在Python中查看列表某一值的索引,可以使用index
方法、循环遍历、enumerate
函数、列表推导式等方法。 index
方法最为常用,因为它简单直接。如果需要处理值不存在的情况,可以使用异常处理机制来避免错误。enumerate
函数和列表推导式则提供了更灵活的方式,可以根据需要选择合适的方法。下面我将详细展开其中一种方法。
使用index
方法时,可以通过异常处理机制来避免在值不存在时发生错误。例如:
my_list = [10, 20, 30, 40, 50]
try:
index = my_list.index(30)
print(f"Index of 30: {index}")
except ValueError:
print("Value not found in the list")
这样可以确保在值不存在时程序不会崩溃,同时提供了用户友好的反馈。
一、使用 index
方法
index
方法是最简单、直接的方式来查找某一值在列表中的索引。它的使用方法如下:
my_list = [10, 20, 30, 40, 50]
index = my_list.index(30)
print(f"Index of 30: {index}")
这种方法的优点是简洁明了,但如果要查找的值不在列表中,会抛出ValueError
异常。因此在实际应用中,通常需要配合异常处理机制。
异常处理
为了避免程序因查找不到值而崩溃,可以使用try
和except
块来捕获异常:
my_list = [10, 20, 30, 40, 50]
try:
index = my_list.index(30)
print(f"Index of 30: {index}")
except ValueError:
print("Value not found in the list")
这样可以确保程序健壮性,并提供友好的用户反馈。
二、使用循环遍历
循环遍历列表是一种更灵活的方法,尤其适用于需要查找多个相同值的索引或者需要进行复杂条件判断的情况。
my_list = [10, 20, 30, 40, 50]
search_value = 30
for i in range(len(my_list)):
if my_list[i] == search_value:
print(f"Index of {search_value}: {i}")
break
这种方法的优点是灵活,可以根据需要在循环中进行各种条件判断和处理。
三、使用 enumerate
函数
enumerate
函数是Python内置的一个实用函数,可以在遍历列表时同时获取索引和值,非常方便。
my_list = [10, 20, 30, 40, 50]
search_value = 30
for index, value in enumerate(my_list):
if value == search_value:
print(f"Index of {search_value}: {index}")
break
使用enumerate
函数可以使代码更加简洁和易读。
四、使用列表推导式
列表推导式是一种简洁优雅的方式来生成列表,但它也可以用来查找某一值的索引。
my_list = [10, 20, 30, 40, 50]
search_value = 30
indices = [index for index, value in enumerate(my_list) if value == search_value]
if indices:
print(f"Index of {search_value}: {indices[0]}")
else:
print("Value not found in the list")
这种方法的优点是简洁,但可能不如index
方法和循环遍历那么直观。
五、查找所有匹配的索引
有时候列表中可能包含多个相同的值,此时需要查找所有匹配的索引。可以使用循环遍历或enumerate
函数来实现:
my_list = [10, 20, 30, 30, 50]
search_value = 30
indices = []
for index, value in enumerate(my_list):
if value == search_value:
indices.append(index)
print(f"Indices of {search_value}: {indices}")
这种方法可以确保找到所有匹配值的索引。
六、性能考虑
在处理大列表时,性能可能成为一个问题。一般来说,index
方法和enumerate
函数的性能都比较高,但循环遍历可能会在列表非常大时导致性能下降。使用合适的数据结构和算法可以提高性能,例如使用字典来存储值和索引的映射关系。
my_list = [10, 20, 30, 40, 50]
value_to_index = {value: index for index, value in enumerate(my_list)}
search_value = 30
index = value_to_index.get(search_value, -1)
print(f"Index of {search_value}: {index}")
这种方法在列表非常大时性能优势明显,因为字典的查找操作是常数时间复杂度。
七、总结
在Python中查看列表某一值的索引有多种方法,选择合适的方法取决于具体的应用场景。index
方法最为简单直接,异常处理机制可以提高程序的健壮性,循环遍历和enumerate
函数提供了更大的灵活性,列表推导式简洁优雅,查找所有匹配的索引需要注意使用合适的方式,性能考虑在处理大列表时尤为重要。
相关问答FAQs:
如何在Python中查找列表中特定值的索引?
在Python中,可以使用list.index()
方法来查找列表中特定值的索引。例如,如果有一个列表my_list = [10, 20, 30, 40]
,要查找值30
的索引,可以使用my_list.index(30)
,这将返回2
,因为30
在列表中的位置是索引2
。需要注意的是,如果列表中没有该值,将会抛出ValueError
异常。
如果列表中有多个相同的值,如何找到所有这些值的索引?
要找到列表中所有相同值的索引,可以使用列表解析(list comprehension)结合enumerate()
函数。例如,对于列表my_list = [10, 20, 30, 20]
,如果你想找到所有值为20
的索引,可以使用[index for index, value in enumerate(my_list) if value == 20]
,这将返回一个包含所有索引的列表,例如[1, 3]
。
如何处理查找索引时的异常情况?
当使用list.index()
方法查找索引时,如果要查找的值不在列表中,会抛出ValueError
。为了避免程序崩溃,可以使用try-except
结构来处理这种情况。例如:
try:
index = my_list.index(value)
except ValueError:
index = -1 # 或者其他处理方式
通过这种方式,可以安全地处理没有找到值的情况,返回一个默认值或进行其他处理。
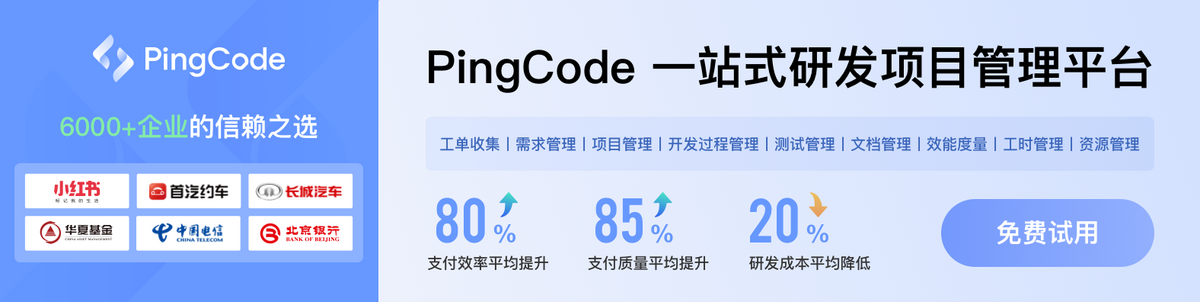