Python中列表里面有字典的遍历方法有以下几种:使用for循环、使用枚举、使用列表推导式。 其中,使用for循环是最常用的方法,它可以方便地访问列表中的每个字典,并根据需要处理字典中的键值对。下面我们详细展开介绍这几种方法。
一、使用for循环
使用for循环是遍历列表中字典的最常见方法。下面是一个例子:
list_of_dicts = [{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 30}, {'name': 'Charlie', 'age': 35}]
for dictionary in list_of_dicts:
for key, value in dictionary.items():
print(f"{key}: {value}")
在这个例子中,我们首先使用外层的for循环遍历列表中的每个字典,然后使用内层的for循环遍历字典中的每个键值对,并打印出来。这样我们就可以方便地访问列表中每个字典的内容。
二、使用枚举
使用枚举函数可以在遍历列表的同时获得索引值,这在需要处理列表的索引时非常有用。下面是一个例子:
list_of_dicts = [{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 30}, {'name': 'Charlie', 'age': 35}]
for index, dictionary in enumerate(list_of_dicts):
print(f"Dictionary {index}:")
for key, value in dictionary.items():
print(f" {key}: {value}")
在这个例子中,我们使用枚举函数遍历列表,并在每次迭代中同时获取列表的索引值和字典。这样我们不仅可以访问字典的内容,还可以知道当前字典在列表中的位置。
三、使用列表推导式
列表推导式是一种简洁的语法,用于创建新的列表。在遍历列表中的字典时,我们也可以使用列表推导式。下面是一个例子:
list_of_dicts = [{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 30}, {'name': 'Charlie', 'age': 35}]
new_list = [f"{key}: {value}" for dictionary in list_of_dicts for key, value in dictionary.items()]
print(new_list)
在这个例子中,我们使用嵌套的列表推导式遍历列表中的每个字典,并将字典中的每个键值对格式化为字符串,最终生成一个新的列表。这样我们就可以在不使用显式的for循环的情况下,简洁地处理列表中的字典。
四、结合以上方法
有时我们需要结合以上方法来实现更复杂的操作。比如,我们可以使用for循环遍历列表中的字典,同时使用枚举函数获取索引值,并使用列表推导式创建新的列表。下面是一个例子:
list_of_dicts = [{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 30}, {'name': 'Charlie', 'age': 35}]
for index, dictionary in enumerate(list_of_dicts):
print(f"Dictionary {index}:")
new_list = [f"{key}: {value}" for key, value in dictionary.items()]
print(new_list)
在这个例子中,我们使用for循环和枚举函数遍历列表中的每个字典,同时使用列表推导式生成新的列表,并打印出来。这样我们就可以在遍历列表的同时,灵活地处理字典中的内容。
五、处理嵌套字典
有时列表中的字典可能包含嵌套的字典。在这种情况下,我们需要使用递归函数来遍历嵌套的字典。下面是一个例子:
list_of_dicts = [{'name': 'Alice', 'info': {'age': 25, 'city': 'New York'}}, {'name': 'Bob', 'info': {'age': 30, 'city': 'Los Angeles'}}]
def traverse_dict(dictionary, indent=0):
for key, value in dictionary.items():
if isinstance(value, dict):
print(' ' * indent + f"{key}:")
traverse_dict(value, indent + 1)
else:
print(' ' * indent + f"{key}: {value}")
for dictionary in list_of_dicts:
traverse_dict(dictionary)
在这个例子中,我们定义了一个递归函数traverse_dict
,用于遍历嵌套的字典。在遍历列表中的每个字典时,我们调用这个递归函数来处理嵌套的字典。这样我们就可以方便地处理嵌套字典的遍历。
六、使用函数式编程
Python还支持函数式编程范式,我们可以使用map和lambda函数来处理列表中的字典。下面是一个例子:
list_of_dicts = [{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 30}, {'name': 'Charlie', 'age': 35}]
def process_dict(dictionary):
return {key: f"Processed {value}" for key, value in dictionary.items()}
new_list = list(map(process_dict, list_of_dicts))
print(new_list)
在这个例子中,我们定义了一个函数process_dict
,用于处理字典中的每个键值对。然后我们使用map函数将这个处理函数应用到列表中的每个字典,并生成一个新的列表。这样我们就可以使用函数式编程的方式,简洁地处理列表中的字典。
七、处理大数据集的性能优化
在处理大数据集时,遍历列表中的字典可能会导致性能问题。为了提高性能,我们可以使用生成器和迭代器来优化遍历过程。下面是一个例子:
list_of_dicts = [{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 30}, {'name': 'Charlie', 'age': 35}]
def generate_processed_dicts(list_of_dicts):
for dictionary in list_of_dicts:
yield {key: f"Processed {value}" for key, value in dictionary.items()}
processed_dicts = generate_processed_dicts(list_of_dicts)
for processed_dict in processed_dicts:
print(processed_dict)
在这个例子中,我们定义了一个生成器函数generate_processed_dicts
,用于生成处理后的字典。使用生成器可以在遍历过程中按需生成数据,避免一次性加载所有数据,从而提高性能。这样我们就可以在处理大数据集时,优化遍历列表中的字典的性能。
八、处理具有特定键的字典
有时我们只需要处理列表中具有特定键的字典。我们可以使用条件判断来筛选这些字典。下面是一个例子:
list_of_dicts = [{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 30}, {'name': 'Charlie', 'city': 'New York'}]
for dictionary in list_of_dicts:
if 'age' in dictionary:
print(f"Name: {dictionary['name']}, Age: {dictionary['age']}")
在这个例子中,我们遍历列表中的每个字典,并使用条件判断筛选出包含特定键(例如'age')的字典,然后处理这些字典。这样我们就可以有选择地处理列表中的字典。
九、处理具有复杂结构的字典
有时列表中的字典可能具有复杂的结构,例如包含列表、元组等。在这种情况下,我们需要使用递归函数来遍历和处理这些复杂结构的字典。下面是一个例子:
list_of_dicts = [{'name': 'Alice', 'info': {'age': 25, 'hobbies': ['reading', 'swimming']}}, {'name': 'Bob', 'info': {'age': 30, 'hobbies': ['hiking', 'cycling']}}]
def traverse_complex_dict(dictionary, indent=0):
for key, value in dictionary.items():
if isinstance(value, dict):
print(' ' * indent + f"{key}:")
traverse_complex_dict(value, indent + 1)
elif isinstance(value, list):
print(' ' * indent + f"{key}:")
for item in value:
print(' ' * (indent + 1) + f"- {item}")
else:
print(' ' * indent + f"{key}: {value}")
for dictionary in list_of_dicts:
traverse_complex_dict(dictionary)
在这个例子中,我们定义了一个递归函数traverse_complex_dict
,用于遍历和处理复杂结构的字典。在处理字典中的每个键值对时,我们使用条件判断来处理不同类型的值(例如字典、列表等),并根据需要进行递归调用。这样我们就可以方便地处理具有复杂结构的字典。
十、处理字典中的重复键
有时列表中的字典可能包含重复的键。在这种情况下,我们需要处理这些重复的键。下面是一个例子:
list_of_dicts = [{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 30}, {'name': 'Charlie', 'age': 35, 'name': 'Charlie'}]
for dictionary in list_of_dicts:
seen_keys = set()
for key, value in dictionary.items():
if key in seen_keys:
print(f"Duplicate key found: {key}")
else:
seen_keys.add(key)
print(f"{key}: {value}")
在这个例子中,我们遍历列表中的每个字典,并使用一个集合seen_keys
记录已处理的键。如果在遍历过程中遇到重复的键,我们可以对其进行处理(例如打印警告信息)。这样我们就可以在处理列表中的字典时,识别和处理重复的键。
通过以上方法,我们可以灵活地遍历和处理Python中列表中的字典。根据具体的需求和数据结构,选择合适的方法可以帮助我们高效地完成任务。希望这篇文章对你有所帮助!
相关问答FAQs:
如何在Python中遍历包含字典的列表?
遍历一个包含字典的列表通常可以使用for循环。你可以直接使用for语句迭代列表中的每个字典。示例代码如下:
list_of_dicts = [{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 30}]
for item in list_of_dicts:
print(item['name'], item['age'])
这样可以方便地访问每个字典中的键值。
使用列表推导式遍历字典列表有什么优势?
列表推导式使得代码更加简洁和易读。当你只需要从字典中提取某些特定的信息时,它非常有用。示例:
names = [item['name'] for item in list_of_dicts]
print(names)
这段代码将提取所有名字,并以列表的形式返回。
如何处理字典列表中缺失的键?
在遍历字典列表时,某些字典可能缺失某些键。可以使用dict.get()
方法来避免KeyError异常。示例:
for item in list_of_dicts:
name = item.get('name', 'Unknown') # 如果'name'不存在,返回'Unknown'
age = item.get('age', 'N/A') # 如果'age'不存在,返回'N/A'
print(name, age)
这种方法确保了代码的健壮性,避免因缺失键而导致程序崩溃。
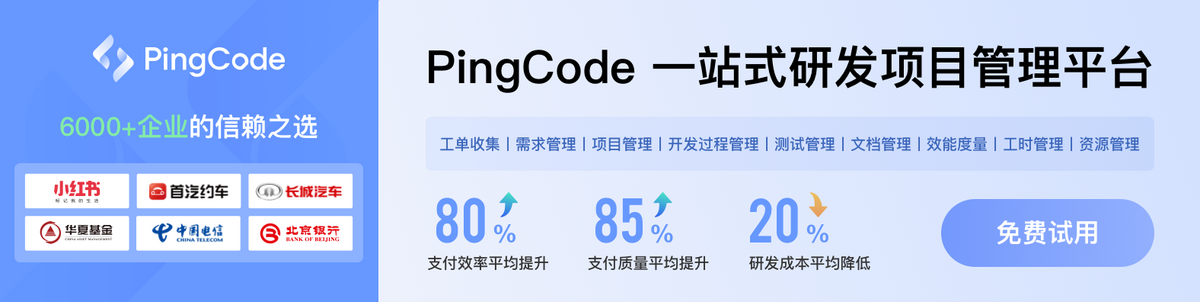