如何用Python计算并排名分数
用Python计算并排名分数的方法有很多种,最常见的几种方法是:使用列表、字典和Pandas库。创建列表或字典来存储学生的分数、使用内置函数进行排序、使用Pandas库进行复杂的数据操作。其中,Pandas库因其强大的数据处理能力和简洁的代码,常被用于复杂的数据分析任务。下面我们将详细探讨这几种方法的实现方式。
一、创建列表或字典来存储学生的分数
使用列表或字典来存储学生的分数是最基础的方式。这种方法适用于较小规模的数据集,因为它们操作起来相对简单且直接。
1. 使用列表存储分数
首先,我们可以使用列表来存储学生的分数,每个元素都是一个元组,包含学生的姓名和分数。以下是一个简单的示例:
# 创建一个包含学生姓名和分数的列表
students_scores = [("Alice", 85), ("Bob", 92), ("Charlie", 78), ("David", 90)]
按分数对列表进行排序
sorted_students_scores = sorted(students_scores, key=lambda x: x[1], reverse=True)
打印排序后的结果
for student, score in sorted_students_scores:
print(f"{student}: {score}")
在这个示例中,我们使用sorted
函数对列表进行排序,并通过lambda
函数指定按分数进行排序。reverse=True
参数表示按降序排序。
2. 使用字典存储分数
字典是一种键值对数据结构,适合存储和快速访问学生分数。以下是使用字典存储和排序分数的示例:
# 创建一个包含学生姓名和分数的字典
students_scores_dict = {"Alice": 85, "Bob": 92, "Charlie": 78, "David": 90}
按分数对字典进行排序,并转换为列表
sorted_students_scores_dict = sorted(students_scores_dict.items(), key=lambda x: x[1], reverse=True)
打印排序后的结果
for student, score in sorted_students_scores_dict:
print(f"{student}: {score}")
在这个示例中,我们将字典的items
方法返回的键值对列表进行排序,并使用lambda
函数指定按分数排序。
二、使用内置函数进行排序
Python内置函数如sorted
和sort
可以方便地对列表进行排序。sorted
函数返回一个新的排序列表,而sort
方法则是直接在原列表上进行排序。
1. sorted
函数
sorted
函数适用于需要保留原列表不变的情况。以下是一个示例:
# 创建一个包含学生姓名和分数的列表
students_scores = [("Alice", 85), ("Bob", 92), ("Charlie", 78), ("David", 90)]
使用sorted函数进行排序
sorted_students_scores = sorted(students_scores, key=lambda x: x[1], reverse=True)
打印排序后的结果
for student, score in sorted_students_scores:
print(f"{student}: {score}")
2. sort
方法
sort
方法适用于直接在原列表上进行排序。以下是一个示例:
# 创建一个包含学生姓名和分数的列表
students_scores = [("Alice", 85), ("Bob", 92), ("Charlie", 78), ("David", 90)]
使用sort方法进行排序
students_scores.sort(key=lambda x: x[1], reverse=True)
打印排序后的结果
for student, score in students_scores:
print(f"{student}: {score}")
三、使用Pandas库进行复杂的数据操作
Pandas是一个强大的数据处理库,适用于处理大型和复杂的数据集。使用Pandas可以简化数据操作过程,并提供丰富的功能来处理数据。
1. 安装Pandas库
如果你还没有安装Pandas库,可以使用以下命令进行安装:
pip install pandas
2. 使用Pandas处理和排序分数
以下是一个使用Pandas库来计算并排名分数的示例:
import pandas as pd
创建一个包含学生姓名和分数的DataFrame
data = {"Name": ["Alice", "Bob", "Charlie", "David"], "Score": [85, 92, 78, 90]}
df = pd.DataFrame(data)
按分数进行排序
sorted_df = df.sort_values(by="Score", ascending=False)
打印排序后的结果
print(sorted_df)
在这个示例中,我们创建了一个包含学生姓名和分数的DataFrame,并使用sort_values
方法按分数进行排序。ascending=False
参数表示按降序排序。
四、综合应用实例
下面我们将综合使用上述方法来创建一个完整的应用实例,该实例能够从用户输入获取分数数据,并输出排序后的结果。
import pandas as pd
def get_student_scores():
students_scores = []
while True:
name = input("Enter student name (or 'done' to finish): ")
if name.lower() == 'done':
break
try:
score = int(input(f"Enter score for {name}: "))
students_scores.append((name, score))
except ValueError:
print("Invalid score, please enter an integer.")
return students_scores
def sort_and_display_scores(students_scores):
# 使用Pandas进行排序
df = pd.DataFrame(students_scores, columns=["Name", "Score"])
sorted_df = df.sort_values(by="Score", ascending=False)
# 打印排序后的结果
print("\nSorted scores:")
print(sorted_df)
if __name__ == "__main__":
students_scores = get_student_scores()
sort_and_display_scores(students_scores)
在这个综合应用实例中,我们定义了两个函数:get_student_scores
和sort_and_display_scores
。get_student_scores
函数用于从用户输入获取学生姓名和分数,并将其存储在一个列表中;sort_and_display_scores
函数则使用Pandas库对分数进行排序并显示结果。
五、总结
通过上述方法,我们可以使用Python轻松地计算并排名分数。创建列表或字典来存储学生的分数、使用内置函数进行排序、使用Pandas库进行复杂的数据操作,这些方法各有优劣,适用于不同的场景和需求。在处理较小规模的数据集时,列表和字典是简单有效的选择;而在处理大型和复杂的数据集时,Pandas库则是更为强大和灵活的工具。
在实际应用中,我们可以根据具体需求选择合适的方法,并灵活运用Python的各种数据处理和排序功能来实现目标。希望本文能够帮助你更好地理解和掌握Python计算并排名分数的方法。
相关问答FAQs:
如何在Python中计算分数的平均值?
在Python中,可以使用内置的sum()
和len()
函数来计算分数的平均值。首先,将所有分数存储在一个列表中,然后使用sum()
函数计算总分,再用len()
函数获取分数的数量,最后用总分除以数量即可得到平均值。以下是一个简单的示例代码:
scores = [85, 90, 78, 92, 88]
average_score = sum(scores) / len(scores)
print("平均分数为:", average_score)
如何在Python中对分数进行排序?
要对分数进行排序,可以使用Python内置的sorted()
函数或列表的sort()
方法。sorted()
函数返回一个新的已排序列表,而sort()
方法会直接修改原列表。以下是使用这两种方法的示例:
scores = [85, 90, 78, 92, 88]
sorted_scores = sorted(scores) # 返回一个新列表
print("已排序的分数:", sorted_scores)
scores.sort() # 原地排序
print("原列表已排序:", scores)
如何在Python中为分数排名?
在Python中,可以使用enumerate()
函数结合sorted()
函数来为分数排名。首先,使用enumerate()
创建一个包含索引和分数的元组列表,然后根据分数排序。最后,输出排名的结果。以下是一个示例代码:
scores = [85, 90, 78, 92, 88]
ranked_scores = sorted(enumerate(scores), key=lambda x: x[1], reverse=True)
for rank, (index, score) in enumerate(ranked_scores, start=1):
print(f"分数 {score} 的排名是: {rank}")
这些方法可以帮助你在Python中有效地计算和排名分数,适用于各种数据分析和处理任务。
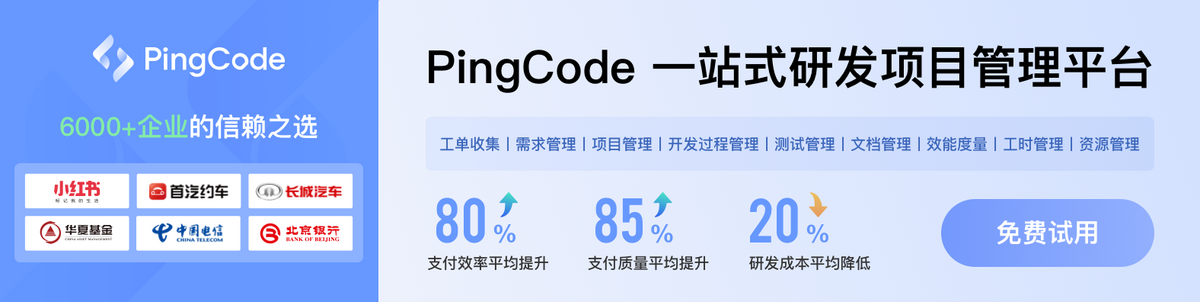