Python3查找字符串的方法有多种,包括使用find()方法、index()方法、正则表达式等。每种方法都有其独特的优点和适用场景。其中,find()方法是最常用的一种,因为它简单易用且效率较高。使用find()方法时,如果找到指定的子字符串,它会返回子字符串的起始位置索引;如果没有找到,则返回-1。具体使用方法如下:
text = "Hello, welcome to the world of Python"
position = text.find("world")
print(position) # 输出:21
另外,index()方法与find()方法类似,但如果未找到子字符串,它会抛出一个ValueError异常。正则表达式则适用于更复杂的字符串查找需求,能够进行模式匹配。以下是对find()方法的详细描述。
一、find()方法
find()方法是Python中查找字符串最常见的方式之一。它用于在字符串中查找指定的子字符串,如果找到则返回子字符串的起始位置索引,否则返回-1。
text = "Hello, welcome to the world of Python"
position = text.find("world")
print(position) # 输出:21
find()方法还可以接受两个可选参数,start和end,用于指定查找的起始和结束位置。
text = "Hello, welcome to the world of Python"
position = text.find("world", 10, 30)
print(position) # 输出:21
二、index()方法
index()方法与find()方法类似,但如果子字符串未找到,它会抛出一个ValueError异常。这在需要确保子字符串存在时非常有用。
text = "Hello, welcome to the world of Python"
try:
position = text.index("world")
print(position) # 输出:21
except ValueError:
print("子字符串未找到")
三、正则表达式
正则表达式提供了更强大的字符串查找功能,能够进行复杂的模式匹配。Python的re模块提供了丰富的正则表达式支持。
import re
text = "Hello, welcome to the world of Python"
pattern = re.compile(r"world")
match = pattern.search(text)
if match:
print(match.start()) # 输出:21
四、count()方法
count()方法用于统计子字符串在字符串中出现的次数。虽然它不返回子字符串的位置,但在需要了解子字符串出现频率时非常有用。
text = "Hello, welcome to the world of Python. Python is great!"
count = text.count("Python")
print(count) # 输出:2
五、使用in运算符
in运算符可以用于检查子字符串是否存在于字符串中,它返回一个布尔值。虽然它不提供子字符串的位置,但在需要简单存在性检查时非常方便。
text = "Hello, welcome to the world of Python"
exists = "world" in text
print(exists) # 输出:True
六、startswith()和endswith()方法
startswith()和endswith()方法用于检查字符串是否以指定的子字符串开头或结尾。这对于特定模式的匹配非常有用。
text = "Hello, welcome to the world of Python"
starts_with = text.startswith("Hello")
print(starts_with) # 输出:True
ends_with = text.endswith("Python")
print(ends_with) # 输出:True
七、查找多个子字符串
有时我们需要在字符串中查找多个子字符串,使用字典可以方便地记录每个子字符串的起始位置。
text = "Hello, welcome to the world of Python"
substrings = ["Hello", "world", "Python"]
positions = {substr: text.find(substr) for substr in substrings}
print(positions) # 输出:{'Hello': 0, 'world': 21, 'Python': 31}
八、字符串切片与查找结合
通过结合字符串切片和查找方法,可以实现更灵活的字符串操作。例如,可以在找到子字符串后截取特定范围的内容。
text = "Hello, welcome to the world of Python"
position = text.find("world")
if position != -1:
snippet = text[position:position + 5] # 截取"world"
print(snippet) # 输出:world
九、自定义查找函数
在某些情况下,内置方法可能无法完全满足需求,可以编写自定义函数来实现更复杂的查找逻辑。例如,查找所有出现位置。
def find_all_occurrences(text, substring):
start = 0
positions = []
while start < len(text):
position = text.find(substring, start)
if position == -1:
break
positions.append(position)
start = position + 1
return positions
text = "Hello, welcome to the world of Python. Python is great!"
positions = find_all_occurrences(text, "Python")
print(positions) # 输出:[31, 39]
十、性能优化
在处理大文本或频繁查找操作时,性能优化显得尤为重要。可以通过以下几种方式提升性能:
- 缓存结果:对于重复查找的子字符串,可以缓存查找结果,避免重复计算。
- 使用合适的数据结构:在某些场景下,使用更高效的数据结构(如Trie树)可以提升查找效率。
- 并行处理:在多核处理器上,可以利用多线程或多进程并行查找,提高处理速度。
# 示例:使用缓存提升查找性能
cache = {}
def find_with_cache(text, substring):
if substring in cache:
return cache[substring]
position = text.find(substring)
cache[substring] = position
return position
text = "Hello, welcome to the world of Python"
position = find_with_cache(text, "world")
print(position) # 输出:21
通过了解和掌握上述各种查找方法及其应用场景,可以在实际编程中灵活选择合适的工具,提升代码的可读性和运行效率。无论是简单的查找需求还是复杂的模式匹配,Python都提供了丰富的解决方案。
相关问答FAQs:
如何在Python3中查找字符串的特定子串?
在Python3中,可以使用str.find()
或str.index()
方法来查找字符串中的特定子串。find()
方法返回子串的起始索引,如果未找到则返回-1;而index()
方法在未找到时会引发ValueError
异常。此外,str.contains()
方法和正则表达式也可以用于更复杂的查找。
Python3中有哪些方法可以进行字符串的模糊查找?
如果需要进行模糊查找,可以使用正则表达式模块re
。例如,re.search()
可以用来查找符合特定模式的字符串,无论其位置如何。此外,str.lower()
和str.upper()
方法可以帮助实现不区分大小写的查找。
如何在Python3中查找字符串的所有出现位置?
为了找到字符串中所有子串的出现位置,可以使用re.finditer()
函数。这个方法返回一个迭代器,包含所有匹配的对象,您可以通过遍历这些对象来获取每次匹配的起始和结束索引。同时,可以结合使用列表推导式来整理这些索引。
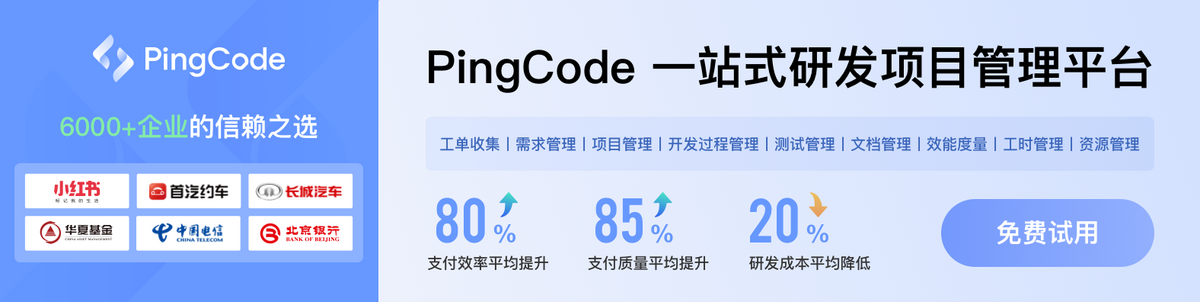