利用Python批量获知城市经纬度的主要方法有:使用地理编码API、利用Python地理编码库、批量处理数据。使用地理编码API是最常见和方便的方法之一。通过调用诸如Google Maps API或OpenStreetMap等服务,可以轻松获取多个城市的经纬度数据。下面将详细介绍如何使用Google Maps API来批量获取城市经纬度。
一、使用地理编码API
1、Google Maps Geocoding API
Google Maps Geocoding API是一种强大的工具,可以将地址转换为地理坐标(纬度和经度)。首先,你需要获取一个Google Cloud Platform API密钥。下面是如何使用Python调用Google Maps Geocoding API的示例:
import requests
def get_geocode(address, api_key):
base_url = 'https://maps.googleapis.com/maps/api/geocode/json'
params = {
'address': address,
'key': api_key
}
response = requests.get(base_url, params=params)
if response.status_code == 200:
data = response.json()
if len(data['results']) > 0:
location = data['results'][0]['geometry']['location']
return (location['lat'], location['lng'])
else:
return None
else:
return None
api_key = 'YOUR_API_KEY'
address = 'New York'
print(get_geocode(address, api_key))
在上述代码中,我们定义了一个get_geocode
函数,接受一个地址和API密钥作为输入,并返回地址的经纬度。如果请求成功并且结果不为空,我们将返回第一个结果的地理坐标。
2、批量处理多个城市
要批量获取多个城市的经纬度,可以将城市名称存储在一个列表中,然后迭代该列表并调用get_geocode
函数:
cities = ['New York', 'Los Angeles', 'Chicago', 'Houston', 'Phoenix']
api_key = 'YOUR_API_KEY'
city_coordinates = {}
for city in cities:
coordinates = get_geocode(city, api_key)
if coordinates:
city_coordinates[city] = coordinates
print(city_coordinates)
在这个示例中,我们定义了一个包含多个城市名称的列表cities
,并为每个城市调用get_geocode
函数。结果存储在city_coordinates
字典中,其中键是城市名称,值是经纬度。
二、利用Python地理编码库
除了直接使用API外,还可以利用一些Python地理编码库,例如geopy
,它提供了一个统一的接口来访问多个地理编码服务。
1、安装和使用geopy
首先,安装geopy
库:
pip install geopy
然后,可以使用以下代码来获取城市的经纬度:
from geopy.geocoders import Nominatim
def get_geocode_geopy(address):
geolocator = Nominatim(user_agent="geoapi")
location = geolocator.geocode(address)
if location:
return (location.latitude, location.longitude)
else:
return None
address = 'New York'
print(get_geocode_geopy(address))
在这个示例中,我们使用Nominatim
地理编码器,这是OpenStreetMap的一个服务。我们定义了一个get_geocode_geopy
函数来获取地址的经纬度。
2、批量处理多个城市
与之前的示例类似,可以批量处理多个城市:
cities = ['New York', 'Los Angeles', 'Chicago', 'Houston', 'Phoenix']
city_coordinates = {}
for city in cities:
coordinates = get_geocode_geopy(city)
if coordinates:
city_coordinates[city] = coordinates
print(city_coordinates)
三、批量处理数据
1、从文件读取城市名称
在实际应用中,城市名称可能存储在文件中,例如CSV文件。可以使用pandas
库读取这些文件并处理数据:
import pandas as pd
读取CSV文件
cities_df = pd.read_csv('cities.csv')
获取城市名称列表
cities = cities_df['City'].tolist()
city_coordinates = {}
for city in cities:
coordinates = get_geocode(city, api_key)
if coordinates:
city_coordinates[city] = coordinates
将结果保存到新的CSV文件
output_df = pd.DataFrame(list(city_coordinates.items()), columns=['City', 'Coordinates'])
output_df.to_csv('city_coordinates.csv', index=False)
在这个示例中,我们使用pandas
库读取CSV文件中的城市名称,并将结果保存到新的CSV文件中。
2、处理大规模数据
对于大规模数据,可以考虑使用多线程或多进程来提高性能。以下是使用concurrent.futures
库的示例:
import concurrent.futures
def get_city_coordinates(city):
return (city, get_geocode(city, api_key))
cities = ['New York', 'Los Angeles', 'Chicago', 'Houston', 'Phoenix']
city_coordinates = {}
with concurrent.futures.ThreadPoolExecutor(max_workers=5) as executor:
future_to_city = {executor.submit(get_city_coordinates, city): city for city in cities}
for future in concurrent.futures.as_completed(future_to_city):
city = future_to_city[future]
try:
city, coordinates = future.result()
if coordinates:
city_coordinates[city] = coordinates
except Exception as e:
print(f"Error retrieving coordinates for {city}: {e}")
print(city_coordinates)
在这个示例中,我们使用线程池来并行处理多个城市的地理编码请求,从而提高处理速度。
四、总结
利用Python批量获知城市经纬度的方法主要包括使用地理编码API、利用Python地理编码库以及批量处理数据。使用地理编码API(如Google Maps Geocoding API)是最常见的方法,通过调用API可以轻松获取多个城市的经纬度;利用Python地理编码库(如geopy
)可以简化编码过程;批量处理数据时可以使用pandas
库来读取和保存数据,并使用多线程或多进程来提高性能。通过结合这些方法,可以高效地批量获取城市的经纬度信息。
相关问答FAQs:
如何使用Python获取多个城市的经纬度信息?
可以通过使用Python中的地理编码库,如Geopy,来批量获取城市的经纬度。通过将城市名称传递给Geopy的地理编码器,您可以轻松获取每个城市的经度和纬度。需要注意的是,确保在代码中处理异常,以防某些城市名称无法解析。
有哪些API可以帮助获取城市的经纬度?
常用的API包括Google Maps Geocoding API、OpenCage Geocoding API和Nominatim(基于OpenStreetMap)。这些API提供了强大的地理编码功能,可以通过城市名称批量请求经纬度数据。在使用API时,需查看相关文档以了解请求限制和使用费用。
在获取城市经纬度时,如何确保数据的准确性?
为了提高城市经纬度数据的准确性,可以考虑使用多个来源的数据进行交叉验证。此外,处理数据时应注意城市名称的拼写和格式,确保一致性。使用标准化的城市名称格式可以有效减少错误和重复查询的情况。
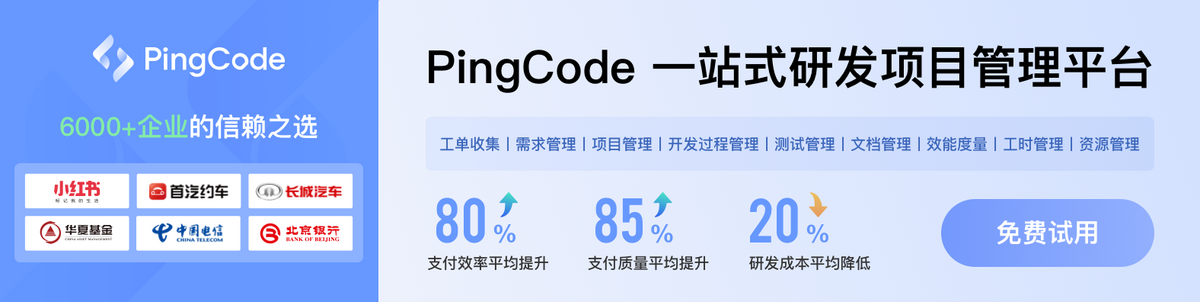