要在Python中替换字符串中的值,可以使用replace()方法、re模块、translate()方法。replace()方法是最常用的方法,因为它简单且易于使用。re模块则适用于更复杂的模式匹配和替换。translate()方法适用于替换多个字符。
replace()方法是最常用的,它可以轻松地替换字符串中的子字符串。
# 示例代码
text = "Hello World"
new_text = text.replace("World", "Python")
print(new_text) # 输出:Hello Python
一、replace()方法
replace()方法是Python中替换字符串值最直接的方法。它用于替换字符串中的指定子字符串。
text = "Hello World! Welcome to the World of Python."
new_text = text.replace("World", "Universe")
print(new_text) # 输出:Hello Universe! Welcome to the Universe of Python.
该方法有两个参数,第一个参数是要替换的子字符串,第二个参数是新的子字符串。replace()方法返回一个新的字符串,并不会更改原始字符串。
使用replace()替换多个子字符串
replace()方法不仅可以替换单个子字符串,还可以通过链式调用替换多个子字符串。
text = "Hello World! Welcome to the World of Python."
new_text = text.replace("World", "Universe").replace("Python", "Programming")
print(new_text) # 输出:Hello Universe! Welcome to the Universe of Programming.
二、re模块
re模块提供了一个更强大的方法来替换字符串中的值,特别是当你需要基于模式进行替换时。re模块中的sub()方法可以用于此目的。
import re
text = "Hello World! Welcome to the World of Python."
new_text = re.sub(r"World", "Universe", text)
print(new_text) # 输出:Hello Universe! Welcome to the Universe of Python.
使用re模块进行复杂替换
re模块非常适合进行复杂的替换操作。例如,你可以使用正则表达式来匹配模式,并替换匹配的部分。
import re
text = "The price is $10.50"
new_text = re.sub(r"\$\d+\.\d+", "$20.00", text)
print(new_text) # 输出:The price is $20.00
在这个示例中,$\d+.\d+ 是一个正则表达式模式,用于匹配价格格式,然后将其替换为新的价格。
三、translate()方法
translate()方法适用于替换字符串中的多个字符。你需要先创建一个翻译表,然后使用translate()方法进行替换。
text = "Hello World"
translation_table = str.maketrans("Helo", "Jplm")
new_text = text.translate(translation_table)
print(new_text) # 输出:Jpllm Wprld
使用translate()方法替换多个字符
text = "abcdef"
translation_table = str.maketrans("abc", "123")
new_text = text.translate(translation_table)
print(new_text) # 输出:123def
在这个示例中,str.maketrans()函数创建了一个翻译表,将字符"a"、"b"、"c"分别替换为"1"、"2"、"3"。
四、使用字符串格式化方法
有时你可能需要在字符串中插入或替换值,这时可以使用字符串格式化方法。
name = "John"
age = 30
text = f"My name is {name} and I am {age} years old."
print(text) # 输出:My name is John and I am 30 years old.
使用.format()方法进行字符串替换
name = "John"
age = 30
text = "My name is {} and I am {} years old.".format(name, age)
print(text) # 输出:My name is John and I am 30 years old.
五、正则表达式的高级用法
正则表达式提供了更多强大的功能,使你能够在字符串中进行复杂的替换操作。例如,使用re模块的sub()方法和lambda函数,你可以根据匹配模式进行动态替换。
import re
text = "The price is $10.50"
new_text = re.sub(r"\$\d+\.\d+", lambda x: f"${float(x.group()[1:]) * 2:.2f}", text)
print(new_text) # 输出:The price is $21.00
在这个示例中,lambda函数根据匹配的模式动态计算新的价格。
六、字符串分割和连接
有时候你可能需要先分割字符串,然后再进行替换操作。可以使用split()方法和join()方法来实现。
text = "Hello World! Welcome to the World of Python."
parts = text.split("World")
new_text = "Universe".join(parts)
print(new_text) # 输出:Hello Universe! Welcome to the Universe of Python.
使用split()和join()进行复杂替换
text = "apple, banana, cherry"
parts = text.split(", ")
new_parts = [part.upper() for part in parts]
new_text = ", ".join(new_parts)
print(new_text) # 输出:APPLE, BANANA, CHERRY
在这个示例中,我们首先分割字符串,然后将每个部分转换为大写,最后重新连接起来。
七、字符串替换的性能考虑
在处理大文本或需要进行大量替换操作时,性能可能成为一个问题。可以考虑使用更高效的方法或优化你的代码。
使用正则表达式进行批量替换
import re
text = "apple, banana, cherry"
patterns = {"apple": "APPLE", "banana": "BANANA", "cherry": "CHERRY"}
pattern = re.compile("|".join(patterns.keys()))
new_text = pattern.sub(lambda x: patterns[x.group()], text)
print(new_text) # 输出:APPLE, BANANA, CHERRY
在这个示例中,我们使用正则表达式进行批量替换,从而提高了性能。
八、实际应用中的字符串替换
字符串替换在实际应用中非常常见。例如,在处理用户输入、生成动态内容、数据清理和转换等方面,都需要进行字符串替换。
处理用户输入
user_input = "Hello, my name is John. I am 30 years old."
cleaned_input = user_input.replace("John", "Jane").replace("30", "25")
print(cleaned_input) # 输出:Hello, my name is Jane. I am 25 years old.
生成动态内容
name = "John"
template = "Hello, {name}! Welcome to our website."
message = template.format(name=name)
print(message) # 输出:Hello, John! Welcome to our website.
在这个示例中,我们使用字符串格式化方法生成动态内容。
数据清理和转换
data = "The price is $10.50"
cleaned_data = re.sub(r"\$\d+\.\d+", "PRICE", data)
print(cleaned_data) # 输出:The price is PRICE
通过使用正则表达式,我们可以轻松地清理和转换数据。
九、总结
在Python中替换字符串中的值有多种方法,包括replace()方法、re模块、translate()方法和字符串格式化方法。每种方法都有其独特的优势和适用场景。replace()方法适用于简单的替换操作,re模块适用于复杂的模式匹配和替换,translate()方法适用于替换多个字符,而字符串格式化方法适用于生成动态内容。了解并掌握这些方法,可以帮助你在不同的应用场景中高效地进行字符串替换操作。
相关问答FAQs:
如何使用Python中的replace()函数替换字符串中的特定值?
在Python中,replace()函数是一个非常方便的方法,可以用于替换字符串中的特定子串。它的基本语法是str.replace(old, new, count)
,其中old
是需要被替换的子串,new
是替换后的新子串,count
是可选参数,表示替换的次数。例如,text.replace("apple", "orange", 1)
将只替换第一个“apple”为“orange”。
有没有其他方法可以在Python中替换字符串的值?
除了使用replace()函数,Python还提供了其他几种方法来替换字符串的值。例如,可以使用正则表达式库re
中的re.sub()
函数来进行更复杂的替换操作。这个方法允许使用模式匹配来找到并替换字符串中的子串,适合处理更复杂的文本替换需求。
在Python中,如何进行批量替换字符串的值?
如果需要在字符串中进行批量替换,可以考虑使用字典结合replace()方法。通过循环遍历字典中的键值对,可以实现多个值的替换。例如,使用一个字典定义要替换的子串及其对应的新值,然后使用for循环逐个调用replace()进行替换。这种方式可以简化代码,提高效率。
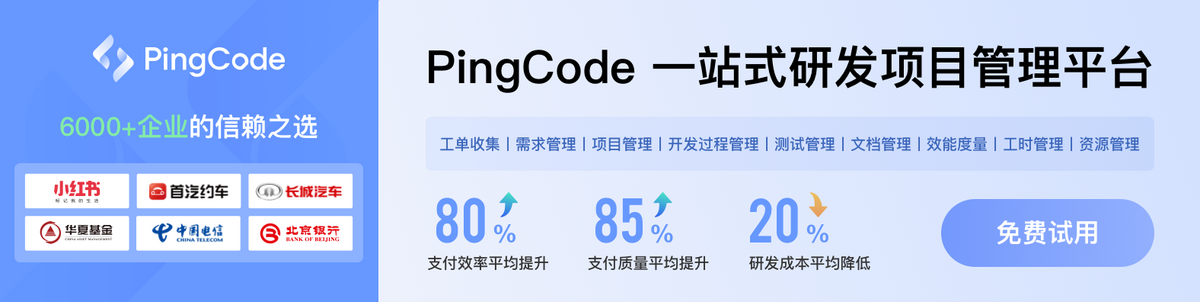