如何将图片剪裁成小图片python
要将图片剪裁成小图片,你可以使用Python中的Pillow库。使用Pillow库、指定剪裁区域、循环剪裁图像、保存剪裁后的图像。其中,Pillow库是实现这一操作的关键。通过Pillow库,你可以方便地加载、处理和保存图像。下面将详细介绍如何使用Pillow库将图片剪裁成小图片。
一、安装Pillow库
要开始处理图像,首先需要安装Pillow库。可以使用以下命令进行安装:
pip install Pillow
二、加载图像
首先,使用Pillow库的Image
模块加载图像。以下是加载图像的示例代码:
from PIL import Image
加载图像
image = Image.open('path_to_your_image.jpg')
三、指定剪裁区域
在剪裁图像之前,需要指定剪裁区域。可以使用crop
方法来指定剪裁区域,该方法接受一个元组,包含左、上、右、下四个坐标。以下是一个简单的示例:
# 指定剪裁区域
left = 100
top = 100
right = 400
bottom = 400
剪裁图像
cropped_image = image.crop((left, top, right, bottom))
四、循环剪裁图像
如果需要将图像剪裁成多个小图片,可以使用循环。以下是一个示例,假设将图像剪裁成大小相等的小图片:
import os
定义剪裁函数
def crop_image(image, crop_width, crop_height, output_dir):
image_width, image_height = image.size
num_crops_x = image_width // crop_width
num_crops_y = image_height // crop_height
# 创建输出目录
if not os.path.exists(output_dir):
os.makedirs(output_dir)
crop_count = 0
for i in range(num_crops_x):
for j in range(num_crops_y):
left = i * crop_width
top = j * crop_height
right = left + crop_width
bottom = top + crop_height
cropped_image = image.crop((left, top, right, bottom))
cropped_image.save(os.path.join(output_dir, f'crop_{crop_count}.jpg'))
crop_count += 1
加载图像
image = Image.open('path_to_your_image.jpg')
设置剪裁参数
crop_width = 100
crop_height = 100
output_dir = 'cropped_images'
剪裁图像
crop_image(image, crop_width, crop_height, output_dir)
五、保存剪裁后的图像
在剪裁图像后,使用save
方法将剪裁后的图像保存到指定的文件路径。以下是保存图像的示例代码:
# 保存剪裁后的图像
cropped_image.save('path_to_save_cropped_image.jpg')
六、处理不同尺寸的图像
有时你可能需要处理不同尺寸的图像,可以使用Pillow库的resize
方法将图像调整到指定尺寸。以下是一个示例:
# 调整图像大小
resized_image = image.resize((800, 600))
保存调整大小后的图像
resized_image.save('path_to_save_resized_image.jpg')
七、添加边框和其他图像处理
Pillow库还提供了其他图像处理功能,例如添加边框、旋转图像等。以下是一些示例代码:
# 添加边框
from PIL import ImageOps
添加边框
border_image = ImageOps.expand(image, border=10, fill='black')
保存添加边框后的图像
border_image.save('path_to_save_border_image.jpg')
旋转图像
rotated_image = image.rotate(45)
保存旋转后的图像
rotated_image.save('path_to_save_rotated_image.jpg')
八、处理不同图像格式
Pillow库支持多种图像格式,例如JPEG、PNG、BMP等。在加载和保存图像时,可以指定图像格式。以下是一些示例代码:
# 加载PNG格式的图像
image = Image.open('path_to_your_image.png')
保存为JPEG格式
image.save('path_to_save_image.jpg', format='JPEG')
保存为BMP格式
image.save('path_to_save_image.bmp', format='BMP')
九、处理透明背景
对于具有透明背景的图像(如PNG格式),可以使用Pillow库处理透明背景。以下是一个示例:
# 加载具有透明背景的图像
image = Image.open('path_to_your_image_with_transparent_background.png')
剪裁图像
left = 50
top = 50
right = 250
bottom = 250
cropped_image = image.crop((left, top, right, bottom))
保存剪裁后的图像
cropped_image.save('path_to_save_cropped_image_with_transparent_background.png', format='PNG')
十、批量处理图像
如果需要批量处理多个图像,可以使用循环批量加载和处理图像。以下是一个示例:
import glob
获取所有图像文件路径
image_paths = glob.glob('path_to_your_images/*.jpg')
批量处理图像
for image_path in image_paths:
image = Image.open(image_path)
# 剪裁图像
cropped_image = image.crop((left, top, right, bottom))
# 保存剪裁后的图像
cropped_image.save(f'path_to_save_cropped_images/cropped_{os.path.basename(image_path)}')
十一、图像增强
Pillow库还提供了图像增强功能,例如调整亮度、对比度、颜色等。以下是一些示例代码:
from PIL import ImageEnhance
调整亮度
enhancer = ImageEnhance.Brightness(image)
bright_image = enhancer.enhance(1.5)
保存调整亮度后的图像
bright_image.save('path_to_save_bright_image.jpg')
调整对比度
enhancer = ImageEnhance.Contrast(image)
contrast_image = enhancer.enhance(1.5)
保存调整对比度后的图像
contrast_image.save('path_to_save_contrast_image.jpg')
调整颜色
enhancer = ImageEnhance.Color(image)
color_image = enhancer.enhance(1.5)
保存调整颜色后的图像
color_image.save('path_to_save_color_image.jpg')
十二、图像滤镜
Pillow库还提供了各种图像滤镜,例如模糊、锐化等。以下是一些示例代码:
from PIL import ImageFilter
应用模糊滤镜
blur_image = image.filter(ImageFilter.BLUR)
保存应用模糊滤镜后的图像
blur_image.save('path_to_save_blur_image.jpg')
应用锐化滤镜
sharp_image = image.filter(ImageFilter.SHARPEN)
保存应用锐化滤镜后的图像
sharp_image.save('path_to_save_sharp_image.jpg')
通过以上步骤,你可以使用Python中的Pillow库将图片剪裁成小图片,并进行各种图像处理。Pillow库功能强大且易于使用,是处理图像的理想选择。希望这篇文章对你有所帮助。
相关问答FAQs:
如何在Python中使用PIL库进行图片剪裁?
使用Python的Pillow库(PIL的分支)可以轻松实现图片剪裁。首先,需要安装Pillow库,可以通过命令pip install Pillow
进行安装。剪裁图片的基本步骤是打开图片,定义剪裁区域的坐标,然后使用crop()
方法进行剪裁。以下是一个简单的示例代码:
from PIL import Image
# 打开图片
image = Image.open('your_image.jpg')
# 定义剪裁区域 (左,上,右,下)
crop_area = (100, 100, 400, 400)
# 剪裁图片
cropped_image = image.crop(crop_area)
# 保存剪裁后的图片
cropped_image.save('cropped_image.jpg')
这样,你就可以根据需要剪裁出指定区域的小图片。
在Python中如何处理多张图片的批量剪裁?
如果需要对多张图片进行剪裁,可以使用循环来处理。首先,将所有需要剪裁的图片放在一个文件夹中,然后利用os
库遍历文件夹中的图片文件。以下是一个示例代码:
import os
from PIL import Image
# 定义图片文件夹路径
folder_path = 'your_images_folder'
# 遍历文件夹中的每一张图片
for filename in os.listdir(folder_path):
if filename.endswith('.jpg') or filename.endswith('.png'):
image = Image.open(os.path.join(folder_path, filename))
crop_area = (100, 100, 400, 400) # 定义剪裁区域
cropped_image = image.crop(crop_area)
cropped_image.save(os.path.join(folder_path, 'cropped_' + filename))
这样,每张图片都会被剪裁并保存为新文件,便于管理和使用。
如何调整剪裁区域以适应不同尺寸的图片?
在剪裁图片时,可能需要根据不同的图片尺寸来调整剪裁区域。可以通过获取图片的宽度和高度来动态设置剪裁区域。例如,可以根据图片的中心位置进行剪裁。以下是一个示例代码:
from PIL import Image
def crop_center(image, crop_width, crop_height):
img_width, img_height = image.size
left = (img_width - crop_width) / 2
top = (img_height - crop_height) / 2
right = (img_width + crop_width) / 2
bottom = (img_height + crop_height) / 2
return image.crop((left, top, right, bottom))
# 使用示例
image = Image.open('your_image.jpg')
cropped_image = crop_center(image, 300, 300) # 剪裁中心区域
cropped_image.save('center_cropped_image.jpg')
这种方法可以确保不同尺寸的图片都能获得所需的剪裁效果,适用于多种应用场景。
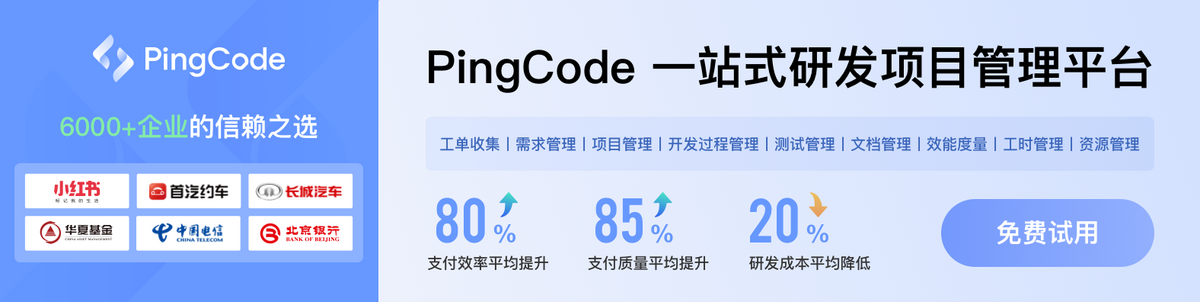