Python 中可以通过几种方法来转换千分位数字,例如使用字符串格式化、locale 模块以及自定义函数。 使用字符串格式化方法最为简洁,而 locale 模块则提供了更强的国际化支持。下面详细描述一种使用字符串格式化方法来实现千分位转换的方法。
一、字符串格式化
使用 Python 的字符串格式化可以非常简单地实现千分位分隔。这里主要使用 format
方法和 f-string
。
1、使用 format
方法
Python 提供了一个内置的 format
方法,可以方便地将数字格式化为带有千分位分隔符的字符串。
num = 1234567890
formatted_num = "{:,}".format(num)
print(formatted_num)
在上面的代码中,{:,}
是格式化字符串的占位符,它告诉 Python 将数字格式化为带有千分位分隔符的字符串。输出结果为:
1,234,567,890
2、使用 f-string
Python 3.6 及以上版本引入了 f-string(格式化字符串字面量),它使字符串格式化更加简洁和直观。
num = 1234567890
formatted_num = f"{num:,}"
print(formatted_num)
同样,输出结果为:
1,234,567,890
二、使用 locale 模块
Python 的 locale
模块提供了全面的国际化支持,可以根据不同的区域设置来格式化数字。
1、设置区域
首先,需要设置适当的区域。下面示例使用美国区域设置。
import locale
locale.setlocale(locale.LC_ALL, 'en_US.UTF-8')
2、格式化数字
使用 locale.format_string
方法来格式化数字。
num = 1234567890
formatted_num = locale.format_string("%d", num, grouping=True)
print(formatted_num)
输出结果为:
1,234,567,890
三、自定义函数
如果希望更灵活地控制数字格式化,还可以编写自定义函数来实现千分位分隔。
def format_number_with_commas(number):
if isinstance(number, int):
return f"{number:,}"
elif isinstance(number, float):
integer_part, decimal_part = f"{number}".split(".")
return f"{int(integer_part):,}.{decimal_part}"
else:
raise ValueError("Input must be an integer or float.")
num1 = 1234567890
num2 = 1234567890.12345
formatted_num1 = format_number_with_commas(num1)
formatted_num2 = format_number_with_commas(num2)
print(formatted_num1)
print(formatted_num2)
输出结果为:
1,234,567,890
1,234,567,890.12345
四、实践应用
1、在数据展示中的应用
在数据展示中,特别是与用户交互的界面中,清晰易读的数字格式是至关重要的。通过对数据进行千分位格式化,可以提高数据的可读性和美观度。
import pandas as pd
data = {
'Sales': [123456, 2345678, 34567890]
}
df = pd.DataFrame(data)
df['Sales'] = df['Sales'].apply(lambda x: f"{x:,}")
print(df)
输出结果为:
Sales
0 123,456
1 2,345,678
2 34,567,890
2、在数据分析中的应用
在数据分析中,通过对数据进行千分位格式化,可以更直观地理解数值的大小和趋势。
import matplotlib.pyplot as plt
sales = [123456, 2345678, 34567890]
years = ['2021', '2022', '2023']
plt.plot(years, sales)
plt.title('Sales Over Years')
plt.xlabel('Year')
plt.ylabel('Sales')
设置 y 轴格式
plt.gca().get_yaxis().set_major_formatter(
plt.FuncFormatter(lambda x, loc: f"{int(x):,}")
)
plt.show()
通过以上代码,可以生成一个带有千分位分隔符的折线图,使数值更加清晰易读。
五、总结
通过字符串格式化、locale 模块以及自定义函数,可以在 Python 中轻松实现千分位格式化。 这些方法各有优缺点,适用于不同的应用场景。字符串格式化方法简洁明了,适合快速处理;locale 模块提供了更强的国际化支持,适合处理多语言环境;自定义函数则提供了最大的灵活性,适合需要复杂格式化的场景。在实际应用中,可以根据具体需求选择合适的方法来实现千分位格式化。
相关问答FAQs:
如何在Python中将数字格式化为千分位?
在Python中,可以使用内置的format()
函数或f字符串来将数字格式化为千分位。例如,format(1234567, ',')
将返回'1,234,567'
。使用f字符串时,可以这样写:f"{1234567:,}"
,效果相同。这样可以轻松地将任何数字转换为千分位格式。
Python中是否有库可以简化千分位数字转换?
是的,Python的locale
模块可以帮助你根据不同地区的千分位规则进行数字格式化。使用locale.setlocale(locale.LC_ALL, 'en_US.UTF-8')
后,调用locale.format_string("%d", 1234567, grouping=True)
可以得到'1,234,567'
。这对于处理国际化的数字格式非常有用。
如何处理浮点数的千分位格式化?
对于浮点数,可以使用相同的方法进行格式化。在format()
函数中使用:,
来实现。例如,format(1234567.89, ',')
会返回'1,234,567.89'
。使用f字符串时,写法为f"{1234567.89:,.2f}"
,可以指定小数点后保留两位。这样可以确保数字在视觉上更易于阅读。
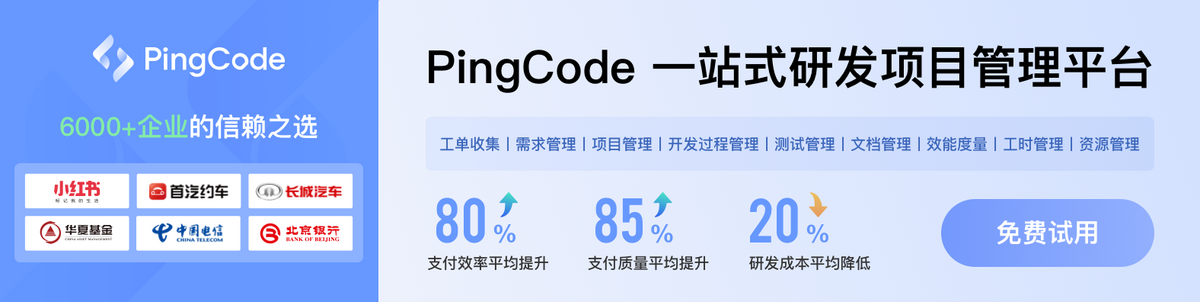