在Python字符串中,匹配数字可以通过使用正则表达式(Regular Expressions)来实现。正则表达式、re模块、\d元字符是实现这一功能的核心工具。正则表达式是一种强大的字符串处理工具,通过其丰富的语法和功能,可以方便地匹配、搜索、替换字符串中的特定模式。Python中提供了re
模块来处理正则表达式。
正则表达式的核心概念:
正则表达式(Regular Expression,简称RegEx)是一种模式匹配的语法规则,通过定义特定的模式,可以匹配字符串中的符合条件的子字符串。在Python中,re
模块提供了丰富的正则表达式操作函数,如re.search()
, re.match()
, re.findall()
, re.sub()
等。
一、使用re模块匹配数字
Python中的re
模块允许我们使用正则表达式来匹配字符串中的数字。以下是一些基本的方法:
1、引入re模块
在使用正则表达式之前,首先需要引入Python的re
模块:
import re
2、使用re.search()函数
re.search(pattern, string)
函数用于在字符串中搜索模式,并返回第一个匹配的对象。如果没有找到匹配项,则返回None
。
import re
text = "The price is 100 dollars"
pattern = r'\d+'
match = re.search(pattern, text)
if match:
print(f"Found a number: {match.group()}")
else:
print("No number found")
在这个例子中,r'\d+'
是正则表达式模式,其中\d
表示任何数字字符,+
表示匹配一个或多个数字字符。match.group()
返回匹配的字符串。
3、使用re.findall()函数
re.findall(pattern, string)
函数返回字符串中所有与模式匹配的子字符串的列表。
import re
text = "There are 3 cats, 4 dogs, and 5 birds"
pattern = r'\d+'
numbers = re.findall(pattern, text)
print(f"Found numbers: {numbers}")
在这个例子中,re.findall()
函数返回所有匹配的数字,并以列表的形式输出。
4、使用re.match()函数
re.match(pattern, string)
函数从字符串的开始位置匹配模式,如果在开始位置没有匹配项,则返回None
。
import re
text = "1234 is a number"
pattern = r'\d+'
match = re.match(pattern, text)
if match:
print(f"Found a number at the beginning: {match.group()}")
else:
print("No number found at the beginning")
在这个例子中,re.match()
函数仅在字符串的开头匹配数字。
5、使用re.sub()函数
re.sub(pattern, repl, string)
函数用于替换字符串中所有与模式匹配的子字符串。
import re
text = "There are 3 cats, 4 dogs, and 5 birds"
pattern = r'\d+'
result = re.sub(pattern, "X", text)
print(f"Replaced text: {result}")
在这个例子中,re.sub()
函数将所有匹配的数字替换为"X"
。
二、详细解读正则表达式中的数字匹配
1、\d元字符
\d
是正则表达式中的一个元字符,表示匹配任何数字字符(0-9)。可以单独使用,也可以与其他字符组合使用。
例如:
r'\d'
:匹配单个数字字符。r'\d+'
:匹配一个或多个连续的数字字符。r'\d{2}'
:匹配恰好两个数字字符。
2、使用字符类
字符类是一种包含在方括号[]
中的字符集合,用于匹配字符类中的任何一个字符。
例如:
[0-9]
:匹配任何单个数字字符。[0-9]+
:匹配一个或多个连续的数字字符。
import re
text = "My phone number is 123-456-7890"
pattern = r'[0-9]+'
numbers = re.findall(pattern, text)
print(f"Found numbers: {numbers}")
3、匹配带小数点的数字
要匹配带小数点的数字,可以使用点字符.
,但需要使用转义字符来表示点字符本身。
例如:
r'\d+\.\d+'
:匹配整数部分和小数部分的数字。
import re
text = "The price is 99.99 dollars"
pattern = r'\d+\.\d+'
match = re.search(pattern, text)
if match:
print(f"Found a decimal number: {match.group()}")
else:
print("No decimal number found")
4、匹配负数
要匹配负数,可以在模式中添加一个可选的负号-
,并使用问号?
表示零个或一个负号。
例如:
r'-?\d+'
:匹配整数,包括可选的负号。r'-?\d+\.\d+'
:匹配带小数点的数字,包括可选的负号。
import re
text = "The temperature is -5.5 degrees"
pattern = r'-?\d+\.\d+'
match = re.search(pattern, text)
if match:
print(f"Found a negative decimal number: {match.group()}")
else:
print("No negative decimal number found")
三、总结
通过使用Python的re
模块和正则表达式,我们可以轻松地在字符串中匹配数字。正则表达式、re模块、\d元字符是实现这一功能的核心工具。通过掌握这些技术,我们可以高效地处理字符串,提取和操作数字内容。无论是简单的整数匹配,还是复杂的小数和负数匹配,正则表达式都能提供强大的支持,帮助我们完成各种文本处理任务。
相关问答FAQs:
在Python中,如何使用正则表达式来匹配字符串中的数字?
使用Python的re
模块可以方便地对字符串进行正则表达式匹配。可以使用re.findall()
函数来查找字符串中的所有数字。例如,re.findall(r'\d+', your_string)
将返回一个包含所有数字的列表。
如何匹配字符串中的小数或负数?
要匹配小数或负数,可以使用更复杂的正则表达式。例如,使用r'-?\d+\.?\d*'
可以匹配负数和小数,这个表达式会包括可选的负号和小数点。
在字符串中找到数字后,如何将其转换为整数或浮点数?
通过使用int()
或float()
函数,可以将匹配到的数字字符串转换为相应的数据类型。可以结合re.findall()
来获取数字字符串后进行转换,例如:
import re
numbers = re.findall(r'\d+', your_string)
integers = [int(num) for num in numbers]
这种方式可以将字符串中的所有数字提取并转换为整数列表。
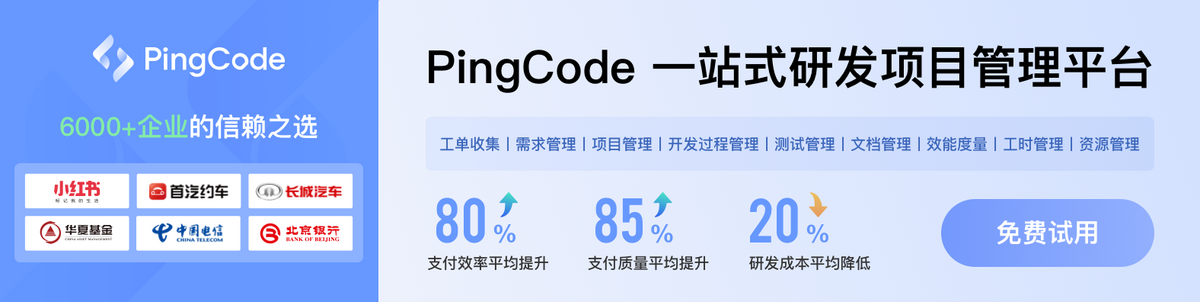