要用Python进行情感分析结果的可视化,可以使用数据可视化库如Matplotlib、Seaborn、Plotly等。首先,我们需要对文本数据进行情感分析,然后将结果可视化。 接下来,我将详细介绍如何在Python中进行情感分析并可视化结果的步骤。
一、准备数据和情感分析工具
为了进行情感分析,我们首先需要一组文本数据。这些数据可以来自社交媒体评论、客户反馈、新闻文章等。常用的情感分析工具包括TextBlob、VADER、和NLTK的情感词典。我们将使用VADER(Valence Aware Dictionary and sEntiment Reasoner)进行情感分析。
- 安装所需库
pip install nltk
pip install vaderSentiment
pip install matplotlib
pip install seaborn
pip install pandas
- 导入必要的库
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
from vaderSentiment.vaderSentiment import SentimentIntensityAnalyzer
- 准备示例数据
data = {
'text': [
'I love this product!',
'This is the worst service ever.',
'Absolutely fantastic!',
'Not very good, I am disappointed.',
'It was okay, nothing special.',
'Great value for the price.',
'I will never buy this again.',
'Totally worth it!',
'Terrible experience.',
'I am happy with the purchase.'
]
}
df = pd.DataFrame(data)
二、进行情感分析
使用VADER对文本数据进行情感分析,并将结果添加到数据框中。
- 初始化情感分析工具
analyzer = SentimentIntensityAnalyzer()
- 计算情感得分
def analyze_sentiment(text):
sentiment = analyzer.polarity_scores(text)
return sentiment['compound']
df['sentiment_score'] = df['text'].apply(analyze_sentiment)
- 分类情感
def categorize_sentiment(score):
if score >= 0.05:
return 'Positive'
elif score <= -0.05:
return 'Negative'
else:
return 'Neutral'
df['sentiment'] = df['sentiment_score'].apply(categorize_sentiment)
三、数据可视化
使用Matplotlib和Seaborn对情感分析结果进行可视化。
- 柱状图可视化情感分类
plt.figure(figsize=(10, 6))
sns.countplot(x='sentiment', data=df, palette='viridis')
plt.title('Sentiment Analysis')
plt.xlabel('Sentiment')
plt.ylabel('Count')
plt.show()
- 分布图可视化情感得分
plt.figure(figsize=(10, 6))
sns.histplot(df['sentiment_score'], kde=True, color='blue')
plt.title('Sentiment Score Distribution')
plt.xlabel('Sentiment Score')
plt.ylabel('Frequency')
plt.show()
- 散点图可视化情感得分
plt.figure(figsize=(10, 6))
sns.scatterplot(x=df.index, y='sentiment_score', data=df, hue='sentiment', palette='viridis')
plt.title('Sentiment Score Scatter Plot')
plt.xlabel('Index')
plt.ylabel('Sentiment Score')
plt.show()
四、深入分析
我们可以通过进一步的分析来获得更多的洞察。例如,分析不同情感类别的平均得分、对比不同时间段的情感变化等。
- 分析不同情感类别的平均得分
average_scores = df.groupby('sentiment')['sentiment_score'].mean()
print(average_scores)
- 对比不同时间段的情感变化
假设我们有一个包含时间戳的数据集,我们可以按时间段进行分析。
# 示例数据包含时间戳
data_with_time = {
'text': [
'I love this product!',
'This is the worst service ever.',
'Absolutely fantastic!',
'Not very good, I am disappointed.',
'It was okay, nothing special.',
'Great value for the price.',
'I will never buy this again.',
'Totally worth it!',
'Terrible experience.',
'I am happy with the purchase.'
],
'timestamp': pd.date_range(start='2023-01-01', periods=10, freq='D')
}
df_time = pd.DataFrame(data_with_time)
df_time['sentiment_score'] = df_time['text'].apply(analyze_sentiment)
df_time['sentiment'] = df_time['sentiment_score'].apply(categorize_sentiment)
按天计算平均得分
daily_sentiment = df_time.groupby(df_time['timestamp'].dt.date)['sentiment_score'].mean()
plt.figure(figsize=(10, 6))
daily_sentiment.plot(kind='line')
plt.title('Daily Sentiment Score')
plt.xlabel('Date')
plt.ylabel('Average Sentiment Score')
plt.show()
通过上述步骤,我们不仅能够使用Python进行情感分析,还能够通过多种方式对分析结果进行可视化,从而帮助我们更好地理解数据的情感分布和变化趋势。这些可视化结果可以用于报告、决策支持以及进一步的数据分析。
相关问答FAQs:
如何选择合适的可视化库来展示情感分析结果?
在Python中,有许多可视化库可以帮助展示情感分析的结果。常用的库包括Matplotlib、Seaborn和Plotly等。Matplotlib非常适合基本的图表绘制,而Seaborn在视觉上更为美观,尤其适合展示复杂的数据集。Plotly则能够生成交互式图表,适合需要更高用户交互的场景。选择时可以考虑数据的复杂性和展示的需求。
情感分析结果可视化的最佳实践是什么?
在可视化情感分析结果时,确保图表清晰易懂是关键。使用合适的颜色来区分不同情感类别(如积极、消极和中性)可以帮助观众快速理解数据。可视化时还可以添加数据标签,以便更好地传达具体数值。此外,提供适当的图例和标题也是提升可读性的重要因素。
如何处理情感分析中数据的不平衡性以提高可视化效果?
在情感分析中,数据可能存在类别不平衡的情况,例如积极评论远多于消极评论。为了解决这个问题,可以在可视化中采用归一化的方法,比如使用百分比而不是绝对数量来展示各类情感的比例。此外,堆叠柱状图或面积图也可以帮助清晰地展示各类别的相对比例,从而避免因数量差异造成的误导性解读。
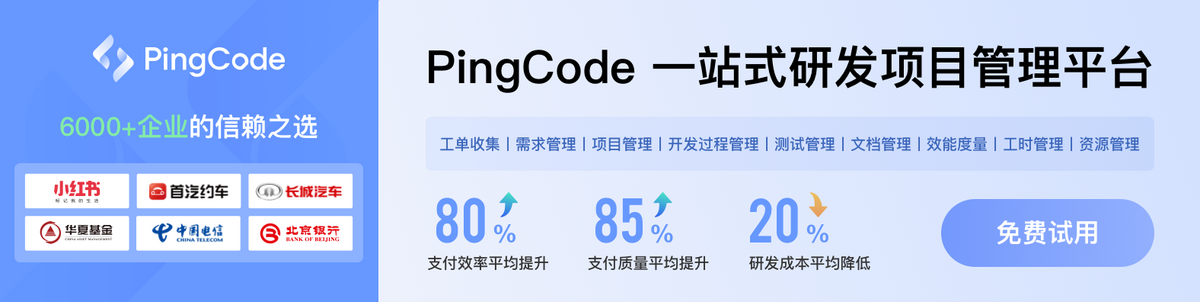