在Python类中,获取并输出类函数的名称可以通过遍历类的属性并使用内置函数来实现,包括 dir()
、getattr()
和 inspect
模块。下面将详细介绍如何在Python类中输出类函数的名称:
要在Python类中输出类函数的名称,可以使用以下几种方法:遍历类中的属性、使用内置函数来检查属性是否是函数、利用 inspect
模块来获取类的成员函数。其中一种常用的方法是使用 dir()
函数来列出类的所有属性和方法,再结合 getattr()
函数和 inspect.ismethod()
或 inspect.isfunction()
来筛选出函数。下面将详细描述其中的一种方法。
一、使用 dir()
和 getattr()
通过 dir()
函数可以获取类的所有属性和方法,然后使用 getattr()
函数和 callable()
函数来判断属性是否为方法,并输出它们的名称。
class MyClass:
def method1(self):
pass
def method2(self):
pass
def method3(self):
pass
@staticmethod
def static_method():
pass
@classmethod
def class_method(cls):
pass
获取类方法名称
def get_class_methods(cls):
methods = [func for func in dir(cls) if callable(getattr(cls, func)) and not func.startswith("__")]
return methods
输出类方法名称
methods = get_class_methods(MyClass)
print("Class Methods:", methods)
在这个例子中,get_class_methods
函数首先使用 dir()
获取类 MyClass
的所有属性和方法,然后通过 callable()
函数和 getattr()
函数筛选出方法,并忽略以双下划线开头的方法(如 __init__
、__str__
等),最终返回方法名称列表。
二、使用 inspect
模块
inspect
模块提供了更多的工具来获取类和对象的详细信息。使用 inspect.getmembers()
函数可以更方便地获取类的所有成员,并通过 inspect.isfunction()
或 inspect.ismethod()
来筛选出方法。
import inspect
class MyClass:
def method1(self):
pass
def method2(self):
pass
def method3(self):
pass
@staticmethod
def static_method():
pass
@classmethod
def class_method(cls):
pass
获取类方法名称
def get_class_methods(cls):
methods = [name for name, member in inspect.getmembers(cls, inspect.isfunction)]
return methods
输出类方法名称
methods = get_class_methods(MyClass)
print("Class Methods:", methods)
在这个例子中,inspect.getmembers()
函数获取类 MyClass
的所有成员,并通过 inspect.isfunction
筛选出函数,然后返回方法名称列表。
三、获取实例方法和类方法
有时需要区分实例方法、类方法和静态方法,可以分别使用 inspect.ismethod
、inspect.isfunction
和 inspect.isroutine
来区分并获取它们。
import inspect
class MyClass:
def method1(self):
pass
def method2(self):
pass
@staticmethod
def static_method():
pass
@classmethod
def class_method(cls):
pass
获取类方法名称
def get_class_methods(cls):
instance_methods = [name for name, member in inspect.getmembers(cls, inspect.isfunction)]
static_methods = [name for name, member in inspect.getmembers(cls, inspect.isroutine) if isinstance(member, staticmethod)]
class_methods = [name for name, member in inspect.getmembers(cls, inspect.isroutine) if isinstance(member, classmethod)]
return instance_methods, static_methods, class_methods
输出类方法名称
instance_methods, static_methods, class_methods = get_class_methods(MyClass)
print("Instance Methods:", instance_methods)
print("Static Methods:", static_methods)
print("Class Methods:", class_methods)
在这个例子中,分别获取并输出实例方法、静态方法和类方法的名称。
四、总结
通过以上几种方法,可以方便地获取并输出Python类中的方法名称。使用 dir()
和 getattr()
可以实现基本的功能,而使用 inspect
模块可以更精确地区分不同类型的方法。这些方法在调试和代码分析时非常有用,可以帮助开发者更好地理解和管理类中的方法。
相关问答FAQs:
如何在Python类中获取当前函数的名称?
在Python中,可以使用内置模块inspect
来获取当前函数的名称。通过inspect.currentframe()
获取当前栈帧,并通过f_code.co_name
获取函数名称。例如,可以在类的函数中使用如下代码:
import inspect
class MyClass:
def my_function(self):
function_name = inspect.currentframe().f_code.co_name
print(f"当前函数名称是: {function_name}")
obj = MyClass()
obj.my_function()
在类中如何动态输出多个函数的名称?
可以通过遍历类的__dict__
属性来获取类中所有函数的名称。使用callable()
函数检查是否为可调用对象,然后输出其名称。示例如下:
class MyClass:
def func_one(self):
pass
def func_two(self):
pass
def list_function_names(self):
function_names = [name for name, func in self.__class__.__dict__.items() if callable(func)]
print("类中的函数名称有:", function_names)
obj = MyClass()
obj.list_function_names()
使用类装饰器如何获取类函数名称?
类装饰器可以用于包装类中的方法,并在方法调用时输出函数名称。这是一种优雅的方式,能够在不修改原有代码结构的情况下实现功能。示例如下:
def function_name_decorator(func):
def wrapper(*args, **kwargs):
print(f"调用的函数名称是: {func.__name__}")
return func(*args, **kwargs)
return wrapper
class MyClass:
@function_name_decorator
def my_function(self):
print("执行 my_function")
obj = MyClass()
obj.my_function()
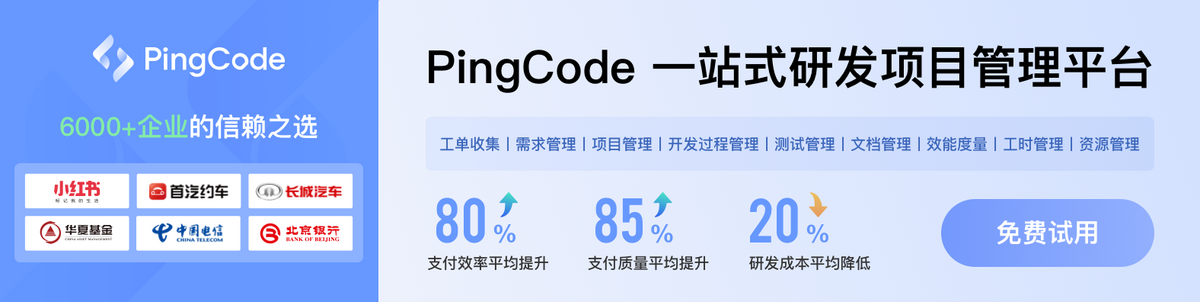