一、Python中去掉字符串中的空格的方法有多种,包括strip()、lstrip()、rstrip()、replace()、join()等。使用strip()去除字符串前后的空格、使用replace()去除字符串中所有空格、使用join()和split()结合去除字符串中所有空格。其中,使用replace()方法较为常见,因为它可以灵活地去除字符串中所有空格,而不仅限于前后或特定位置的空格。
在实际应用中,replace()方法非常有用,例如在处理用户输入时,去除多余的空格可以确保数据的一致性和准确性。此外,使用join()和split()方法组合也可以达到同样的效果,这种方法会先将字符串分割成单词,再合并为没有空格的新字符串。
二、Python去掉字符串前后的空格
1、strip()方法
text = " hello world "
result = text.strip()
print(result) # 输出: "hello world"
strip()方法用于去除字符串前后的空格。它不会去除字符串中间的空格。
2、lstrip()方法
text = " hello world "
result = text.lstrip()
print(result) # 输出: "hello world "
lstrip()方法用于去除字符串前面的空格。
3、rstrip()方法
text = " hello world "
result = text.rstrip()
print(result) # 输出: " hello world"
rstrip()方法用于去除字符串后面的空格。
三、Python去掉字符串中的所有空格
1、replace()方法
text = " hello world "
result = text.replace(" ", "")
print(result) # 输出: "helloworld"
replace()方法用于将字符串中的所有空格替换为其他字符,通常替换为空字符,从而去除所有空格。
2、join()和split()方法结合使用
text = " hello world "
result = "".join(text.split())
print(result) # 输出: "helloworld"
split()方法将字符串分割成单词列表,而join()方法将这些单词合并为一个没有空格的新字符串。这种方法也可以达到去除所有空格的效果。
四、Python去掉字符串中指定位置的空格
1、通过字符串切片
text = "hello world"
result = text[:5] + text[6:]
print(result) # 输出: "helloworld"
通过字符串切片,可以去除指定位置的空格。这种方法需要知道空格的具体位置。
2、使用正则表达式
import re
text = "hello world"
result = re.sub(r'\s+', '', text)
print(result) # 输出: "helloworld"
正则表达式提供了强大的字符串匹配和替换功能,使用re.sub()方法可以去除字符串中的所有空白字符,包括空格、制表符和换行符。
五、Python处理用户输入中的空格
1、去除前后空格
user_input = " user input "
cleaned_input = user_input.strip()
print(cleaned_input) # 输出: "user input"
在处理用户输入时,通常需要去除前后的空格,以确保数据的一致性。
2、去除所有空格
user_input = " user input "
cleaned_input = user_input.replace(" ", "")
print(cleaned_input) # 输出: "userinput"
去除用户输入中的所有空格,可以确保数据在存储和处理时更加简洁。
六、Python处理多行字符串中的空格
1、去除每行前后的空格
text = """
line 1
line 2
line 3
"""
lines = text.splitlines()
cleaned_lines = [line.strip() for line in lines]
cleaned_text = "\n".join(cleaned_lines)
print(cleaned_text)
在处理多行字符串时,可以逐行去除前后的空格,从而保持每行的整洁。
2、去除每行中的所有空格
text = """
line 1
line 2
line 3
"""
lines = text.splitlines()
cleaned_lines = [line.replace(" ", "") for line in lines]
cleaned_text = "\n".join(cleaned_lines)
print(cleaned_text)
逐行去除所有空格,可以确保多行字符串在存储和处理时更加简洁。
七、Python处理字符串中多余的空格
1、去除重复的空格
text = "hello world"
result = " ".join(text.split())
print(result) # 输出: "hello world"
通过split()和join()方法,可以去除字符串中重复的空格,只保留一个空格。
2、去除特定字符间的空格
text = "hello - world"
result = text.replace(" - ", "-")
print(result) # 输出: "hello-world"
通过replace()方法,可以去除特定字符间的空格,从而保持字符串的整洁。
八、Python处理文件中的空格
1、读取文件并去除空格
with open("file.txt", "r") as file:
lines = file.readlines()
cleaned_lines = [line.strip() for line in lines]
with open("cleaned_file.txt", "w") as file:
file.writelines(cleaned_lines)
在处理文件时,可以逐行读取文件内容,并去除每行前后的空格,然后将处理后的内容写入新文件。
2、去除文件内容中的所有空格
with open("file.txt", "r") as file:
content = file.read()
cleaned_content = content.replace(" ", "")
with open("cleaned_file.txt", "w") as file:
file.write(cleaned_content)
通过读取文件内容并去除所有空格,可以确保文件内容在存储和处理时更加简洁。
九、Python处理字符串中空格的常见问题
1、如何去除字符串中的制表符和换行符?
可以使用replace()方法或正则表达式去除字符串中的制表符和换行符。
text = "hello\tworld\n"
result = text.replace("\t", "").replace("\n", "")
print(result) # 输出: "helloworld"
2、如何去除字符串中的多种空白字符?
可以使用正则表达式去除字符串中的多种空白字符,包括空格、制表符和换行符。
import re
text = "hello\tworld\n"
result = re.sub(r'\s+', '', text)
print(result) # 输出: "helloworld"
3、如何高效地去除大字符串中的空格?
对于大字符串,可以使用正则表达式或字符串方法结合的方式高效地去除空格。
import re
text = "large string with many spaces"
result = re.sub(r'\s+', '', text)
print(result)
或者使用join()和split()方法结合的方式:
text = "large string with many spaces"
result = "".join(text.split())
print(result)
总之,在Python中去除字符串中的空格有多种方法,可以根据具体需求选择合适的方法。无论是去除前后的空格、所有空格,还是特定位置的空格,都可以通过字符串方法或正则表达式实现。在处理用户输入、文件内容和多行字符串时,去除空格可以确保数据的一致性和整洁性,从而提高数据处理的准确性和效率。
相关问答FAQs:
如何在Python中去掉字符串两端的空格?
在Python中,可以使用strip()
方法去掉字符串两端的空格。例如:
text = " Hello, World! "
cleaned_text = text.strip()
print(cleaned_text) # 输出: "Hello, World!"
这个方法也可以去掉其他指定的字符,只需将其作为参数传递给strip()
函数。
Python中如何去掉字符串中间的空格?
要去掉字符串中间的空格,可以使用replace()
方法,将空格替换为空字符串。例如:
text = "Hello, World! "
cleaned_text = text.replace(" ", "")
print(cleaned_text) # 输出: "Hello,World!"
这种方法会去掉字符串中所有的空格,包括两端和中间的。
在Python中如何使用正则表达式去掉空格?
使用re
模块中的sub()
函数,可以通过正则表达式更灵活地去掉字符串中的空格。示例如下:
import re
text = " Hello, World! "
cleaned_text = re.sub(r'\s+', ' ', text).strip()
print(cleaned_text) # 输出: "Hello, World!"
这个方法不仅去掉了多余的空格,还能将多个空格合并为一个空格。
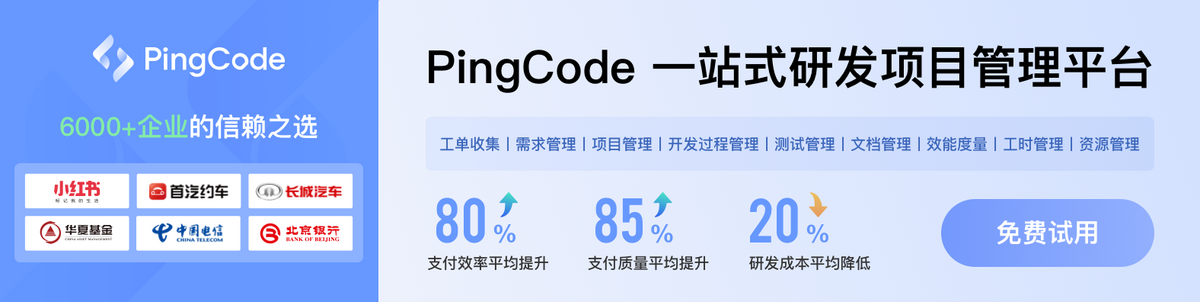