Python转换为字符串类型数据的方法有:使用str()函数、使用repr()函数、格式化字符串、使用f-string。 其中最常用的是str()函数,它能够将多种数据类型转换为字符串类型。str()函数不仅可以处理基本的数据类型如整数、浮点数等,还能处理复杂的数据类型如列表、元组、字典等。下面我们将详细介绍这些方法,并提供相应的示例代码。
一、使用str()函数
str()函数是Python中最常用的将其他类型转换为字符串类型的方法。它可以将整数、浮点数、列表、元组、字典等类型转换为字符串。
# 示例代码
int_value = 123
float_value = 45.67
list_value = [1, 2, 3]
tuple_value = (4, 5, 6)
dict_value = {'a': 1, 'b': 2}
使用str()函数转换
str_int = str(int_value)
str_float = str(float_value)
str_list = str(list_value)
str_tuple = str(tuple_value)
str_dict = str(dict_value)
print(str_int) # 输出: '123'
print(str_float) # 输出: '45.67'
print(str_list) # 输出: '[1, 2, 3]'
print(str_tuple) # 输出: '(4, 5, 6)'
print(str_dict) # 输出: '{'a': 1, 'b': 2}'
二、使用repr()函数
repr()函数返回一个对象的“官方”字符串表示,可以用来生成解释器易读的字符串。与str()不同的是,repr()函数的输出通常更适合调试和开发。
# 示例代码
int_value = 123
float_value = 45.67
list_value = [1, 2, 3]
tuple_value = (4, 5, 6)
dict_value = {'a': 1, 'b': 2}
使用repr()函数转换
repr_int = repr(int_value)
repr_float = repr(float_value)
repr_list = repr(list_value)
repr_tuple = repr(tuple_value)
repr_dict = repr(dict_value)
print(repr_int) # 输出: '123'
print(repr_float) # 输出: '45.67'
print(repr_list) # 输出: '[1, 2, 3]'
print(repr_tuple) # 输出: '(4, 5, 6)'
print(repr_dict) # 输出: '{'a': 1, 'b': 2}'
三、使用格式化字符串
Python支持多种字符串格式化方法,包括百分号(%)格式化、str.format()方法和f-string格式化。格式化字符串不仅可以将数据转换为字符串,还可以控制其格式。
1. 百分号(%)格式化
# 示例代码
name = "Alice"
age = 30
formatted_str = "Name: %s, Age: %d" % (name, age)
print(formatted_str) # 输出: 'Name: Alice, Age: 30'
2. str.format()方法
# 示例代码
name = "Alice"
age = 30
formatted_str = "Name: {}, Age: {}".format(name, age)
print(formatted_str) # 输出: 'Name: Alice, Age: 30'
3. f-string格式化(Python 3.6+)
# 示例代码
name = "Alice"
age = 30
formatted_str = f"Name: {name}, Age: {age}"
print(formatted_str) # 输出: 'Name: Alice, Age: 30'
四、结合上述方法的实用技巧
1. 转换复杂嵌套数据结构
当处理复杂的嵌套数据结构时,可以结合使用str()和repr()函数来获得更直观的输出。例如:
# 示例代码
nested_data = {
'name': 'Alice',
'age': 30,
'scores': [85, 90, 78],
'details': {
'city': 'New York',
'occupation': 'Engineer'
}
}
str_nested = str(nested_data)
repr_nested = repr(nested_data)
print(str_nested) # 输出: {'name': 'Alice', 'age': 30, 'scores': [85, 90, 78], 'details': {'city': 'New York', 'occupation': 'Engineer'}}
print(repr_nested) # 输出: {'name': 'Alice', 'age': 30, 'scores': [85, 90, 78], 'details': {'city': 'New York', 'occupation': 'Engineer'}}
2. 将数值转换为特定格式字符串
有时候需要将数值转换为具有特定格式的字符串,例如保留小数点后两位或以科学计数法表示。这时可以使用格式化字符串的技巧。
# 示例代码
pi = 3.141592653589793
保留小数点后两位
formatted_pi = "{:.2f}".format(pi)
print(formatted_pi) # 输出: '3.14'
以科学计数法表示
formatted_sci = "{:.2e}".format(pi)
print(formatted_sci) # 输出: '3.14e+00'
3. 处理特殊字符
在处理包含特殊字符的数据时,使用repr()函数可以更清楚地看到字符串的原始表示。例如:
# 示例代码
special_str = "Hello\nWorld\t!"
str_special = str(special_str)
repr_special = repr(special_str)
print(str_special) # 输出: 'Hello
# World !'
print(repr_special) # 输出: 'Hello\nWorld\t!'
五、总结
在Python中,将其他类型的数据转换为字符串类型是一个常见的需求。本文介绍了使用str()函数、repr()函数、格式化字符串(包括百分号格式化、str.format()方法和f-string格式化)来实现这一转换的多种方法。通过这些方法,你可以轻松地将整数、浮点数、列表、元组、字典等类型的数据转换为字符串,并根据需要调整其格式。希望这些方法和技巧能对你在Python编程中的字符串处理有所帮助。
相关问答FAQs:
如何在Python中将整数转换为字符串?
在Python中,可以使用str()
函数将整数转换为字符串。例如,num = 10
,使用str(num)
即可将num
转换为字符串类型,结果为'10'
。
Python中有哪几种方法可以将列表转换为字符串?
可以通过多种方式将列表转换为字符串。其中一种常用的方法是使用join()
函数。例如,如果有一个列表my_list = ['Python', 'is', 'great']
,可以使用' '.join(my_list)
将其转换为字符串,结果为'Python is great'
。此外,还可以使用列表推导式结合str()
函数进行转换。
在Python中如何处理字符串和其他数据类型的转换?
Python提供了多种数据类型之间的转换方法。对于字符串和浮点数之间的转换,使用float()
函数可以将字符串转换为浮点数,例如float('3.14')
。反之,使用str()
函数可以将浮点数转换为字符串,如str(3.14)
。通过这些内置函数,用户可以方便地处理不同类型的数据。
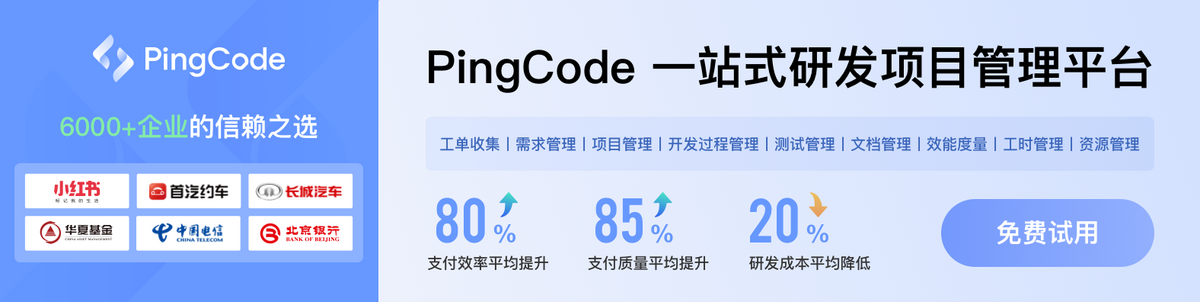