlabel('X axis')
plt.ylabel('Y axis')
plt.title('Sine Wave')
plt.show()
2. 使用Seaborn进行高级数据可视化
Seaborn是基于Matplotlib的高级数据可视化库,提供了更美观的图表样式和更强大的数据可视化功能。
import seaborn as sns
创建数据
data = sns.load_dataset('iris')
绘制散点图
sns.scatterplot(x='sepal_length', y='sepal_width', hue='species', data=data)
plt.xlabel('Sepal Length')
plt.ylabel('Sepal Width')
plt.title('Iris Sepal Dimensions')
plt.show()
七、异常处理和调试
1. 异常处理
Python提供了强大的异常处理机制,可以通过try...except
语句捕获和处理运行时错误,确保程序的健壮性。
try:
result = 10 / 0
except ZeroDivisionError as e:
print(f"Error: {e}")
finally:
print("This block always executes")
2. 调试
Python提供了多种调试工具,如pdb
、logging
模块,可以方便地调试和记录程序运行过程中的信息。
import logging
logging.basicConfig(level=logging.DEBUG)
def divide(a, b):
logging.debug(f"Dividing {a} by {b}")
return a / b
try:
result = divide(10, 0)
except ZeroDivisionError as e:
logging.error(f"Error: {e}")
八、面向对象编程
1. 类和对象
Python支持面向对象编程,可以通过定义类和创建对象来封装数据和功能。
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
创建对象
person = Person("Alice", 30)
person.greet()
2. 继承和多态
Python支持类的继承,可以通过继承父类来创建子类,并重写父类的方法实现多态。
class Animal:
def speak(self):
pass
class Dog(Animal):
def speak(self):
print("Woof!")
class Cat(Animal):
def speak(self):
print("Meow!")
创建对象
animals = [Dog(), Cat()]
for animal in animals:
animal.speak()
九、单元测试
1. 使用unittest进行单元测试
Python的unittest
模块提供了丰富的单元测试功能,可以方便地编写和运行测试用例,确保代码的正确性。
import unittest
def add(a, b):
return a + b
class TestMathFunctions(unittest.TestCase):
def test_add(self):
self.assertEqual(add(1, 2), 3)
self.assertEqual(add(-1, 1), 0)
if __name__ == '__main__':
unittest.main()
2. 使用pytest进行单元测试
pytest
是一个功能强大的第三方测试框架,提供了更简洁的语法和更丰富的功能,适用于编写和运行复杂的测试用例。
import pytest
def add(a, b):
return a + b
def test_add():
assert add(1, 2) == 3
assert add(-1, 1) == 0
if __name__ == '__main__':
pytest.main()
十、并发编程
1. 使用多线程
Python的threading
模块提供了多线程编程的功能,可以通过创建线程来实现并发执行,提高程序的执行效率。
import threading
import time
def worker():
print("Worker thread is running")
time.sleep(2)
print("Worker thread is done")
创建和启动线程
thread = threading.Thread(target=worker)
thread.start()
等待线程结束
thread.join()
print("Main thread is done")
2. 使用多进程
Python的multiprocessing
模块提供了多进程编程的功能,可以通过创建进程来实现并发执行,适用于CPU密集型任务。
import multiprocessing
import time
def worker():
print("Worker process is running")
time.sleep(2)
print("Worker process is done")
创建和启动进程
process = multiprocessing.Process(target=worker)
process.start()
等待进程结束
process.join()
print("Main process is done")
结论
通过本文的介绍,我们详细探讨了如何与Python一起使用。安装和配置Python环境、数据分析与科学计算、机器学习与深度学习、Web开发、自动化脚本、数据可视化、异常处理和调试、面向对象编程、单元测试、并发编程等方面的内容。Python作为一门功能强大且易于学习的编程语言,提供了丰富的库和工具,能够满足各种开发需求。希望通过本文的介绍,能够帮助读者更好地理解和使用Python进行开发工作。
相关问答FAQs:
如何在Python中调用R脚本?
可以通过使用rpy2
库将R与Python结合使用。安装rpy2
后,可以在Python代码中导入R的功能,调用R脚本并处理数据。例如,您可以使用rpy2.robjects
来执行R代码,或者将数据框从Python转换为R的数据帧。
R与Python的结合是否适合数据科学项目?
绝对适合。R在统计分析和图形可视化方面拥有丰富的库,而Python在数据处理和机器学习方面表现出色。通过将两者结合,您可以利用各自的优势,从而增强数据科学项目的效率和效果。
如何在Jupyter Notebook中使用R和Python?
在Jupyter Notebook中,可以通过安装IRkernel
来支持R语言,同时保持Python内核。用户可以在同一个Notebook中创建不同的单元格,分别编写R和Python代码,实现无缝的数据处理和分析。此外,rpy2
库也可以在Python单元中直接调用R代码,使得跨语言操作变得更加灵活。
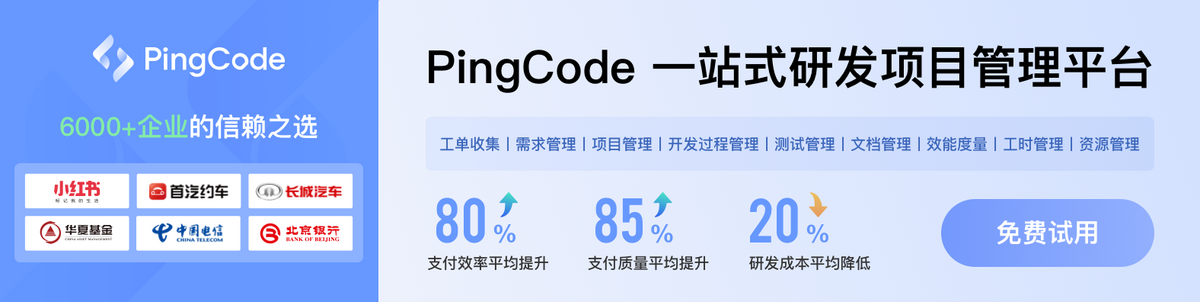