开头段落:
在Python文件里写判断语句的方法包括使用if语句、if-else语句、if-elif-else语句、三元运算符。这些语句结构能够帮助程序根据条件的不同执行不同的代码块。if语句是最基本的判断语句,只需要一个条件表达式,如果该条件为真,则执行相应的代码块。如果需要在条件为假时执行其他代码块,可以使用if-else语句。当有多个条件需要判断时,可以使用if-elif-else语句。此外,对于简单的条件判断,可以使用三元运算符来简化代码。if语句是最基础的控制流语句,在Python中使用频率非常高,掌握它的用法是学习Python编程的第一步。
一、IF语句
if语句是Python中最简单的控制流语句,用于在条件为真时执行特定的代码块。其基本语法如下:
if condition:
# code to execute if condition is true
例如,我们可以编写一个简单的程序来判断一个数字是否为正数:
number = 10
if number > 0:
print("The number is positive")
在上面的代码中,if number > 0:
是条件表达式,如果number
的值大于0,则执行print("The number is positive")
。
1.1、嵌套if语句
有时我们可能需要在一个if语句内部再嵌套一个或多个if语句。这种情况常见于多个条件需要同时满足的情况下。例如:
number = 10
if number > 0:
if number % 2 == 0:
print("The number is positive and even")
在这个例子中,如果number
大于0且是偶数,那么会打印出"The number is positive and even"。
二、IF-ELSE语句
if-else语句用于在条件表达式为假时执行另一段代码。其基本语法如下:
if condition:
# code to execute if condition is true
else:
# code to execute if condition is false
例如,我们可以扩展之前的例子,添加一个else语句来处理负数的情况:
number = -5
if number > 0:
print("The number is positive")
else:
print("The number is negative")
在上面的代码中,如果number
的值大于0,则执行print("The number is positive")
,否则执行print("The number is negative")
。
2.1、嵌套if-else语句
同样,if-else语句也可以嵌套使用。例如:
number = -5
if number > 0:
if number % 2 == 0:
print("The number is positive and even")
else:
print("The number is positive and odd")
else:
print("The number is negative")
在这个例子中,如果number
为正数且是偶数,则打印"The number is positive and even",否则打印"The number is positive and odd"。如果number
为负数,则打印"The number is negative"。
三、IF-ELIF-ELSE语句
当有多个条件需要判断时,可以使用if-elif-else语句。其基本语法如下:
if condition1:
# code to execute if condition1 is true
elif condition2:
# code to execute if condition2 is true
else:
# code to execute if none of the above conditions are true
例如,我们可以编写一个程序来判断一个数字是正数、零还是负数:
number = 0
if number > 0:
print("The number is positive")
elif number == 0:
print("The number is zero")
else:
print("The number is negative")
在上面的代码中,如果number
大于0,则执行print("The number is positive")
;如果number
等于0,则执行print("The number is zero")
;否则执行print("The number is negative")
。
3.1、多个elif语句
if-elif-else语句可以包含多个elif语句,用于处理更多的条件。例如:
score = 85
if score >= 90:
print("Grade: A")
elif score >= 80:
print("Grade: B")
elif score >= 70:
print("Grade: C")
elif score >= 60:
print("Grade: D")
else:
print("Grade: F")
在上面的代码中,根据score
的值,会打印出相应的等级。
四、三元运算符
三元运算符是一种简洁的条件判断语法,用于在单行代码中实现简单的条件判断。其基本语法如下:
value_if_true if condition else value_if_false
例如,我们可以使用三元运算符来判断一个数字是正数还是负数:
number = 10
result = "Positive" if number > 0 else "Negative"
print(result)
在上面的代码中,如果number
大于0,则result
的值为"Positive",否则为"Negative"。
4.1、嵌套三元运算符
三元运算符也可以嵌套使用,但为了代码的可读性,建议尽量避免过多的嵌套。例如:
number = 0
result = "Positive" if number > 0 else ("Zero" if number == 0 else "Negative")
print(result)
在这个例子中,如果number
大于0,则result
的值为"Positive";如果number
等于0,则result
的值为"Zero";否则result
的值为"Negative"。
五、逻辑运算符
在判断语句中,逻辑运算符(and、or、not)可以用来组合多个条件。使用逻辑运算符可以使条件判断更加复杂和灵活。
5.1、AND逻辑运算符
and逻辑运算符用于在多个条件都为真时执行代码块。例如:
age = 25
income = 50000
if age > 18 and income > 30000:
print("Eligible for loan")
在上面的代码中,只有当age
大于18且income
大于30000时,才会打印"Eligible for loan"。
5.2、OR逻辑运算符
or逻辑运算符用于在任意一个条件为真时执行代码块。例如:
age = 25
income = 20000
if age > 18 or income > 30000:
print("Eligible for credit card")
在上面的代码中,只要age
大于18或income
大于30000中的任意一个条件满足,都会打印"Eligible for credit card"。
5.3、NOT逻辑运算符
not逻辑运算符用于取反条件表达式的值。例如:
is_raining = False
if not is_raining:
print("Go for a walk")
在上面的代码中,如果is_raining
为False(即不下雨),则会打印"Go for a walk"。
六、成员运算符和身份运算符
除了逻辑运算符,Python还提供了成员运算符(in、not in)和身份运算符(is、is not)用于条件判断。
6.1、成员运算符
成员运算符用于检查某个值是否存在于一个序列(如列表、元组、字符串)中。例如:
fruits = ["apple", "banana", "cherry"]
if "banana" in fruits:
print("Banana is in the list")
在上面的代码中,如果"banana"存在于fruits
列表中,则会打印"Banana is in the list"。
6.2、身份运算符
身份运算符用于比较两个对象的身份(即内存地址)。例如:
a = [1, 2, 3]
b = a
if a is b:
print("a and b refer to the same object")
在上面的代码中,如果a
和b
引用的是同一个对象,则会打印"a and b refer to the same object"。
七、综合实例
通过结合以上各种判断语句和运算符,我们可以编写更加复杂的程序。例如,编写一个简单的用户认证系统:
username = "admin"
password = "123456"
input_username = input("Enter your username: ")
input_password = input("Enter your password: ")
if input_username == username and input_password == password:
print("Login successful")
elif input_username != username:
print("Invalid username")
else:
print("Invalid password")
在这个例子中,程序首先提示用户输入用户名和密码,然后使用if-elif-else语句和逻辑运算符来判断输入的用户名和密码是否正确,并打印相应的提示信息。
通过以上内容的学习和实践,相信你已经掌握了Python文件中如何写判断语句的各种方法和技巧。希望这些知识能帮助你在编写Python程序时更加得心应手。
相关问答FAQs:
判断语句在Python文件中的基本结构是什么?
在Python文件中,判断语句通常使用if
、elif
和else
关键字来实现条件判断。基本结构如下:
if condition:
# 当条件成立时执行的代码
elif another_condition:
# 当第一个条件不成立,第二个条件成立时执行的代码
else:
# 当所有条件都不成立时执行的代码
在实际编写时,condition
和another_condition
可以是任何能够返回布尔值的表达式。
如何在Python中使用多条件判断?
在Python中,可以使用逻辑运算符如and
、or
和not
来组合多个条件进行判断。例如:
if condition1 and condition2:
# 当两个条件都成立时执行的代码
elif condition3 or condition4:
# 当至少一个条件成立时执行的代码
else:
# 所有条件不成立时执行的代码
这种方式使得判断语句更加灵活,可以处理复杂的逻辑情况。
如何调试Python判断语句中的逻辑错误?
调试判断语句时,可以使用print()
函数在条件判断前后输出相关变量的值,帮助确认条件是否如预期那样成立。此外,使用Python的内置调试工具如pdb
也可以逐步执行代码,观察程序的运行状态,找到潜在的逻辑错误。示例:
print("当前值:", variable)
if variable > 10:
print("条件成立")
else:
print("条件不成立")
这种方法可以帮助开发者更好地理解程序的执行流程。
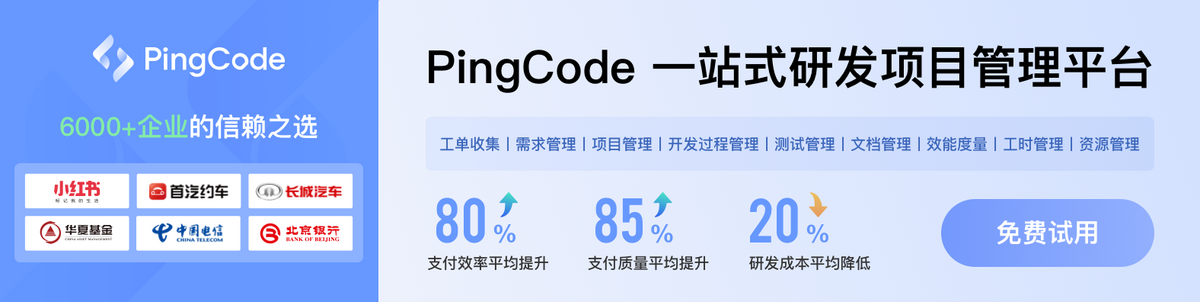