在Python中,可以使用多种方法将几个文件夹里的图片进行操作和处理,其中包括合并图片、移动图片、复制图片等。下面,我们将详细介绍如何使用Python来将几个文件夹里的图片进行操作,并提供代码示例和解释。
一、导入必要的库
在进行图片操作之前,我们需要导入一些必要的Python库。常用的库包括os
、shutil
和PIL
(Python Imaging Library)。这些库可以帮助我们处理文件系统和图片。
import os
import shutil
from PIL import Image
二、获取文件夹中的图片列表
首先,我们需要获取指定文件夹中的所有图片文件。可以使用os.listdir()
函数来获取文件夹中的所有文件,然后过滤出图片文件。
def get_image_files(folder_path):
image_extensions = ['.jpg', '.jpeg', '.png', '.gif', '.bmp']
return [f for f in os.listdir(folder_path) if os.path.splitext(f)[1].lower() in image_extensions]
三、合并文件夹中的图片
如果我们想将几个文件夹中的图片合并到一个文件夹中,可以使用shutil.copy2()
函数将图片文件复制到目标文件夹。
def merge_folders(source_folders, destination_folder):
if not os.path.exists(destination_folder):
os.makedirs(destination_folder)
for folder in source_folders:
image_files = get_image_files(folder)
for image_file in image_files:
source_path = os.path.join(folder, image_file)
destination_path = os.path.join(destination_folder, image_file)
shutil.copy2(source_path, destination_path)
四、重命名图片文件
在合并文件夹中的图片时,可能会遇到文件名冲突的问题。可以在复制图片时对文件名进行重命名,以避免冲突。
def merge_and_rename_folders(source_folders, destination_folder):
if not os.path.exists(destination_folder):
os.makedirs(destination_folder)
file_index = 1
for folder in source_folders:
image_files = get_image_files(folder)
for image_file in image_files:
source_path = os.path.join(folder, image_file)
new_file_name = f'image_{file_index}{os.path.splitext(image_file)[1]}'
destination_path = os.path.join(destination_folder, new_file_name)
shutil.copy2(source_path, destination_path)
file_index += 1
五、示例代码
下面是一个完整的示例代码,将多个文件夹中的图片合并到一个文件夹中,并对文件名进行重命名。
import os
import shutil
from PIL import Image
def get_image_files(folder_path):
image_extensions = ['.jpg', '.jpeg', '.png', '.gif', '.bmp']
return [f for f in os.listdir(folder_path) if os.path.splitext(f)[1].lower() in image_extensions]
def merge_and_rename_folders(source_folders, destination_folder):
if not os.path.exists(destination_folder):
os.makedirs(destination_folder)
file_index = 1
for folder in source_folders:
image_files = get_image_files(folder)
for image_file in image_files:
source_path = os.path.join(folder, image_file)
new_file_name = f'image_{file_index}{os.path.splitext(image_file)[1]}'
destination_path = os.path.join(destination_folder, new_file_name)
shutil.copy2(source_path, destination_path)
file_index += 1
示例文件夹路径
source_folders = ['folder1', 'folder2', 'folder3']
destination_folder = 'merged_folder'
合并并重命名图片
merge_and_rename_folders(source_folders, destination_folder)
六、批量处理图片
除了合并图片,Python还可以用来批量处理图片,比如调整图片大小、转换图片格式等。
调整图片大小
def resize_images_in_folder(folder_path, new_size):
image_files = get_image_files(folder_path)
for image_file in image_files:
image_path = os.path.join(folder_path, image_file)
with Image.open(image_path) as img:
img = img.resize(new_size)
img.save(image_path)
调整合并后的图片大小
resize_images_in_folder(destination_folder, (800, 600))
转换图片格式
def convert_images_in_folder(folder_path, new_format):
image_files = get_image_files(folder_path)
for image_file in image_files:
image_path = os.path.join(folder_path, image_file)
with Image.open(image_path) as img:
new_image_path = os.path.splitext(image_path)[0] + new_format
img.save(new_image_path)
将合并后的图片转换为JPEG格式
convert_images_in_folder(destination_folder, '.jpg')
七、总结
通过使用Python,我们可以轻松地将多个文件夹中的图片进行操作和处理。主要步骤包括获取图片列表、合并图片、重命名图片、调整图片大小和转换图片格式。这些操作可以帮助我们更好地管理和处理大量图片文件,提高工作效率。
以上代码示例展示了如何使用Python的os
、shutil
和PIL
库来实现这些操作。根据具体需求,还可以扩展和自定义这些功能,以满足不同的应用场景。
相关问答FAQs:
如何使用Python批量处理多个文件夹中的图片?
在Python中,您可以使用os
库和PIL
(Pillow)库来处理多个文件夹中的图片。首先,您需要遍历文件夹,找到所有的图片文件。接着,您可以对这些图片进行处理,例如调整大小、转换格式等。以下是一个简单的示例代码:
import os
from PIL import Image
def process_images_in_folders(root_folder):
for foldername, subfolders, filenames in os.walk(root_folder):
for filename in filenames:
if filename.endswith(('.png', '.jpg', '.jpeg')):
image_path = os.path.join(foldername, filename)
with Image.open(image_path) as img:
img = img.resize((800, 600)) # 调整大小
img.save(os.path.join(foldername, 'processed_' + filename))
process_images_in_folders('您的文件夹路径')
在Python中如何读取和显示多个文件夹中的图片?
要读取和显示多个文件夹中的图片,您可以使用matplotlib
库结合PIL
库。您可以遍历指定的文件夹,读取每一张图片并使用imshow
函数显示出来。下面是一个示例代码:
import os
from PIL import Image
import matplotlib.pyplot as plt
def show_images_in_folders(root_folder):
for foldername, subfolders, filenames in os.walk(root_folder):
for filename in filenames:
if filename.endswith(('.png', '.jpg', '.jpeg')):
image_path = os.path.join(foldername, filename)
img = Image.open(image_path)
plt.imshow(img)
plt.axis('off') # 不显示坐标轴
plt.show()
show_images_in_folders('您的文件夹路径')
如何使用Python将多个文件夹中的图片转换为另一种格式?
使用Python将多个文件夹中的图片转换格式相对简单。您同样可以利用os
和PIL
库来实现。只需遍历文件夹,打开图片,并将其保存为所需格式。以下是一个示例,演示如何将所有JPEG格式的图片转换为PNG格式:
import os
from PIL import Image
def convert_images_to_png(root_folder):
for foldername, subfolders, filenames in os.walk(root_folder):
for filename in filenames:
if filename.endswith('.jpg') or filename.endswith('.jpeg'):
image_path = os.path.join(foldername, filename)
img = Image.open(image_path)
png_path = os.path.join(foldername, filename.rsplit('.', 1)[0] + '.png')
img.save(png_path)
convert_images_to_png('您的文件夹路径')
这些示例代码展示了如何在Python中处理和操作多个文件夹中的图片,您可以根据需要进行调整和扩展。
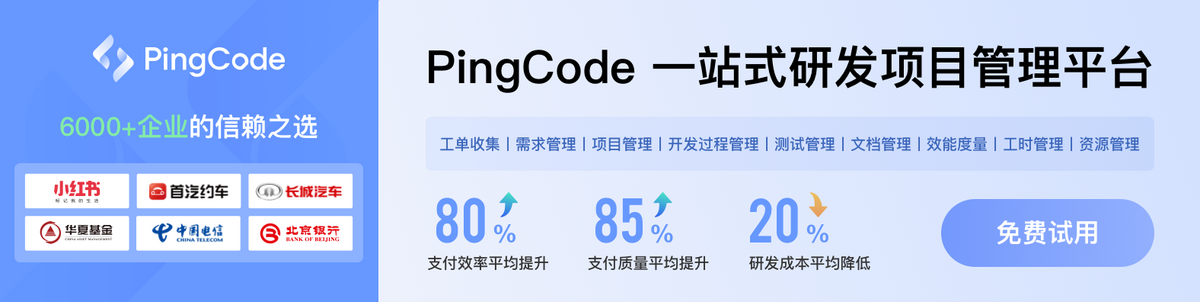