Python可以根据地址下载图片到本地。 主要方法包括使用requests
库、urllib
库、以及wget
库等。使用requests
库是其中最常见的一种方法, 因为它简单且功能强大。安装requests
库、发送HTTP请求获取图片数据、将图片数据写入本地文件是使用requests
库下载图片的核心步骤。接下来,我们将详细介绍这些步骤,并讨论其他几种方法。
一、使用Requests库下载图片
1. 安装和导入Requests库
首先,你需要确保已经安装了requests
库。如果没有安装,可以使用以下命令进行安装:
pip install requests
然后,在你的Python脚本中导入requests
库:
import requests
2. 发送HTTP请求获取图片数据
使用requests.get
方法发送HTTP请求,获取图片数据:
response = requests.get('https://example.com/image.jpg')
3. 将图片数据写入本地文件
在获取到图片数据后,你需要将其写入本地文件。可以使用with open
方法来创建并写入文件:
with open('image.jpg', 'wb') as file:
file.write(response.content)
二、使用Urllib库下载图片
1. 导入Urllib库
urllib
库是Python自带的库,不需要额外安装。你可以直接在脚本中导入:
import urllib.request
2. 下载图片并保存到本地
使用urllib.request.urlretrieve
方法下载图片并保存:
urllib.request.urlretrieve('https://example.com/image.jpg', 'image.jpg')
三、使用Wget库下载图片
1. 安装和导入Wget库
如果你没有安装wget
库,可以使用以下命令进行安装:
pip install wget
然后,在你的Python脚本中导入wget
库:
import wget
2. 下载图片并保存到本地
使用wget.download
方法下载图片并保存:
wget.download('https://example.com/image.jpg', 'image.jpg')
四、使用PIL库处理下载的图片
有时候,你可能需要对下载的图片进行处理,例如调整大小、裁剪等。可以使用PIL
库(也称为Pillow
)来完成这些操作。
1. 安装和导入PIL库
如果你没有安装PIL
库,可以使用以下命令进行安装:
pip install pillow
然后,在你的Python脚本中导入PIL
库:
from PIL import Image
2. 打开并处理图片
使用Image.open
方法打开图片,并进行相应的处理:
img = Image.open('image.jpg')
img_resized = img.resize((200, 200))
img_resized.save('image_resized.jpg')
五、处理下载图片的错误和异常
在下载图片时,你可能会遇到各种错误和异常。例如,网络连接问题、URL无效、文件写入权限等。你可以使用try
和except
块来捕获并处理这些异常。
try:
response = requests.get('https://example.com/image.jpg')
response.raise_for_status() # 检查是否有HTTP错误
with open('image.jpg', 'wb') as file:
file.write(response.content)
except requests.exceptions.RequestException as e:
print(f"Error downloading image: {e}")
六、批量下载图片
如果你需要批量下载多个图片,可以使用循环和列表来实现。例如:
urls = [
'https://example.com/image1.jpg',
'https://example.com/image2.jpg',
'https://example.com/image3.jpg'
]
for url in urls:
try:
response = requests.get(url)
response.raise_for_status()
file_name = url.split('/')[-1]
with open(file_name, 'wb') as file:
file.write(response.content)
except requests.exceptions.RequestException as e:
print(f"Error downloading {url}: {e}")
七、保存图片到指定目录
有时候,你可能需要将图片保存到特定的目录。你可以使用os
库来创建目录并保存图片。
1. 导入OS库
import os
2. 创建目录并保存图片
directory = 'images'
if not os.path.exists(directory):
os.makedirs(directory)
url = 'https://example.com/image.jpg'
response = requests.get(url)
file_name = os.path.join(directory, url.split('/')[-1])
with open(file_name, 'wb') as file:
file.write(response.content)
八、总结
通过上述方法,你可以使用Python根据地址下载图片到本地。无论是使用requests
库、urllib
库,还是wget
库,每种方法都有其优点和适用场景。你可以根据具体需求选择合适的方法。此外,还可以结合PIL
库对下载的图片进行处理,并处理下载过程中可能遇到的错误和异常。通过批量下载和保存图片到指定目录,你可以更高效地管理和处理图片数据。
相关问答FAQs:
如何在Python中指定下载图片的保存路径?
在Python中,可以使用requests
库下载图片,并通过指定文件路径将其保存到本地。首先,确保安装了requests
库。使用open()
函数配合'wb'
模式可以创建并写入文件。例如,使用以下代码可以将图片保存到指定的文件夹:
import requests
url = '图片链接'
response = requests.get(url)
with open('保存路径/图片名.jpg', 'wb') as file:
file.write(response.content)
确保替换图片链接
和保存路径/图片名.jpg
为实际的链接和文件名。
下载图片时是否需要处理异常情况?
在下载图片的过程中,网络问题或无效的链接可能会导致错误。因此,使用try-except
语句捕获异常是一个好习惯。可以通过检查响应的状态码来判断请求是否成功。例如:
try:
response = requests.get(url)
response.raise_for_status() # 如果请求失败,将引发HTTPError
except requests.exceptions.RequestException as e:
print(f"下载图片时发生错误: {e}")
这样可以确保程序在遇到问题时不会崩溃,而是给出相应的错误提示。
是否可以批量下载多个图片?
当然可以。可以将图片链接存储在列表中,并通过循环遍历列表来下载每个图片。以下是一个简单的示例代码:
urls = ['图片链接1', '图片链接2', '图片链接3'] # 替换为实际的链接
for index, url in enumerate(urls):
try:
response = requests.get(url)
response.raise_for_status()
with open(f'保存路径/图片{index + 1}.jpg', 'wb') as file:
file.write(response.content)
except requests.exceptions.RequestException as e:
print(f"下载图片 {url} 时发生错误: {e}")
这种方法可以高效地下载多个图片,并为每个图片命名。
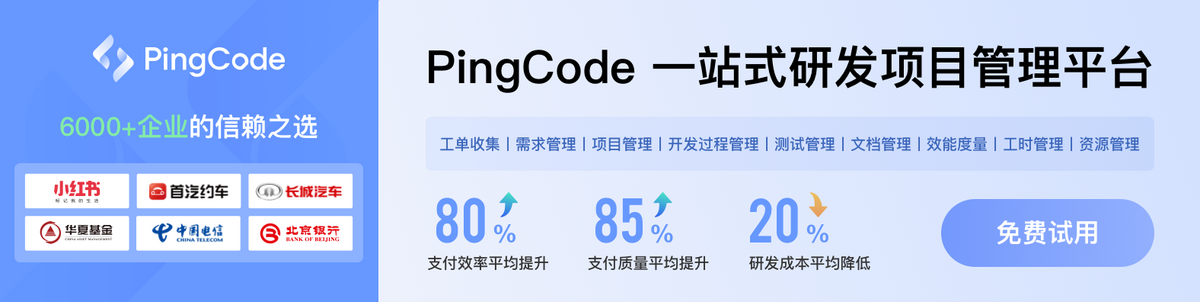