Python如何同时处理字符串和数字
在Python中,可以通过多种方法同时处理字符串和数字,包括类型转换、字符串格式化、正则表达式处理等。接下来,我们将详细探讨这些方法。
类型转换
在Python中,类型转换是一种非常常见且重要的操作。当需要在字符串和数字之间进行转换时,Python提供了非常简便的方法。主要的类型转换函数包括str()
和int()
,这些函数可以在不同的数据类型之间进行转换。
例如,我们可以使用str()
函数将一个数字转换成字符串:
number = 123
string_number = str(number)
print(string_number) # 输出:'123'
相反,我们可以使用int()
函数将一个字符串转换成数字:
string_number = "123"
number = int(string_number)
print(number) # 输出:123
需要注意的是,当字符串无法转换成数字时,会引发ValueError
异常,因此需要特别小心处理。
字符串格式化
字符串格式化是另一种同时处理字符串和数字的有效方法。Python提供了多种字符串格式化方式,例如%
格式化、str.format()
方法和f-strings(格式化字符串字面量)。
- 百分号格式化
name = "John"
age = 30
formatted_string = "My name is %s and I am %d years old." % (name, age)
print(formatted_string) # 输出:My name is John and I am 30 years old.
- str.format()方法
name = "John"
age = 30
formatted_string = "My name is {} and I am {} years old.".format(name, age)
print(formatted_string) # 输出:My name is John and I am 30 years old.
- f-strings(格式化字符串字面量)
name = "John"
age = 30
formatted_string = f"My name is {name} and I am {age} years old."
print(formatted_string) # 输出:My name is John and I am 30 years old.
正则表达式处理
正则表达式是一种强大的工具,用于在字符串中查找和处理特定模式的数据。Python的re
模块提供了对正则表达式的支持,可以很方便地在字符串中查找数字或将数字和字符串混合处理。
例如,提取字符串中的所有数字:
import re
text = "The price is 100 dollars and the discount is 20%"
numbers = re.findall(r'\d+', text)
print(numbers) # 输出:['100', '20']
一、类型转换
类型转换在Python中非常重要,尤其是当需要同时处理字符串和数字时。不同的数据类型之间的转换操作可以帮助我们更灵活地处理数据。
数字到字符串的转换
使用str()
函数可以将数字转换成字符串。这在需要将数字嵌入到字符串中的时候非常有用。例如:
number = 42
string_number = str(number)
print(f"The number is {string_number}") # 输出:The number is 42
字符串到数字的转换
同样,使用int()
或float()
函数可以将字符串转换成整数或浮点数。这在需要对字符串中包含的数字进行数学运算时非常有用。例如:
string_number = "42"
number = int(string_number)
print(number + 8) # 输出:50
string_float = "3.14"
float_number = float(string_float)
print(float_number * 2) # 输出:6.28
需要注意的是,当字符串无法转换成数字时,会引发ValueError
异常。例如,试图将"abc"
转换成数字会失败:
invalid_string = "abc"
try:
invalid_number = int(invalid_string)
except ValueError:
print("Cannot convert to an integer") # 输出:Cannot convert to an integer
二、字符串格式化
字符串格式化在Python中是非常常用的一种方法,用于将变量的值嵌入到字符串中。Python提供了多种字符串格式化方式,适用于不同的场景。
百分号格式化
百分号格式化是Python中最早的一种字符串格式化方法。它使用%
操作符将变量的值插入到字符串的占位符中。例如:
name = "Alice"
age = 25
formatted_string = "My name is %s and I am %d years old." % (name, age)
print(formatted_string) # 输出:My name is Alice and I am 25 years old.
str.format()方法
str.format()
方法是Python 3中引入的字符串格式化方法,它比百分号格式化更灵活、更强大。例如:
name = "Bob"
age = 30
formatted_string = "My name is {} and I am {} years old.".format(name, age)
print(formatted_string) # 输出:My name is Bob and I am 30 years old.
我们还可以使用索引来指定占位符的顺序:
formatted_string = "My name is {1} and I am {0} years old.".format(age, name)
print(formatted_string) # 输出:My name is Bob and I am 30 years old.
f-strings(格式化字符串字面量)
f-strings是Python 3.6中引入的一种新的字符串格式化方法,它提供了一种更简洁、更直观的方式来嵌入变量的值。f-strings使用f
前缀和大括号{}
来包含变量。例如:
name = "Charlie"
age = 35
formatted_string = f"My name is {name} and I am {age} years old."
print(formatted_string) # 输出:My name is Charlie and I am 35 years old.
f-strings还支持表达式的计算:
width = 10
height = 5
formatted_string = f"The area is {width * height} square units."
print(formatted_string) # 输出:The area is 50 square units.
三、正则表达式处理
正则表达式是一种强大的工具,用于在字符串中查找和处理特定模式的数据。Python的re
模块提供了对正则表达式的支持,可以很方便地在字符串中查找数字或将数字和字符串混合处理。
查找字符串中的数字
我们可以使用re.findall()
函数来查找字符串中的所有数字。例如:
import re
text = "The population is 7.8 billion and the area is 9.8 million km^2"
numbers = re.findall(r'\d+\.\d+|\d+', text)
print(numbers) # 输出:['7.8', '9.8']
在这个例子中,正则表达式\d+\.\d+|\d+
用于匹配整数和浮点数。
替换字符串中的数字
我们还可以使用re.sub()
函数来替换字符串中的数字。例如:
import re
text = "The price is 100 dollars and the discount is 20%"
new_text = re.sub(r'\d+', 'XXX', text)
print(new_text) # 输出:The price is XXX dollars and the discount is XXX%
在这个例子中,所有的数字都被替换成了XXX
。
提取并计算字符串中的数字
我们可以从字符串中提取数字,然后进行计算。例如:
import re
text = "The length is 5 meters and the width is 3 meters"
numbers = re.findall(r'\d+', text)
length = int(numbers[0])
width = int(numbers[1])
area = length * width
print(f"The area is {area} square meters.") # 输出:The area is 15 square meters.
四、字符串与数字的混合处理
在实际编程中,处理包含字符串和数字的数据是很常见的需求。通过结合前面介绍的技术,我们可以灵活地处理这类数据。
处理包含数值的字符串
例如,我们可能会遇到包含数值的字符串,需要提取数值并进行计算:
import re
text = "The price of item A is 30 dollars, and the price of item B is 45 dollars."
prices = re.findall(r'\d+', text)
total_price = sum(map(int, prices))
print(f"The total price is {total_price} dollars.") # 输出:The total price is 75 dollars.
动态生成包含数值的字符串
我们还可以根据数值动态生成字符串。例如,生成一个带有序号的列表:
items = ["apple", "banana", "cherry"]
for i, item in enumerate(items, start=1):
print(f"{i}. {item}")
输出:
1. apple
2. banana
3. cherry
处理带有单位的数值
在处理带有单位的数值时,我们可以使用正则表达式提取数值和单位,然后进行相应的处理:
import re
text = "The file size is 15MB and the download speed is 2.5MB/s"
matches = re.findall(r'(\d+\.\d+|\d+)([a-zA-Z/]+)', text)
for match in matches:
value, unit = match
print(f"Value: {value}, Unit: {unit}")
输出:
Value: 15, Unit: MB
Value: 2.5, Unit: MB/s
在这个例子中,我们提取了数值和单位,并分别打印出来。这种方法可以帮助我们更好地处理和理解带有单位的数值数据。
五、综合应用
通过结合前面介绍的技术,我们可以在实际应用中灵活地处理字符串和数字,解决各种复杂的数据处理需求。
解析和计算数据
例如,我们可能会遇到需要解析和计算数据的情况:
import re
text = """
Item: Apple, Quantity: 10, Price: 1.5
Item: Banana, Quantity: 5, Price: 0.8
Item: Cherry, Quantity: 20, Price: 0.2
"""
items = re.findall(r'Item: (\w+), Quantity: (\d+), Price: (\d+\.\d+)', text)
total_cost = 0
for item in items:
name, quantity, price = item
cost = int(quantity) * float(price)
total_cost += cost
print(f"Item: {name}, Quantity: {quantity}, Price: {price}, Cost: {cost}")
print(f"Total cost: {total_cost}")
输出:
Item: Apple, Quantity: 10, Price: 1.5, Cost: 15.0
Item: Banana, Quantity: 5, Price: 0.8, Cost: 4.0
Item: Cherry, Quantity: 20, Price: 0.2, Cost: 4.0
Total cost: 23.0
在这个例子中,我们解析了包含商品信息的字符串,并计算了每种商品的总成本和总成本。
动态生成报告
我们还可以根据数据动态生成报告,例如生成一个包含统计信息的报告:
items = [
{"name": "Apple", "quantity": 10, "price": 1.5},
{"name": "Banana", "quantity": 5, "price": 0.8},
{"name": "Cherry", "quantity": 20, "price": 0.2}
]
report = "Item Report:\n"
total_cost = 0
for item in items:
name = item["name"]
quantity = item["quantity"]
price = item["price"]
cost = quantity * price
total_cost += cost
report += f"Item: {name}, Quantity: {quantity}, Price: {price}, Cost: {cost}\n"
report += f"Total cost: {total_cost}\n"
print(report)
输出:
Item Report:
Item: Apple, Quantity: 10, Price: 1.5, Cost: 15.0
Item: Banana, Quantity: 5, Price: 0.8, Cost: 4.0
Item: Cherry, Quantity: 20, Price: 0.2, Cost: 4.0
Total cost: 23.0
在这个例子中,我们根据商品数据生成了一个包含统计信息的报告。
通过结合类型转换、字符串格式化、正则表达式处理等技术,我们可以灵活地处理字符串和数字,解决各种复杂的数据处理需求。无论是解析数据、计算数值,还是动态生成报告,这些技术都可以帮助我们高效地完成任务。
相关问答FAQs:
在Python中,如何将字符串和数字进行组合处理?
在Python中,可以使用字符串格式化方法将字符串和数字结合在一起。常用的格式化方法包括f-string(格式化字符串),str.format()
,和百分号(%)格式化。例如,使用f-string可以这样写:name = "Alice"; age = 30; result = f"{name} is {age} years old."
。这会生成字符串"Alice is 30 years old."。
如何在Python中对字符串和数字进行数学运算?
在Python中,字符串和数字不能直接进行数学运算。不过,可以通过将字符串转换为数字来实现。例如,使用int()
或float()
函数将字符串转换为相应的数字类型,如result = int("5") + 3
,结果将是8。确保字符串内容可转换为数字,否则会引发错误。
在处理字符串和数字时,如何避免类型错误?
为了避免类型错误,始终在进行操作前检查数据类型。可以使用isinstance()
函数来判断变量类型。例如,if isinstance(variable, str):
可以确保变量是字符串。如果需要进行运算,确保将字符串转换为数字。添加异常处理机制,如try...except
块,可以帮助捕捉并处理潜在的转换错误,确保程序的稳定性。
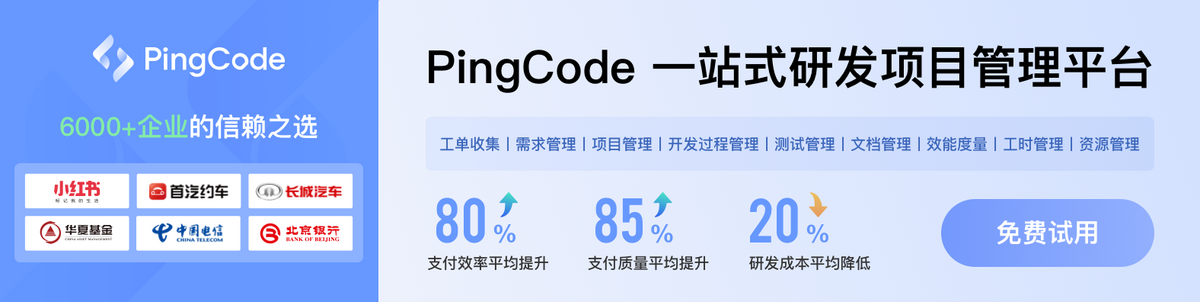