在Python中,同时判断两个条件可以使用逻辑运算符“and”或“or”,它们可以将两个条件组合在一起,逻辑运算符“and”用于判断两个条件都为真,逻辑运算符“or”用于判断其中一个条件为真。 例如:if condition1 and condition2:
和 if condition1 or condition2:
。在实际应用中,我们经常需要对复杂逻辑进行判断,这时逻辑运算符的使用会使代码更加简洁明了。
一、逻辑运算符“and”
1. 基本用法
在Python中,逻辑运算符“and”用于同时判断多个条件是否都为真。如果所有的条件都为真,则返回真,否则返回假。举个简单的例子:
a = 10
b = 20
if a < 15 and b > 15:
print("Both conditions are met.")
else:
print("One or both conditions are not met.")
在这个例子中,变量a
小于15且变量b
大于15,因此这两个条件都为真,最终输出结果为“Both conditions are met.”。
2. 多条件判断
有时候,我们需要判断多个条件是否同时满足。这时可以使用“and”将多个条件组合在一起:
x = 5
y = 10
z = 15
if x < y and y < z and x < z:
print("All conditions are met.")
else:
print("One or more conditions are not met.")
在上述代码中,所有的条件都为真,因此输出“All conditions are met.”。
二、逻辑运算符“or”
1. 基本用法
逻辑运算符“or”用于判断至少一个条件为真。如果有一个条件为真,则返回真。示例如下:
a = 10
b = 5
if a < 15 or b > 15:
print("At least one condition is met.")
else:
print("No conditions are met.")
在这个例子中,变量a
小于15,因此至少有一个条件为真,最终输出结果为“At least one condition is met.”。
2. 多条件判断
同样的,我们也可以使用“or”将多个条件组合在一起,判断是否至少有一个条件为真:
x = 5
y = 20
z = 25
if x > 10 or y > 15 or z < 30:
print("At least one condition is met.")
else:
print("No conditions are met.")
在上述代码中,变量y
大于15且变量z
小于30,因此至少有一个条件为真,最终输出“At least one condition is met.”。
三、逻辑运算符的嵌套使用
有时候,我们需要对复杂的逻辑进行判断,这时可以将“and”和“or”嵌套使用。下面是一个嵌套使用的示例:
a = 10
b = 20
c = 30
if (a < 15 and b > 15) or c == 30:
print("Complex condition is met.")
else:
print("Complex condition is not met.")
在这个例子中,前半部分的条件(a < 15 and b > 15)
为真,后半部分的条件c == 30
也为真,因此整个条件表达式为真,最终输出“Complex condition is met.”。
四、逻辑运算符的优先级
在使用“and”和“or”组合多个条件时,需要注意逻辑运算符的优先级。“and”优先级高于“or”,因此在表达式中会先计算“and”条件,再计算“or”条件:
a = 10
b = 20
c = 5
if a < 15 and b > 15 or c > 10:
print("Condition is met.")
else:
print("Condition is not met.")
在这个例子中,首先计算a < 15 and b > 15
,结果为真,然后计算整个表达式,结果仍为真,最终输出“Condition is met.”。
五、逻辑运算符的短路效应
逻辑运算符具有短路效应,即当使用“and”时,如果第一个条件为假,则不再判断后续条件;当使用“or”时,如果第一个条件为真,则不再判断后续条件。示例如下:
def func1():
print("func1")
return True
def func2():
print("func2")
return False
if func1() and func2():
print("Both functions returned True.")
else:
print("At least one function returned False.")
在这个例子中,func1
函数返回真,因此需要继续判断func2
函数的返回值,最终输出结果为:
func1
func2
At least one function returned False.
使用“or”时,如果第一个条件为真,则不再判断后续条件:
if func1() or func2():
print("At least one function returned True.")
else:
print("Both functions returned False.")
在这个例子中,func1
函数返回真,因此不再判断func2
函数的返回值,最终输出结果为:
func1
At least one function returned True.
六、实际应用中的例子
1. 用户登录验证
在实际项目中,经常需要对用户的登录信息进行验证,判断用户名和密码是否正确:
username = "admin"
password = "123456"
input_username = input("Enter username: ")
input_password = input("Enter password: ")
if input_username == username and input_password == password:
print("Login successful.")
else:
print("Invalid username or password.")
2. 判断成绩等级
根据学生的成绩判断其等级,例如,成绩大于90为优秀,成绩在60到90之间为合格,成绩小于60为不合格:
score = int(input("Enter your score: "))
if score >= 90:
print("Excellent.")
elif score >= 60:
print("Pass.")
else:
print("Fail.")
七、总结
在Python中,同时判断两个条件可以使用逻辑运算符“and”和“or”。“and”用于判断两个条件都为真,“or”用于判断其中一个条件为真。在实际编程中,合理使用逻辑运算符可以使代码更加简洁明了。在复杂逻辑判断中,可以将“and”和“or”嵌套使用,并注意逻辑运算符的优先级和短路效应。通过理解和掌握这些知识,可以更好地编写高效、易读的Python代码。
相关问答FAQs:
在Python中,如何有效地使用逻辑运算符来同时判断多个条件?
在Python中,可以使用逻辑运算符and
、or
和not
来同时判断多个条件。and
运算符用于判断多个条件是否同时为真,只有当所有条件都为真时,结果才为真。相对地,or
运算符只要有一个条件为真,结果就为真。使用这些运算符可以让代码逻辑更加清晰与简洁。例如:
if condition1 and condition2:
# 执行某些操作
如何在Python中处理多个条件的复杂判断?
在处理复杂判断时,可以将条件组合成更易于理解的表达式。可以使用括号来明确条件的优先级,从而避免逻辑混淆。例如:
if (condition1 and condition2) or condition3:
# 执行特定操作
这种结构使得代码更具可读性,并且易于维护。
有什么常见的错误需要避免,在同时判断多个条件时?
在同时判断多个条件时,常见的错误包括逻辑顺序错误和条件拼写错误。确保每个条件的逻辑关系清晰,避免混淆and
与or
的使用。此外,注意条件的有效性,确保所有变量和表达式在执行判断前都已正确定义。这些细节对于避免运行时错误至关重要。
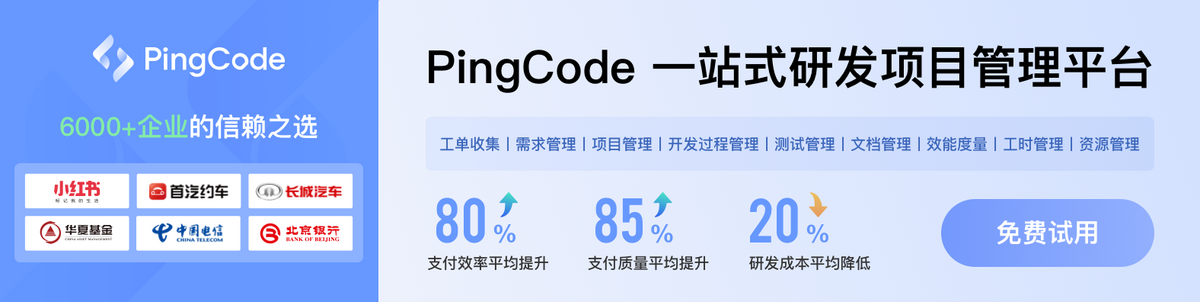