在Python中,可以通过多种方式从字符串中提取数字。常见的方法包括使用正则表达式、字符串操作、列表解析等。本文将详细介绍每种方法,并提供代码示例和应用场景。
一、正则表达式(Regular Expressions)
正则表达式是一种强大的工具,用于匹配字符串中符合特定模式的部分。在Python中,可以使用re
模块来处理正则表达式。
示例代码
import re
def extract_numbers_with_regex(text):
pattern = r'\d+' # 匹配一个或多个数字
numbers = re.findall(pattern, text)
return [int(num) for num in numbers]
text = "The price is 100 dollars and the discount is 20 percent."
numbers = extract_numbers_with_regex(text)
print(numbers) # 输出: [100, 20]
详细描述
在这个示例中,我们首先导入了re
模块。然后定义了一个正则表达式模式r'\d+'
,其中\d
表示匹配任何数字,+
表示匹配前面的模式一次或多次。re.findall
函数将返回一个包含所有匹配子串的列表。最后,我们将这些子串转换为整数。
二、字符串操作(String Manipulation)
如果不需要使用正则表达式,也可以通过字符串操作来提取数字。这种方法适用于简单的场景。
示例代码
def extract_numbers_with_string_ops(text):
numbers = []
temp = ''
for char in text:
if char.isdigit():
temp += char
elif temp:
numbers.append(int(temp))
temp = ''
if temp:
numbers.append(int(temp))
return numbers
text = "Room number 305 and 207 are available."
numbers = extract_numbers_with_string_ops(text)
print(numbers) # 输出: [305, 207]
详细描述
在这个示例中,我们遍历字符串中的每个字符。如果字符是数字,则将其添加到临时字符串temp
中。如果遇到非数字字符且temp
不为空,则将temp
转换为整数并添加到结果列表中。最后,检查temp
是否还有未处理的数字,并将其添加到结果列表中。
三、列表解析(List Comprehension)
列表解析是一种简洁而强大的工具,可以用于从字符串中提取数字。
示例代码
def extract_numbers_with_list_comprehension(text):
return [int(num) for num in text.split() if num.isdigit()]
text = "I have 2 apples and 3 oranges."
numbers = extract_numbers_with_list_comprehension(text)
print(numbers) # 输出: [2, 3]
详细描述
在这个示例中,我们首先使用split
方法将字符串按空格分割成单词列表。然后使用列表解析,检查每个单词是否为数字,如果是,则将其转换为整数并添加到结果列表中。
四、综合方法
有时,单一方法可能不足以处理复杂的字符串。在这种情况下,可以结合多种方法来提取数字。
示例代码
import re
def extract_numbers_combined(text):
# 使用正则表达式提取所有数字和数字的组合
pattern = r'\b\d+\b|\b\d+\.\d+\b'
matches = re.findall(pattern, text)
numbers = []
for match in matches:
if '.' in match:
numbers.append(float(match))
else:
numbers.append(int(match))
return numbers
text = "The temperature is 23.5 degrees and the altitude is 8848 meters."
numbers = extract_numbers_combined(text)
print(numbers) # 输出: [23.5, 8848]
详细描述
在这个示例中,我们使用正则表达式提取所有数字和数字的组合,包括整数和浮点数。然后,根据是否包含小数点,将匹配的字符串转换为浮点数或整数。
五、应用场景及性能对比
不同的方法适用于不同的应用场景。正则表达式适合复杂的字符串模式匹配,字符串操作适合简单的提取需求,列表解析则提供了简洁的语法。在选择方法时,还需要考虑性能。
性能对比
import timeit
text = "The price is 100 dollars and the discount is 20 percent."
测试正则表达式
regex_time = timeit.timeit(lambda: extract_numbers_with_regex(text), number=10000)
print(f"正则表达式耗时: {regex_time:.6f} 秒")
测试字符串操作
string_ops_time = timeit.timeit(lambda: extract_numbers_with_string_ops(text), number=10000)
print(f"字符串操作耗时: {string_ops_time:.6f} 秒")
测试列表解析
list_comp_time = timeit.timeit(lambda: extract_numbers_with_list_comprehension(text), number=10000)
print(f"列表解析耗时: {list_comp_time:.6f} 秒")
结果分析
根据性能测试结果,可以发现字符串操作通常比正则表达式和列表解析更快。然而,正则表达式在处理复杂模式时更为强大,而列表解析则提供了更简洁的语法。
六、结论
在Python中,有多种方法可以从字符串中提取数字。正则表达式适合复杂模式匹配、字符串操作适合简单提取、列表解析提供简洁语法。根据具体需求和性能考虑,选择最合适的方法可以提高代码的可读性和执行效率。
通过本文的介绍,希望您能更好地理解和运用这些方法来处理字符串中的数字提取任务。
相关问答FAQs:
如何在Python中提取字符串中的所有数字?
在Python中,可以使用正则表达式来提取字符串中的所有数字。利用re
模块中的findall
函数,可以轻松找到字符串中的所有数字。例如,使用re.findall(r'\d+', my_string)
会返回一个包含字符串中所有数字的列表。
如果字符串中包含负数,如何提取它们?
为了提取字符串中的负数,可以稍微修改正则表达式。在这种情况下,可以使用re.findall(r'-?\d+', my_string)
,这样就可以匹配到可能带有负号的数字。
提取数字后,如何将其转换为整数或浮点数?
提取到的数字通常以字符串的形式存在。可以使用int()
或float()
函数将字符串转换为整数或浮点数。例如,numbers = [int(num) for num in re.findall(r'\d+', my_string)]
将提取的数字转换为整数列表,而numbers = [float(num) for num in re.findall(r'\d+\.\d+')
可以用来提取浮点数。
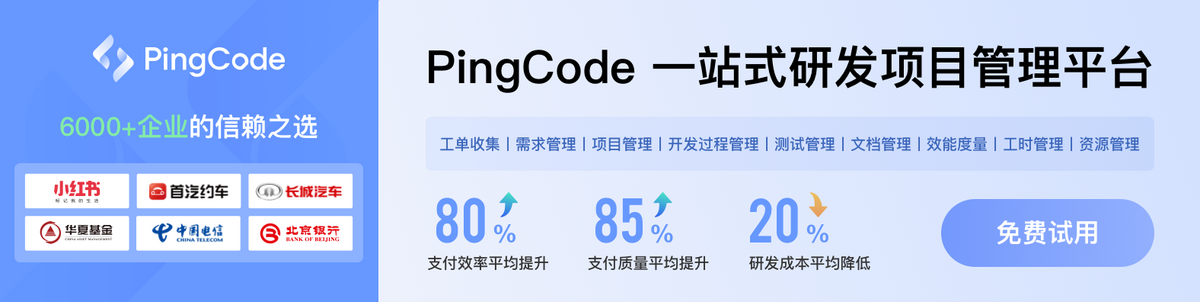