使用Python爬取网页数据通常需要以下几个步骤:选择合适的工具、发送HTTP请求、解析网页内容、提取所需数据。 其中,选择合适的工具是最为关键的一步,常见的工具包括Requests库、BeautifulSoup库、Selenium库等。下面将详细介绍如何在Python中爬取网页数据。
一、选择合适的工具
选择合适的工具是进行网页数据爬取的第一步。Python中常用的爬虫工具有以下几种:
1、Requests库
Requests库是一个简单易用的HTTP库,可以轻松地发送HTTP请求,获取网页内容。其主要功能包括发送GET和POST请求、携带请求参数、处理Cookies等。
2、BeautifulSoup库
BeautifulSoup是一个解析HTML和XML的库,可以轻松地提取网页中的数据。它支持多种解析器,如lxml、html.parser等,可以根据需求选择。
3、Selenium库
Selenium是一个用于自动化测试的工具,可以模拟浏览器行为,适用于处理动态加载的网页数据。通过Selenium,可以实现页面的点击、表单填写、滚动等操作。
4、Scrapy框架
Scrapy是一个功能强大的爬虫框架,适用于复杂的爬虫任务。它提供了丰富的功能模块,如请求调度、数据存储、爬虫中间件等,可以高效地进行数据爬取。
二、发送HTTP请求
发送HTTP请求是获取网页内容的关键步骤。通过Requests库,可以方便地发送GET和POST请求,获取网页的HTML代码。
1、发送GET请求
import requests
url = 'https://example.com'
response = requests.get(url)
print(response.text)
2、发送POST请求
import requests
url = 'https://example.com/login'
data = {'username': 'your_username', 'password': 'your_password'}
response = requests.post(url, data=data)
print(response.text)
3、处理请求参数
import requests
url = 'https://example.com/search'
params = {'q': 'python'}
response = requests.get(url, params=params)
print(response.text)
三、解析网页内容
解析网页内容是从HTML代码中提取所需数据的关键步骤。通过BeautifulSoup库,可以方便地解析HTML代码,提取所需数据。
1、解析HTML代码
from bs4 import BeautifulSoup
html = '<html><head><title>Example</title></head><body><h1>Hello, world!</h1></body></html>'
soup = BeautifulSoup(html, 'html.parser')
print(soup.prettify())
2、提取网页数据
from bs4 import BeautifulSoup
html = '<html><head><title>Example</title></head><body><h1>Hello, world!</h1></body></html>'
soup = BeautifulSoup(html, 'html.parser')
title = soup.title.string
heading = soup.h1.string
print('Title:', title)
print('Heading:', heading)
四、提取所需数据
提取所需数据是网页爬取的最终目标。通过BeautifulSoup库,可以根据标签、属性等条件,提取所需的数据。
1、根据标签提取数据
from bs4 import BeautifulSoup
html = '''<html><body><h1>Hello, world!</h1><p>This is a paragraph.</p></body></html>'''
soup = BeautifulSoup(html, 'html.parser')
heading = soup.find('h1').string
paragraph = soup.find('p').string
print('Heading:', heading)
print('Paragraph:', paragraph)
2、根据属性提取数据
from bs4 import BeautifulSoup
html = '''<html><body><div class="content">This is content.</div><div class="footer">This is footer.</div></body></html>'''
soup = BeautifulSoup(html, 'html.parser')
content = soup.find('div', {'class': 'content'}).string
footer = soup.find('div', {'class': 'footer'}).string
print('Content:', content)
print('Footer:', footer)
五、处理动态加载的网页数据
对于动态加载的网页数据,可以使用Selenium库进行处理。Selenium可以模拟浏览器行为,执行JavaScript代码,从而获取动态加载的数据。
1、安装Selenium和WebDriver
pip install selenium
2、使用Selenium获取动态加载的数据
from selenium import webdriver
url = 'https://example.com'
driver = webdriver.Chrome()
driver.get(url)
html = driver.page_source
print(html)
driver.quit()
3、模拟用户操作
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
url = 'https://example.com/login'
driver = webdriver.Chrome()
driver.get(url)
username_input = driver.find_element(By.NAME, 'username')
password_input = driver.find_element(By.NAME, 'password')
login_button = driver.find_element(By.NAME, 'login')
username_input.send_keys('your_username')
password_input.send_keys('your_password')
login_button.click()
html = driver.page_source
print(html)
driver.quit()
六、处理反爬虫机制
在实际爬虫过程中,可能会遇到反爬虫机制,如IP封禁、验证码等。为了应对这些问题,可以采取以下措施:
1、使用代理IP
使用代理IP可以隐藏真实IP,避免被封禁。可以通过代理池来动态切换IP。
import requests
url = 'https://example.com'
proxies = {'http': 'http://your_proxy_ip:your_proxy_port'}
response = requests.get(url, proxies=proxies)
print(response.text)
2、模拟浏览器行为
通过设置请求头,可以模拟浏览器行为,避免被识别为爬虫。
import requests
url = 'https://example.com'
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
print(response.text)
3、处理验证码
对于需要验证码的网页,可以使用OCR技术识别验证码,或通过第三方打码平台进行识别。
七、存储爬取的数据
爬取到的数据需要进行存储,以便后续分析和处理。可以选择以下几种方式进行存储:
1、存储到文件
可以将爬取到的数据存储到本地文件,如CSV、JSON、TXT等。
data = {'Title': 'Example', 'Heading': 'Hello, world!'}
with open('data.json', 'w') as file:
json.dump(data, file)
2、存储到数据库
可以将爬取到的数据存储到数据库,如MySQL、MongoDB等。
import pymysql
connection = pymysql.connect(host='localhost', user='user', password='password', database='database')
cursor = connection.cursor()
data = {'Title': 'Example', 'Heading': 'Hello, world!'}
sql = "INSERT INTO table_name (title, heading) VALUES (%s, %s)"
cursor.execute(sql, (data['Title'], data['Heading']))
connection.commit()
connection.close()
八、总结
使用Python爬取网页数据是一项复杂但有趣的任务。通过选择合适的工具、发送HTTP请求、解析网页内容、提取所需数据,可以高效地获取网页数据。同时,需要注意处理反爬虫机制,确保爬虫的稳定性和数据的完整性。希望这篇文章能够帮助你更好地理解和应用Python进行网页数据爬取。
相关问答FAQs:
如何选择合适的Python库进行网页数据爬取?
在Python中,有多个库可以用来爬取网页数据,最常用的包括Requests和BeautifulSoup。Requests库用于发送网络请求,获取网页内容,而BeautifulSoup则用于解析HTML和XML文档,提取所需的数据。此外,Scrapy是一个功能强大的框架,适合处理复杂的爬虫项目。选择合适的库取决于你的需求,比如数据量、页面结构的复杂性等。
在进行网页爬取时,如何处理反爬机制?
许多网站会采取反爬机制来防止自动化脚本访问。常见的措施包括IP封禁、请求频率限制等。为了绕过这些限制,可以采取一些策略,例如使用代理IP、设置请求头以模拟浏览器行为、随机化请求间隔时间等。此外,利用浏览器自动化工具如Selenium可以帮助处理需要JavaScript加载的页面。
爬取的数据如何进行存储和处理?
在获取网页数据后,存储和处理是非常重要的一步。你可以将数据存储在CSV文件、数据库(如SQLite、MySQL)或NoSQL数据库(如MongoDB)中,具体选择取决于数据的结构和后续分析需求。使用Pandas库可以方便地对爬取的数据进行清洗、分析和可视化,从而更有效地提取有价值的信息。
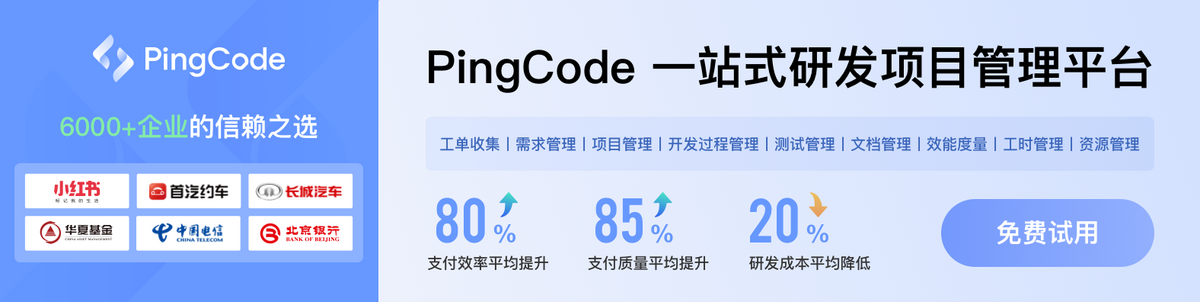