如何检查python爬虫库是否安装成功
检查Python爬虫库是否安装成功的核心步骤包括:使用命令行检查、尝试导入库、运行简单代码。其中最直接的方法是通过命令行输入命令来检查库是否已经成功安装。
使用命令行检查
在终端或命令提示符中输入以下命令,可以查看是否安装了特定的Python爬虫库:
pip show requests
pip show scrapy
pip show beautifulsoup4
如果库已经安装成功,会显示该库的详细信息,如版本号、依赖项等。如果库未安装,则不会显示任何信息或者提示找不到该库。这个方法不仅能确认库是否安装,还能检查安装的版本信息等。
一、使用命令行检查
使用命令行是最直接的方法之一。在终端或命令提示符中输入以下命令,可以查看是否安装了特定的Python爬虫库。
1. 检查requests
库
requests
库是一个非常流行的HTTP库,通常用于发送HTTP请求。要检查是否安装了requests
库,可以在命令行输入以下命令:
pip show requests
如果库已经成功安装,会显示如下信息:
Name: requests
Version: 2.25.1
Summary: Python HTTP for Humans.
Home-page: https://requests.readthedocs.io
Author: Kenneth Reitz
Author-email: me@kennethreitz.org
License: Apache 2.0
Location: /usr/local/lib/python3.8/dist-packages
Requires: certifi, chardet, idna, urllib3
如果没有安装,则会提示找不到该库。
2. 检查Scrapy
库
Scrapy
是一个功能强大的爬虫框架,适用于构建大规模爬虫项目。要检查是否安装了Scrapy
库,可以使用如下命令:
pip show scrapy
成功安装后会显示如下信息:
Name: Scrapy
Version: 2.5.0
Summary: A high-level Web Crawling and Web Scraping framework
Home-page: https://scrapy.org
Author: Scrapy developers
Author-email: scrapy@googlegroups.com
License: BSD
Location: /usr/local/lib/python3.8/dist-packages
Requires: Twisted, w3lib, queuelib, lxml, parsel, PyDispatcher, service-identity, protego, itemadapter
3. 检查BeautifulSoup
库
BeautifulSoup
是一个用于解析HTML和XML的库,通常与requests
库配合使用。要检查是否安装了BeautifulSoup
库,可以使用如下命令:
pip show beautifulsoup4
成功安装后会显示如下信息:
Name: beautifulsoup4
Version: 4.9.3
Summary: Screen-scraping library
Home-page: http://www.crummy.com/software/BeautifulSoup/bs4/
Author: Leonard Richardson
Author-email: leonardr@segfault.org
License: MIT
Location: /usr/local/lib/python3.8/dist-packages
Requires: soupsieve
二、尝试导入库
另一个检查库是否安装成功的方法是尝试在Python脚本中导入该库。如果没有安装或安装不成功,导入时会报错。以下是示例代码:
try:
import requests
print("requests库安装成功")
except ImportError:
print("requests库未安装")
try:
import scrapy
print("Scrapy库安装成功")
except ImportError:
print("Scrapy库未安装")
try:
from bs4 import BeautifulSoup
print("BeautifulSoup库安装成功")
except ImportError:
print("BeautifulSoup库未安装")
运行以上代码,如果没有出现ImportError
,则说明这些库已经成功安装。
三、运行简单代码
为了进一步确认库是否正常工作,可以运行一些简单的代码来测试其功能。例如,使用requests
库发送一个HTTP请求,或者使用BeautifulSoup
库解析一个HTML文档。
1. 使用requests
库发送HTTP请求
import requests
response = requests.get('https://www.example.com')
if response.status_code == 200:
print("requests库工作正常")
else:
print("requests库出现问题")
2. 使用BeautifulSoup
库解析HTML文档
from bs4 import BeautifulSoup
html_doc = """
<html>
<head><title>Test Page</title></head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
</body>
</html>
"""
soup = BeautifulSoup(html_doc, 'html.parser')
print(soup.title.string) # 输出: Test Page
print(soup.find_all('a')) # 输出: [<a class="sister" href="http://example.com/elsie" id="link1">Elsie</a>, <a class="sister" href="http://example.com/lacie" id="link2">Lacie</a>, <a class="sister" href="http://example.com/tillie" id="link3">Tillie</a>]
print("BeautifulSoup库工作正常")
3. 使用Scrapy
库创建一个简单的爬虫
import scrapy
from scrapy.crawler import CrawlerProcess
class ExampleSpider(scrapy.Spider):
name = "example"
start_urls = ['https://www.example.com']
def parse(self, response):
self.log('访问的URL: %s' % response.url)
process = CrawlerProcess()
process.crawl(ExampleSpider)
process.start()
print("Scrapy库工作正常")
四、检查虚拟环境
在开发Python项目时,建议使用虚拟环境来管理依赖项。虚拟环境可以确保项目之间的依赖项不会相互干扰。要检查虚拟环境中是否安装了特定的库,可以激活虚拟环境后使用上述方法。
1. 创建虚拟环境
python3 -m venv myenv
2. 激活虚拟环境
在Windows上:
myenv\Scripts\activate
在macOS和Linux上:
source myenv/bin/activate
3. 安装依赖项
pip install requests scrapy beautifulsoup4
4. 检查安装情况
激活虚拟环境后,可以使用上述命令检查库是否安装成功:
pip show requests
pip show scrapy
pip show beautifulsoup4
五、使用工具检查依赖项
一些工具可以帮助我们管理和检查项目的依赖项。例如,pipreqs
可以生成项目的requirements.txt
文件,pipdeptree
可以显示项目的依赖项树。
1. 使用pipreqs
生成requirements.txt
文件
首先安装pipreqs
:
pip install pipreqs
然后在项目目录下运行:
pipreqs ./
这将生成一个包含所有项目依赖项的requirements.txt
文件。
2. 使用pipdeptree
显示依赖项树
首先安装pipdeptree
:
pip install pipdeptree
然后运行:
pipdeptree
这将显示项目的依赖项树,有助于检查是否所有依赖项都已正确安装。
六、检查项目配置文件
在一些项目中,依赖项可能定义在配置文件中,例如setup.py
、requirements.txt
或Pipfile
。确保这些文件中列出的所有依赖项都已正确安装。
1. 检查requirements.txt
文件
项目的requirements.txt
文件通常列出了所有的依赖项。可以使用以下命令安装文件中列出的所有依赖项:
pip install -r requirements.txt
2. 检查setup.py
文件
如果项目使用setup.py
来管理依赖项,可以查看文件中的install_requires
部分:
from setuptools import setup, find_packages
setup(
name='myproject',
version='1.0.0',
packages=find_packages(),
install_requires=[
'requests',
'scrapy',
'beautifulsoup4',
],
)
可以使用以下命令安装文件中列出的依赖项:
pip install .
3. 检查Pipfile
如果项目使用pipenv
来管理依赖项,可以查看Pipfile
:
[[source]]
name = "pypi"
url = "https://pypi.org/simple"
verify_ssl = true
[packages]
requests = "*"
scrapy = "*"
beautifulsoup4 = "*"
[dev-packages]
可以使用以下命令安装文件中列出的依赖项:
pipenv install
七、确保Python解释器正确
有时,可能会遇到多个Python解释器版本的问题,导致库安装在不同的解释器下。确保正在使用的Python解释器与安装库时使用的解释器一致。
1. 检查Python解释器路径
可以使用以下命令检查当前使用的Python解释器路径:
which python
在Windows上:
where python
2. 检查pip路径
可以使用以下命令检查当前使用的pip
路径:
which pip
在Windows上:
where pip
确保Python解释器和pip
路径一致。
八、总结
检查Python爬虫库是否安装成功的方法包括使用命令行检查、尝试导入库、运行简单代码、检查虚拟环境、使用工具检查依赖项、检查项目配置文件、确保Python解释器正确。通过这些方法,可以确保项目中所需的依赖项已经正确安装,并且可以正常工作。
相关问答FAQs:
如何确认我安装的Python爬虫库的版本?
要确认已安装的Python爬虫库版本,可以在终端或命令提示符中使用pip show <库名>
命令。例如,如果想查看BeautifulSoup的版本,可以输入pip show beautifulsoup4
。这将显示库的详细信息,包括版本号、安装位置和依赖关系。
如果我在安装爬虫库时遇到错误,应该怎么办?
如果在安装爬虫库时遇到错误,可以首先检查Python和pip的版本是否兼容。确保你的Python环境已经更新,并使用pip install --upgrade pip
命令来升级pip。查看错误信息,可能是缺少某些依赖库或权限问题,针对具体问题查找解决方案通常能够帮助你顺利安装。
是否有推荐的工具来管理Python库的安装和依赖?
使用虚拟环境管理工具如venv
或conda
可以更好地管理Python库的安装和依赖关系。虚拟环境允许你为每个项目创建独立的环境,避免库版本冲突。通过pip freeze
命令可以生成当前环境中所有安装库的列表,便于后续的管理和分享。
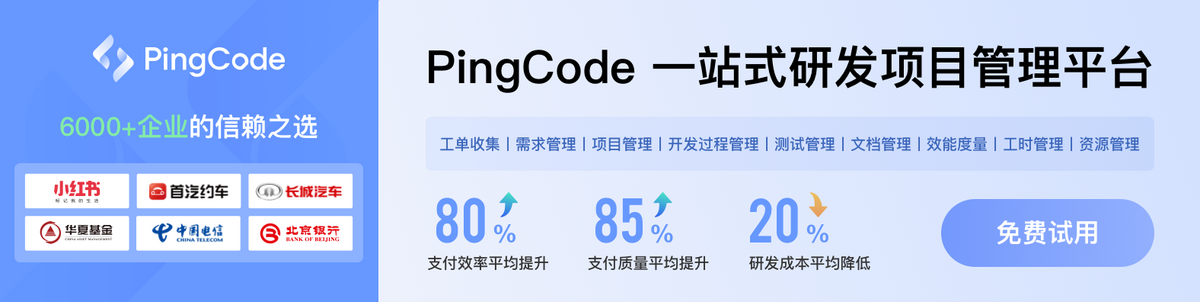