使用Python计算两点间距离的方法有多种,常见的方法包括使用欧几里得距离公式、曼哈顿距离、哈弗辛公式等。 其中,最常用的是欧几里得距离公式,因为它适用于多种应用场景,特别是在二维或三维空间中。本文将详细介绍如何使用这些方法计算两点间的距离,并提供相关的代码示例。
一、使用欧几里得距离公式
欧几里得距离是最常见的距离度量方法之一,适用于平面上的任意两点。计算公式如下:
[ d = \sqrt{(x_2 – x_1)^2 + (y_2 – y_1)^2} ]
代码示例
import math
def euclidean_distance(point1, point2):
return math.sqrt((point1[0] - point2[0])<strong>2 + (point1[1] - point2[1])</strong>2)
示例
point1 = (1, 2)
point2 = (4, 6)
distance = euclidean_distance(point1, point2)
print(f"Euclidean Distance: {distance}")
二、使用曼哈顿距离
曼哈顿距离也称为L1距离,是一种基于网格的距离度量方法。计算公式如下:
[ d = |x_2 – x_1| + |y_2 – y_1| ]
代码示例
def manhattan_distance(point1, point2):
return abs(point1[0] - point2[0]) + abs(point1[1] - point2[1])
示例
point1 = (1, 2)
point2 = (4, 6)
distance = manhattan_distance(point1, point2)
print(f"Manhattan Distance: {distance}")
三、使用哈弗辛公式(适用于地理坐标)
哈弗辛公式主要用于计算地球表面两点间的距离。计算公式较为复杂,但Python的math
库能够很好地支持这些计算。
代码示例
import math
def haversine_distance(coord1, coord2):
R = 6371 # 地球半径,单位为千米
lat1, lon1 = coord1
lat2, lon2 = coord2
dlat = math.radians(lat2 - lat1)
dlon = math.radians(lon2 - lon1)
a = math.sin(dlat / 2)<strong>2 + math.cos(math.radians(lat1)) * math.cos(math.radians(lat2)) * math.sin(dlon / 2)</strong>2
c = 2 * math.atan2(math.sqrt(a), math.sqrt(1 - a))
distance = R * c
return distance
示例
coord1 = (52.2296756, 21.0122287) # 华沙
coord2 = (41.8919300, 12.5113300) # 罗马
distance = haversine_distance(coord1, coord2)
print(f"Haversine Distance: {distance} km")
四、使用SciPy库计算多种距离
Python的SciPy库提供了多种距离计算方法,包括欧几里得距离、曼哈顿距离、切比雪夫距离等,可以方便地应用于多种场景。
代码示例
from scipy.spatial import distance
def calculate_distances(point1, point2):
euclidean = distance.euclidean(point1, point2)
manhattan = distance.cityblock(point1, point2)
chebyshev = distance.chebyshev(point1, point2)
return euclidean, manhattan, chebyshev
示例
point1 = (1, 2)
point2 = (4, 6)
distances = calculate_distances(point1, point2)
print(f"Euclidean Distance: {distances[0]}")
print(f"Manhattan Distance: {distances[1]}")
print(f"Chebyshev Distance: {distances[2]}")
五、应用场景和性能优化
在实际应用中,选择合适的距离计算方法取决于具体的业务场景。例如,欧几里得距离适用于几何问题,曼哈顿距离适用于网格布局,哈弗辛公式适用于地理信息系统(GIS)。
性能优化:在大规模数据处理中,计算距离可能会成为瓶颈。可以通过以下方法进行优化:
- 矢量化操作:使用NumPy进行矢量化计算,提高计算效率。
- 并行计算:使用多线程或多进程进行并行计算,减少计算时间。
- 数据降维:通过降维技术(如PCA)减少计算量。
矢量化操作示例
import numpy as np
def vectorized_euclidean_distance(points1, points2):
return np.linalg.norm(points1 - points2, axis=1)
示例
points1 = np.array([[1, 2], [3, 4], [5, 6]])
points2 = np.array([[4, 6], [6, 8], [8, 10]])
distances = vectorized_euclidean_distance(points1, points2)
print(f"Vectorized Euclidean Distances: {distances}")
六、总结
使用Python计算两点间距离的方法有多种,选择合适的距离计算方法取决于具体的应用场景。通过本文的介绍,我们了解了欧几里得距离、曼哈顿距离、哈弗辛公式等常见方法及其应用场景。同时,借助SciPy库和NumPy库,可以实现更高效的距离计算。在大规模数据处理场景下,性能优化是关键,可以通过矢量化操作和并行计算等方法提高效率。
相关问答FAQs:
如何使用Python计算两点之间的距离?
要计算两点之间的距离,您可以使用数学库中的公式。常用的欧几里得距离公式为:d = √((x2 – x1)² + (y2 – y1)²)。您可以通过导入math
库,并使用math.sqrt()
函数来实现这一点。示例代码如下:
import math
def calculate_distance(point1, point2):
return math.sqrt((point2[0] - point1[0])<strong>2 + (point2[1] - point1[1])</strong>2)
distance = calculate_distance((1, 2), (4, 6))
print(distance)
Python中还有哪些其他方式可以计算两点距离?
除了使用欧几里得距离外,您可以使用numpy
库来计算距离。numpy
提供了强大的数组操作功能,您可以通过以下方式实现:
import numpy as np
point1 = np.array([1, 2])
point2 = np.array([4, 6])
distance = np.linalg.norm(point2 - point1)
print(distance)
此外,您也可以考虑使用scipy.spatial.distance
模块来计算各种类型的距离,如曼哈顿距离或余弦相似度。
在计算距离时,如何处理三维或更高维的点?
在处理三维或更高维的点时,您可以扩展欧几里得距离的公式。对于三维空间,公式为:d = √((x2 – x1)² + (y2 – y1)² + (z2 – z1)²)。只需在代码中增加额外的维度即可。例如:
def calculate_3d_distance(point1, point2):
return math.sqrt((point2[0] - point1[0])<strong>2 + (point2[1] - point1[1])</strong>2 + (point2[2] - point1[2])**2)
distance = calculate_3d_distance((1, 2, 3), (4, 6, 8))
print(distance)
此方法适用于任意维度,只需确保您的点坐标是相同长度的元组或列表。
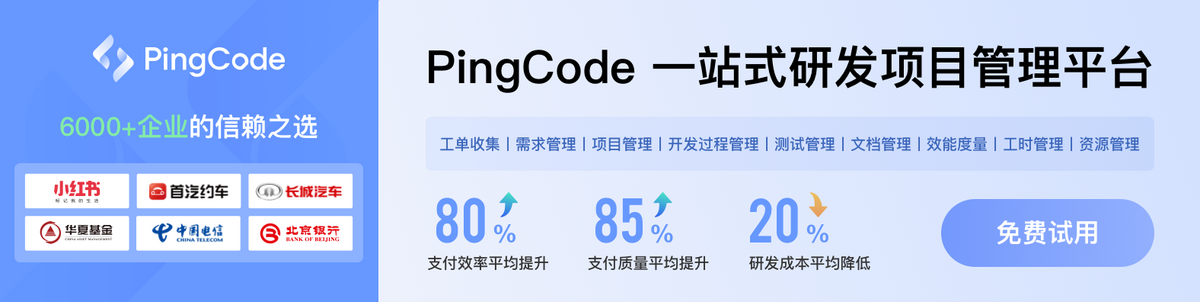