生成随机密码的几种方法包括:使用内置库、结合字母和特殊字符、调整密码长度和复杂度。其中,使用Python内置库是最简单和安全的方法,因为这些库已经经过优化和测试,可以确保生成的密码具有高随机性和安全性。
使用Python的内置库,如random
和secrets
,可以轻松生成随机密码。secrets
库是专为生成密码或其他安全令牌而设计的,它比random
库更安全。下面将详细介绍如何使用这些库生成随机密码。
一、使用random库生成随机密码
Python的random
库提供了多种方法来生成随机数和选择随机元素。下面是一个简单的例子,使用random
库生成一个包含字母、数字和特殊字符的随机密码:
import random
import string
def generate_password(length=12):
characters = string.ascii_letters + string.digits + string.punctuation
password = ''.join(random.choice(characters) for i in range(length))
return password
password = generate_password()
print(password)
这个函数generate_password
接受一个参数length
,表示密码的长度,默认值为12。string
模块提供了所有字母、数字和特殊字符的集合,通过random.choice
随机选择这些字符,生成一个随机密码。
二、使用secrets库生成随机密码
secrets
库在生成密码方面比random
库更安全。它专门设计用于生成密码、认证令牌和其他需要高随机性的数据。下面是一个使用secrets
库生成随机密码的例子:
import secrets
import string
def generate_secure_password(length=12):
characters = string.ascii_letters + string.digits + string.punctuation
password = ''.join(secrets.choice(characters) for i in range(length))
return password
secure_password = generate_secure_password()
print(secure_password)
这个函数与前面的例子类似,但使用secrets.choice
来选择字符,确保密码的随机性和安全性。
三、调整密码长度和复杂度
根据不同的需求,可以调整密码的长度和复杂度。对于某些应用,可能需要更长、更复杂的密码。以下是一个例子,生成一个长度为16的复杂密码:
import secrets
import string
def generate_complex_password(length=16):
characters = string.ascii_letters + string.digits + string.punctuation
password = ''.join(secrets.choice(characters) for i in range(length))
return password
complex_password = generate_complex_password()
print(complex_password)
这个函数generate_complex_password
生成一个包含字母、数字和特殊字符的16位长的复杂密码。
四、确保密码的多样性
为了提高密码的安全性,可以确保生成的密码包含至少一个大写字母、一个小写字母、一个数字和一个特殊字符。下面是一个改进的例子,确保密码的多样性:
import secrets
import string
def generate_diverse_password(length=12):
if length < 4:
raise ValueError("Password length must be at least 4")
characters = string.ascii_letters + string.digits + string.punctuation
password = [
secrets.choice(string.ascii_uppercase),
secrets.choice(string.ascii_lowercase),
secrets.choice(string.digits),
secrets.choice(string.punctuation)
]
password += [secrets.choice(characters) for i in range(length - 4)]
secrets.SystemRandom().shuffle(password)
return ''.join(password)
diverse_password = generate_diverse_password()
print(diverse_password)
在这个函数中,首先确保密码包含至少一个大写字母、一个小写字母、一个数字和一个特殊字符。然后,生成其余的字符,并将所有字符打乱以确保随机性。
五、使用外部库生成随机密码
除了Python内置库,还有许多外部库可以用来生成随机密码,例如passlib
和cryptography
。这些库提供了更多的功能和更高的安全性。以下是使用passlib
库生成随机密码的例子:
from passlib.context import CryptContext
pwd_context = CryptContext(schemes=["pbkdf2_sha256"], deprecated="auto")
def generate_passlib_password(length=12):
password = pwd_context.hash(secrets.token_urlsafe(length))
return password
passlib_password = generate_passlib_password()
print(passlib_password)
这个例子使用passlib
库生成一个随机密码,并使用pbkdf2_sha256
算法对其进行哈希处理。
六、生成符合特定规则的密码
在某些情况下,可能需要生成符合特定规则的密码。例如,某些系统要求密码必须包含特定数量的大写字母、小写字母、数字和特殊字符。下面是一个例子,生成一个符合这些特定规则的密码:
import secrets
import string
def generate_custom_password(length=12, upper=2, lower=2, digits=2, special=2):
if length < upper + lower + digits + special:
raise ValueError("Password length is too short for the given requirements")
password = [
secrets.choice(string.ascii_uppercase) for _ in range(upper)
] + [
secrets.choice(string.ascii_lowercase) for _ in range(lower)
] + [
secrets.choice(string.digits) for _ in range(digits)
] + [
secrets.choice(string.punctuation) for _ in range(special)
]
remaining_length = length - len(password)
all_characters = string.ascii_letters + string.digits + string.punctuation
password += [secrets.choice(all_characters) for _ in range(remaining_length)]
secrets.SystemRandom().shuffle(password)
return ''.join(password)
custom_password = generate_custom_password(length=16, upper=4, lower=4, digits=4, special=4)
print(custom_password)
在这个函数中,参数upper
、lower
、digits
和special
分别指定大写字母、小写字母、数字和特殊字符的数量。首先生成这些特定数量的字符,然后生成剩余的字符,并将所有字符打乱以确保随机性。
七、生成基于用户输入的密码
有时候,用户可能希望生成一个基于特定输入的随机密码,例如基于某个关键词。下面是一个例子,生成一个基于用户输入的随机密码:
import secrets
import string
def generate_password_from_input(input_string, length=12):
if not input_string:
raise ValueError("Input string cannot be empty")
characters = string.ascii_letters + string.digits + string.punctuation
random_part = ''.join(secrets.choice(characters) for _ in range(length - len(input_string)))
password = list(input_string + random_part)
secrets.SystemRandom().shuffle(password)
return ''.join(password)
input_string = "UserInput"
password_from_input = generate_password_from_input(input_string, length=16)
print(password_from_input)
在这个函数中,input_string
是用户提供的输入字符串。函数首先确保输入字符串不为空,然后生成剩余的随机字符,并将所有字符打乱以确保随机性。
八、生成符合特定字符集的密码
有时候,可能需要生成仅包含特定字符集的密码。例如,某些系统可能不允许使用特殊字符。下面是一个例子,生成一个仅包含字母和数字的随机密码:
import secrets
import string
def generate_alpha_numeric_password(length=12):
characters = string.ascii_letters + string.digits
password = ''.join(secrets.choice(characters) for i in range(length))
return password
alpha_numeric_password = generate_alpha_numeric_password()
print(alpha_numeric_password)
在这个函数中,characters
仅包含字母和数字,通过secrets.choice
随机选择这些字符,生成一个随机密码。
九、生成符合特定安全标准的密码
某些行业和应用有特定的安全标准,例如PCI DSS(支付卡行业数据安全标准)。下面是一个例子,生成一个符合PCI DSS标准的随机密码:
import secrets
import string
def generate_pci_dss_password(length=12):
if length < 7:
raise ValueError("Password length must be at least 7 for PCI DSS compliance")
characters = string.ascii_letters + string.digits + string.punctuation
password = ''.join(secrets.choice(characters) for i in range(length))
# Ensure the password meets PCI DSS complexity requirements
if (not any(c.islower() for c in password) or
not any(c.isupper() for c in password) or
not any(c.isdigit() for c in password) or
not any(c in string.punctuation for c in password)):
return generate_pci_dss_password(length)
return password
pci_dss_password = generate_pci_dss_password()
print(pci_dss_password)
在这个函数中,首先确保密码的长度至少为7,然后生成一个随机密码。接着,检查密码是否符合PCI DSS的复杂性要求,如果不符合,则重新生成密码。
十、生成基于时间戳的随机密码
在某些情况下,可能需要生成基于时间戳的随机密码,以确保每次生成的密码都唯一。下面是一个例子,生成一个基于时间戳的随机密码:
import secrets
import string
import time
def generate_timestamp_password(length=12):
timestamp = str(int(time.time()))
characters = string.ascii_letters + string.digits + string.punctuation
random_part = ''.join(secrets.choice(characters) for i in range(length - len(timestamp)))
password = list(timestamp + random_part)
secrets.SystemRandom().shuffle(password)
return ''.join(password)
timestamp_password = generate_timestamp_password()
print(timestamp_password)
在这个函数中,首先获取当前的时间戳,并将其转换为字符串。然后生成剩余的随机字符,并将所有字符打乱以确保随机性。
结论
生成随机密码是确保数据安全的重要一步。使用Python的内置库random
和secrets
,可以轻松生成高随机性和高安全性的密码。还可以根据特定需求调整密码的长度和复杂度,确保密码符合特定规则或安全标准。无论是生成简单的随机密码,还是符合复杂规则的密码,Python提供了丰富的工具和库来满足各种需求。
相关问答FAQs:
如何在Python3中生成安全的随机密码?
在Python3中,可以使用secrets
模块生成安全的随机密码。该模块专为生成密码和密钥而设计,提供比random
模块更高的安全性。可以使用以下代码示例生成随机密码:
import secrets
import string
def generate_password(length=12):
alphabet = string.ascii_letters + string.digits + string.punctuation
password = ''.join(secrets.choice(alphabet) for _ in range(length))
return password
print(generate_password(12))
这个函数将生成一个包含字母、数字和符号的随机密码,长度可以根据需要调整。
生成随机密码时有哪些安全性考虑?
在生成随机密码时,确保密码的长度和复杂性至关重要。建议密码长度至少为12个字符,并包含大写字母、小写字母、数字和特殊字符。此外,避免使用常见单词或模式,以降低被破解的风险。使用secrets
模块可以确保密码的随机性。
可以自定义密码生成的字符集吗?
是的,可以根据需求自定义密码生成的字符集。例如,如果希望生成一个仅包含字母和数字的密码,可以修改代码中的alphabet
变量,只包含string.ascii_letters
和string.digits
。如下所示:
alphabet = string.ascii_letters + string.digits
这样生成的密码会更简洁,但安全性可能会有所降低。
如何确保生成的密码不会重复?
如果需要确保生成的密码唯一,可以将已生成的密码存储在列表或集合中,每次生成新密码时,检查该密码是否已经存在于存储中。如果存在,则重新生成,直到获得一个唯一的密码。例如:
passwords = set() # 用于存储已生成的密码
def unique_password(length=12):
while True:
new_password = generate_password(length)
if new_password not in passwords:
passwords.add(new_password)
return new_password
这种方法可以有效避免重复密码的生成。
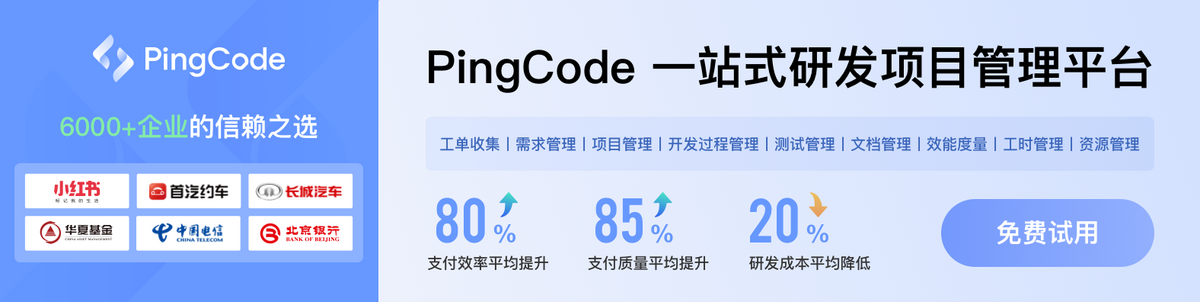