使用Python在手机上发送微信消息,需要使用微信的API或第三方库来实现。实现这个功能的步骤包括:安装第三方库、登录微信、发送消息、处理异常。接下来,我们将详细展开其中的一个重点步骤:使用微信的API或第三方库来实现微信消息发送,这个过程涉及到使用相关的Python库,如itchat。
一、安装第三方库itchat
itchat是一个开源的Python库,它可以帮助我们通过Python与微信进行交互。首先,我们需要安装这个库:
pip install itchat
安装完成后,我们需要进行一些基础的配置,以便在Python脚本中使用它。
二、登录微信
在使用itchat之前,我们需要通过扫描二维码登录微信。以下是一个示例代码:
import itchat
登录微信
itchat.auto_login(hotReload=True)
在运行这段代码时,会弹出一个二维码,用微信扫描这个二维码进行登录。hotReload=True
参数的作用是在一定时间内保持登录状态,不需要每次运行脚本都重新扫描二维码。
三、发送微信消息
登录成功后,我们可以使用以下代码发送微信消息:
# 发送文本消息
itchat.send('Hello, this is a message from Python!', toUserName='filehelper')
发送图片消息
itchat.send('@img@path/to/image.jpg', toUserName='filehelper')
在上面的代码中,toUserName='filehelper'
表示消息将发送到文件传输助手。如果你想发送消息到特定的联系人或微信群,需要获取他们的UserName。
四、获取联系人列表
我们可以使用以下代码获取联系人列表,并找到需要发送消息的用户:
# 获取所有联系人
friends = itchat.get_friends(update=True)
打印联系人列表
for friend in friends:
print(friend['NickName'], friend['UserName'])
通过这段代码,我们可以获得所有联系人和他们的UserName。接下来,我们可以使用这些UserName发送消息。
五、发送群消息
发送群消息的步骤类似于发送个人消息,首先我们需要获取群的UserName:
# 获取所有群聊
chatrooms = itchat.get_chatrooms(update=True)
打印群聊列表
for chatroom in chatrooms:
print(chatroom['NickName'], chatroom['UserName'])
获得群聊的UserName后,我们可以发送消息:
# 发送群消息
itchat.send('Hello, this is a message to the group!', toUserName='群聊的UserName')
六、处理异常
在实际操作中,可能会遇到一些异常情况,如网络问题、登录失效等。我们需要添加异常处理机制,以确保程序的稳定性:
try:
itchat.auto_login(hotReload=True)
itchat.send('Hello, this is a message from Python!', toUserName='filehelper')
except Exception as e:
print(f'Error: {e}')
通过以上步骤,我们就可以使用Python在手机上发送微信消息了。下面是一个完整的示例代码:
import itchat
try:
# 登录微信
itchat.auto_login(hotReload=True)
# 获取联系人列表
friends = itchat.get_friends(update=True)
for friend in friends:
print(friend['NickName'], friend['UserName'])
# 发送消息到文件传输助手
itchat.send('Hello, this is a message from Python!', toUserName='filehelper')
# 获取群聊列表
chatrooms = itchat.get_chatrooms(update=True)
for chatroom in chatrooms:
print(chatroom['NickName'], chatroom['UserName'])
# 发送群消息
itchat.send('Hello, this is a message to the group!', toUserName='群聊的UserName')
except Exception as e:
print(f'Error: {e}')
七、自动回复消息
除了发送消息外,我们还可以使用itchat实现自动回复功能:
import itchat
@itchat.msg_register(itchat.content.TEXT)
def text_reply(msg):
return f'I received: {msg["Text"]}'
itchat.auto_login(hotReload=True)
itchat.run()
在这段代码中,当收到文本消息时,程序会自动回复收到的消息。
八、发送文件和图片
除了文本消息,我们还可以发送文件和图片:
# 发送文件
itchat.send_file('path/to/file.txt', toUserName='filehelper')
发送图片
itchat.send_image('path/to/image.jpg', toUserName='filehelper')
九、扩展功能
itchat库还提供了许多其他功能,如获取聊天记录、管理群聊等。我们可以根据需要进行扩展和定制。
十、总结
通过以上步骤,我们可以使用Python在手机上发送微信消息。关键步骤包括:安装itchat库、登录微信、获取联系人和群聊列表、发送消息、处理异常和扩展功能。希望这篇文章能帮助你更好地理解和使用Python进行微信消息发送。如果你有任何问题或建议,欢迎留言讨论。
相关问答FAQs:
如何在手机上使用Python发送微信消息?
要在手机上使用Python发送微信消息,您可以借助一些第三方库,例如itchat
。首先,您需要在手机上安装Python环境,然后通过命令行或Python IDE编写脚本,利用itchat
库实现发送消息的功能。请确保您已登录微信,并获得相应的权限。
在手机上运行Python脚本需要什么环境?
在手机上运行Python脚本,您需要安装Python的移动版本,如QPython或Pydroid 3等。这些应用程序能够提供Python解释器和必要的库支持,让您能够在移动设备上顺利运行Python代码。
如何确保在发送微信消息时不被封号?
为了避免在使用Python发送微信消息时遭到封号,建议您遵循一些基本原则:控制消息发送频率,避免批量发送相同内容,保持与朋友的正常互动,以及遵守微信的使用规范。此外,定期更新您的库和工具,以确保它们符合最新的安全标准。
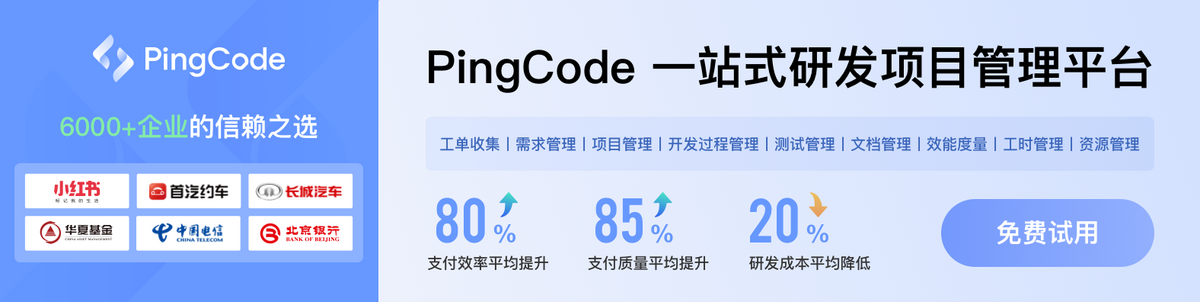