Python3如何把程序打包为exe?可以使用PyInstaller、cx_Freeze、py2exe、PyOxidizer等工具,PyInstaller是最常用的。它能够将Python程序及其所有依赖项打包成一个独立的可执行文件,方便分发和部署。下面将详细介绍如何使用PyInstaller将Python程序打包为exe文件。
一、安装PyInstaller
首先,我们需要安装PyInstaller。你可以使用pip来安装它。在命令行中运行以下命令:
pip install pyinstaller
这将下载并安装PyInstaller及其所有依赖项。
二、基础使用
1、创建示例Python程序
在开始打包之前,我们需要一个Python程序作为示例。创建一个名为example.py
的文件,并添加以下内容:
print("Hello, World!")
2、使用PyInstaller打包
在命令行中导航到包含example.py
的目录,然后运行以下命令:
pyinstaller example.py
PyInstaller将创建几个文件和文件夹,包括一个dist
文件夹,里面包含你的可执行文件,以及一个build
文件夹,包含中间构建文件,还有一个example.spec
文件,包含打包配置。
在dist
文件夹中,你会找到一个名为example
的文件夹,里面包含你的可执行文件example.exe
。
三、详细配置
1、使用.spec文件
PyInstaller生成的.spec
文件包含了打包配置。你可以编辑这个文件来定制你的打包过程。例如,你可以添加图标、数据文件、改变打包模式等。
打开example.spec
文件,你会看到类似以下内容:
# -*- mode: python ; coding: utf-8 -*-
block_cipher = None
a = Analysis(
['example.py'],
pathex=[],
binaries=[],
datas=[],
hiddenimports=[],
hookspath=[],
runtime_hooks=[],
excludes=[],
win_no_prefer_redirects=False,
win_private_assemblies=False,
cipher=block_cipher,
)
pyz = PYZ(a.pure, a.zipped_data,
cipher=block_cipher,
)
exe = EXE(
pyz,
a.scripts,
[],
exclude_binaries=True,
name='example',
debug=False,
bootloader_ignore_signals=False,
strip=False,
upx=True,
console=True,
disable_windowed_traceback=False,
target_arch=None,
codesign_identity=None,
entitlements_file=None,
)
coll = COLLECT(
exe,
a.binaries,
a.zipfiles,
a.datas,
strip=False,
upx=True,
upx_exclude=[],
name='example',
)
2、添加图标
如果你想为你的exe文件添加一个图标,你可以在.spec文件的EXE部分中添加icon
参数,例如:
exe = EXE(
pyz,
a.scripts,
[],
exclude_binaries=True,
name='example',
debug=False,
bootloader_ignore_signals=False,
strip=False,
upx=True,
console=True,
disable_windowed_traceback=False,
target_arch=None,
codesign_identity=None,
entitlements_file=None,
icon='path/to/icon.ico', # 添加图标路径
)
3、打包数据文件
如果你的程序需要一些外部数据文件,你可以在.spec文件的Analysis部分添加datas
参数,例如:
a = Analysis(
['example.py'],
pathex=[],
binaries=[],
datas=[('path/to/datafile', 'destination_directory')],
hiddenimports=[],
hookspath=[],
runtime_hooks=[],
excludes=[],
win_no_prefer_redirects=False,
win_private_assemblies=False,
cipher=block_cipher,
)
四、常见问题及解决方法
1、打包后的程序无法运行
如果打包后的程序无法运行,可能是因为缺少某些依赖项。你可以尝试在.spec文件的Analysis部分添加hiddenimports
参数,显式指定这些依赖项。例如:
a = Analysis(
['example.py'],
pathex=[],
binaries=[],
datas=[],
hiddenimports=['some_module', 'another_module'],
hookspath=[],
runtime_hooks=[],
excludes=[],
win_no_prefer_redirects=False,
win_private_assemblies=False,
cipher=block_cipher,
)
2、减少可执行文件的大小
打包后的可执行文件可能会非常大,你可以使用UPX(Ultimate Packer for eXecutables)来压缩它。确保UPX已安装,并在.spec文件的EXE部分设置upx=True
,例如:
exe = EXE(
pyz,
a.scripts,
[],
exclude_binaries=True,
name='example',
debug=False,
bootloader_ignore_signals=False,
strip=False,
upx=True, # 启用UPX压缩
console=True,
disable_windowed_traceback=False,
target_arch=None,
codesign_identity=None,
entitlements_file=None,
)
3、在打包过程中忽略某些文件
如果你想在打包过程中忽略某些文件,可以在.spec文件的Analysis部分添加excludes
参数,例如:
a = Analysis(
['example.py'],
pathex=[],
binaries=[],
datas=[],
hiddenimports=[],
hookspath=[],
runtime_hooks=[],
excludes=['unwanted_module', 'another_unwanted_module'],
win_no_prefer_redirects=False,
win_private_assemblies=False,
cipher=block_cipher,
)
五、总结
将Python程序打包为exe文件是分发和部署Python应用程序的重要步骤。PyInstaller是一个强大且灵活的工具,它能够处理大多数打包需求。通过安装PyInstaller、创建示例程序、使用基本命令打包程序、以及详细配置.spec文件,你可以轻松地将Python程序打包为exe文件。希望这篇文章能帮助你更好地理解并使用PyInstaller。如果你遇到任何问题,可以参考PyInstaller的官方文档或社区资源以获得更多帮助。
相关问答FAQs:
如何使用Python将我的程序打包成exe文件?
要将Python程序打包为exe文件,可以使用像PyInstaller、cx_Freeze或py2exe等工具。PyInstaller是最常用的选择,它可以将Python脚本及其依赖项打包成一个独立的可执行文件。使用方法通常包括在命令行中运行pyinstaller your_script.py
,生成的exe文件会在dist文件夹中找到。
打包后的exe文件能在没有Python环境的计算机上运行吗?
是的,使用PyInstaller等工具打包后的exe文件是独立的,可以在没有安装Python的计算机上运行。打包过程中,工具会将Python解释器和所有依赖的库打包到exe文件中,确保程序可以在目标计算机上正常执行。
打包exe文件后如何处理程序图标和其他资源文件?
在使用PyInstaller打包时,可以通过添加参数来指定程序的图标和其他资源文件。例如,使用--icon=icon.ico
参数可以设置程序的图标。如果你的程序还需要其他文件(如配置文件、数据文件等),可以在.spec文件中进行相应的配置,确保这些资源文件被正确包含在exe文件中。
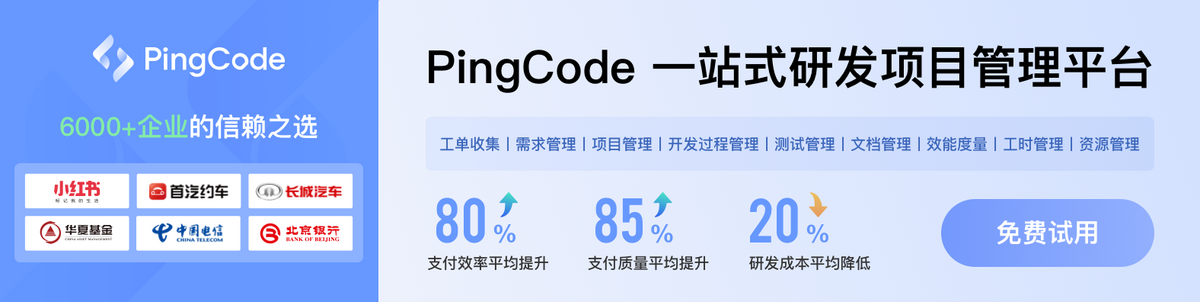