Python处理输入的字符串的常见方法有:使用input()函数获取输入、字符串切片、字符串方法(如strip()、split()、join()等)、正则表达式、格式化字符串。下面将详细解释其中一种方法——字符串方法。
在Python中,处理字符串是非常常见的任务。通过使用字符串方法,我们可以对输入的字符串进行各种操作,例如删除空格、分割字符串、替换字符等。下面我们将详细解释一些常用的字符串方法,并提供代码示例。
一、获取输入字符串
在Python中,获取用户输入的最常见方法是使用input()函数。input()函数将用户输入的内容作为字符串返回。以下是一个简单的例子:
user_input = input("请输入一个字符串:")
print(f"你输入的字符串是:{user_input}")
二、字符串方法
1、去除空格和特殊字符
有时候,用户输入的字符串可能包含前后的空格或其他不需要的特殊字符。我们可以使用strip()、lstrip()和rstrip()方法来去除这些字符。
user_input = " Hello, World! "
cleaned_input = user_input.strip()
print(f"去除空格后的字符串:'{cleaned_input}'")
2、分割字符串
split()方法可以将字符串按照指定的分隔符分割成一个列表。默认情况下,split()方法会按照空格分割字符串。
user_input = "Hello, World! Welcome to Python."
words = user_input.split()
print(f"分割后的单词列表:{words}")
3、拼接字符串
join()方法可以将一个列表中的元素拼接成一个字符串。我们可以指定一个分隔符来拼接这些元素。
words = ["Hello", "World", "Welcome", "to", "Python"]
sentence = " ".join(words)
print(f"拼接后的字符串:{sentence}")
4、替换字符
replace()方法可以将字符串中的某些字符替换为其他字符。
user_input = "Hello, World!"
new_input = user_input.replace("World", "Python")
print(f"替换后的字符串:{new_input}")
三、字符串切片
字符串切片是指从一个字符串中提取出一个子字符串。我们可以使用切片操作符[]来实现这一点。切片操作符的语法为[start:stop:step],其中start表示起始位置,stop表示结束位置(不包括该位置),step表示步长。
user_input = "Hello, World!"
substring = user_input[0:5]
print(f"提取的子字符串:{substring}")
四、正则表达式
正则表达式是一种强大的字符串处理工具,可以用来匹配、搜索和替换字符串中的特定模式。Python的re模块提供了对正则表达式的支持。
import re
user_input = "Hello, World! Welcome to Python."
pattern = r"\b\w+\b"
matches = re.findall(pattern, user_input)
print(f"匹配的单词列表:{matches}")
五、格式化字符串
格式化字符串可以让我们更方便地插入变量或表达式的值。Python提供了多种格式化字符串的方法,包括百分号格式化、str.format()方法和f-strings。
1、百分号格式化
name = "Python"
version = 3.9
formatted_string = "Welcome to %s version %.1f!" % (name, version)
print(formatted_string)
2、str.format()方法
name = "Python"
version = 3.9
formatted_string = "Welcome to {} version {:.1f}!".format(name, version)
print(formatted_string)
3、f-strings
name = "Python"
version = 3.9
formatted_string = f"Welcome to {name} version {version:.1f}!"
print(formatted_string)
六、综合示例
下面是一个综合示例,演示了如何使用上述方法处理用户输入的字符串:
import re
获取用户输入
user_input = input("请输入一个字符串:")
去除空格
cleaned_input = user_input.strip()
分割字符串
words = cleaned_input.split()
替换字符
new_input = cleaned_input.replace("World", "Python")
提取子字符串
substring = cleaned_input[0:5]
使用正则表达式匹配单词
pattern = r"\b\w+\b"
matches = re.findall(pattern, cleaned_input)
格式化字符串
formatted_string = f"你输入的字符串是:'{cleaned_input}'"
输出结果
print(formatted_string)
print(f"分割后的单词列表:{words}")
print(f"替换后的字符串:{new_input}")
print(f"提取的子字符串:{substring}")
print(f"匹配的单词列表:{matches}")
通过以上方法,我们可以对输入的字符串进行各种处理,使其符合我们的需求。这些方法不仅在日常编程中非常实用,还能帮助我们更好地理解和掌握Python的字符串处理能力。
相关问答FAQs:
如何在Python中读取用户输入的字符串?
在Python中,使用input()
函数可以轻松地读取用户输入的字符串。该函数会暂停程序执行,直到用户输入内容并按下回车键。例如,您可以使用以下代码来获取用户的名字:
name = input("请输入您的名字:")
print(f"你好,{name}!")
Python对字符串的处理有哪些常用方法?
Python提供了多种字符串处理方法,例如strip()
用于去除字符串两端的空白字符,split()
可以将字符串根据指定分隔符分割成列表,replace()
用于替换字符串中的某部分内容。以下是一些示例:
text = " Hello, World! "
clean_text = text.strip() # 去除空白
words = clean_text.split(", ") # 分割字符串
new_text = clean_text.replace("World", "Python") # 替换内容
在Python中如何进行字符串格式化?
字符串格式化在Python中可以通过多种方式实现,最常用的是使用f-string(格式化字符串字面量)和format()
方法。例如,您可以使用f-string来格式化输出:
name = "Alice"
age = 30
message = f"{name} is {age} years old."
print(message)
另一种方式是使用format()
方法:
message = "{} is {} years old.".format(name, age)
print(message)
这两种方法都可以使输出更加直观和易于阅读。
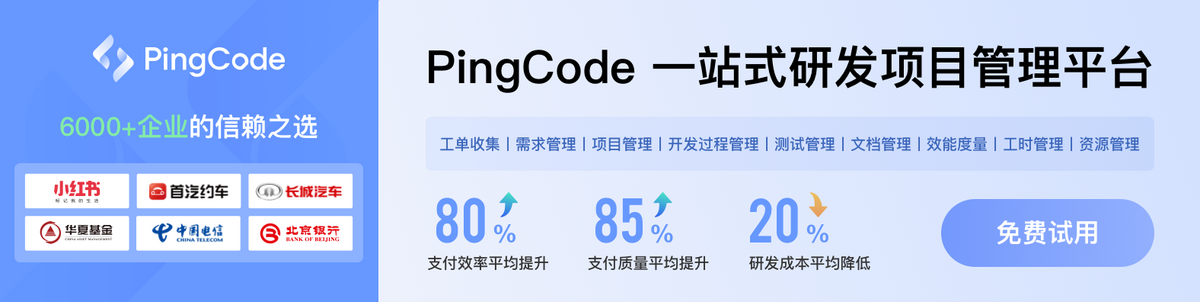