开头段落: Python中选中一个单词的方法包括使用正则表达式、字符串方法、NLP工具包。其中,最常见的方式是使用正则表达式(Regular Expressions,简称正则)。正则表达式是一种强大的工具,可以用来匹配复杂的字符串模式,提取特定的子字符串。在Python中,正则表达式通过re
模块来实现。我们可以通过定义一个正则表达式模式来匹配单词,然后使用re
模块中的函数进行搜索和提取。下面将详细介绍几种方法。
一、使用正则表达式
正则表达式是一种用于描述字符模式的工具。在Python中,可以使用re
模块来进行正则表达式的操作。正则表达式可以用来匹配单词、提取单词、替换单词等。
- 匹配单词
要在字符串中匹配单词,可以使用正则表达式模式r'\b\w+\b'
。其中,\b
表示单词边界,\w+
表示一个或多个字母、数字或下划线的组合。以下是一个例子:
import re
text = "This is a sample text with several words."
pattern = r'\b\w+\b'
matches = re.findall(pattern, text)
print(matches)
输出:
['This', 'is', 'a', 'sample', 'text', 'with', 'several', 'words']
在这个例子中,re.findall()
函数会返回一个包含所有匹配单词的列表。
- 提取单词
如果只想提取特定的单词,可以使用更具体的正则表达式模式。例如,要提取所有以字母"s"开头的单词,可以使用模式r'\bs\w*\b'
:
import re
text = "This is a sample text with several words."
pattern = r'\bs\w*\b'
matches = re.findall(pattern, text)
print(matches)
输出:
['sample', 'several']
在这个例子中,\bs\w*\b
模式表示以字母"s"开头的单词。
- 替换单词
使用正则表达式还可以进行单词替换。例如,要将所有单词"sample"替换为"example",可以使用以下代码:
import re
text = "This is a sample text with several sample words."
pattern = r'\bsample\b'
replacement = "example"
new_text = re.sub(pattern, replacement, text)
print(new_text)
输出:
This is a example text with several example words.
在这个例子中,re.sub()
函数用于替换所有匹配的单词。
二、使用字符串方法
除了正则表达式,Python的字符串方法也可以用来处理单词。这些方法包括split()
、replace()
等。
- 分割字符串
使用split()
方法可以将字符串分割成单词列表。例如:
text = "This is a sample text with several words."
words = text.split()
print(words)
输出:
['This', 'is', 'a', 'sample', 'text', 'with', 'several', 'words']
在这个例子中,split()
方法默认以空格为分隔符,将字符串分割成单词列表。
- 替换单词
使用replace()
方法可以替换字符串中的特定单词。例如:
text = "This is a sample text with several sample words."
new_text = text.replace("sample", "example")
print(new_text)
输出:
This is a example text with several example words.
在这个例子中,replace()
方法将所有"sample"替换为"example"。
三、使用NLP工具包
自然语言处理(NLP)工具包如NLTK、spaCy等也可以用来处理单词。这些工具包提供了强大的文本处理功能,包括分词、词性标注、命名实体识别等。
- 使用NLTK
NLTK(Natural Language Toolkit)是一个广泛使用的Python库,用于处理自然语言数据。以下是一个使用NLTK进行分词的例子:
import nltk
from nltk.tokenize import word_tokenize
text = "This is a sample text with several words."
nltk.download('punkt')
words = word_tokenize(text)
print(words)
输出:
['This', 'is', 'a', 'sample', 'text', 'with', 'several', 'words', '.']
在这个例子中,word_tokenize()
函数用于将字符串分割成单词列表。
- 使用spaCy
spaCy是另一个流行的NLP库,提供了高效的文本处理功能。以下是一个使用spaCy进行分词的例子:
import spacy
nlp = spacy.load("en_core_web_sm")
text = "This is a sample text with several words."
doc = nlp(text)
words = [token.text for token in doc]
print(words)
输出:
['This', 'is', 'a', 'sample', 'text', 'with', 'several', 'words', '.']
在这个例子中,spaCy的分词功能可以高效地将字符串分割成单词列表。
四、使用Pandas
Pandas是一个强大的数据处理库,通常用于数据分析和操作。虽然Pandas主要用于处理结构化数据,但它也可以用来处理文本数据。以下是一个使用Pandas处理文本数据的例子:
- 分割字符串
import pandas as pd
data = {'text': ["This is a sample text with several words."]}
df = pd.DataFrame(data)
df['words'] = df['text'].str.split()
print(df)
输出:
text words
0 This is a sample text with several words. [This, is, a, sample, text, with, several, words.]
在这个例子中,Pandas的str.split()
方法用于将字符串分割成单词列表。
- 替换单词
import pandas as pd
data = {'text': ["This is a sample text with several sample words."]}
df = pd.DataFrame(data)
df['new_text'] = df['text'].str.replace("sample", "example")
print(df)
输出:
text new_text
0 This is a sample text with several sample words. This is a example text with several example words.
在这个例子中,Pandas的str.replace()
方法用于替换字符串中的特定单词。
五、使用TextBlob
TextBlob是一个简单易用的Python库,用于处理文本数据。它提供了分词、词性标注、情感分析等功能。以下是一个使用TextBlob进行分词的例子:
- 分割字符串
from textblob import TextBlob
text = "This is a sample text with several words."
blob = TextBlob(text)
words = blob.words
print(words)
输出:
['This', 'is', 'a', 'sample', 'text', 'with', 'several', 'words']
在这个例子中,TextBlob的words
属性用于将字符串分割成单词列表。
- 替换单词
TextBlob没有直接的替换单词功能,但可以通过字符串方法实现替换。例如:
from textblob import TextBlob
text = "This is a sample text with several sample words."
blob = TextBlob(text)
new_text = text.replace("sample", "example")
print(new_text)
输出:
This is a example text with several example words.
在这个例子中,使用字符串的replace()
方法替换单词。
总结
在Python中,有多种方法可以选中一个单词,包括使用正则表达式、字符串方法、NLP工具包、Pandas、TextBlob等。每种方法都有其优点和适用场景,可以根据具体需求选择合适的方法。正则表达式适用于复杂的字符串模式匹配,字符串方法简单易用,NLP工具包提供了强大的文本处理功能,Pandas适用于处理结构化数据,TextBlob则是一个简单易用的文本处理库。希望这些方法能帮助你在Python中高效地选中和处理单词。
相关问答FAQs:
在Python中,如何使用正则表达式选中一个单词?
正则表达式是Python中处理字符串的强大工具。您可以使用re
模块来匹配单词。例如,如果您想选中字符串中的“apple”这个单词,可以使用以下代码:
import re
text = "I like to eat an apple."
match = re.search(r'\bapple\b', text)
if match:
print("选中的单词:", match.group())
这里的\b
表示单词边界,确保只匹配完整的单词。
如何在Python中对字符串进行分词以选中单个单词?
使用Python的split()
方法可以将字符串分割成单词列表。以下示例展示了如何选中列表中的特定单词:
text = "I like to eat an apple."
words = text.split()
selected_word = "apple" if "apple" in words else "未找到该单词"
print("选中的单词:", selected_word)
这种方法适用于简单的分词需求。
在Python中,有哪些方法可以区分大小写地选中单词?
如果需要区分大小写来选中单词,可以在使用字符串的find()
方法时设置参数,或者在使用正则表达式时不加入re.IGNORECASE
标志。以下是一个示例:
text = "I like to eat an Apple."
if "Apple" in text:
print("选中的单词: Apple")
else:
print("未找到该单词")
这样可以确保只有完全匹配大小写的单词会被选中。
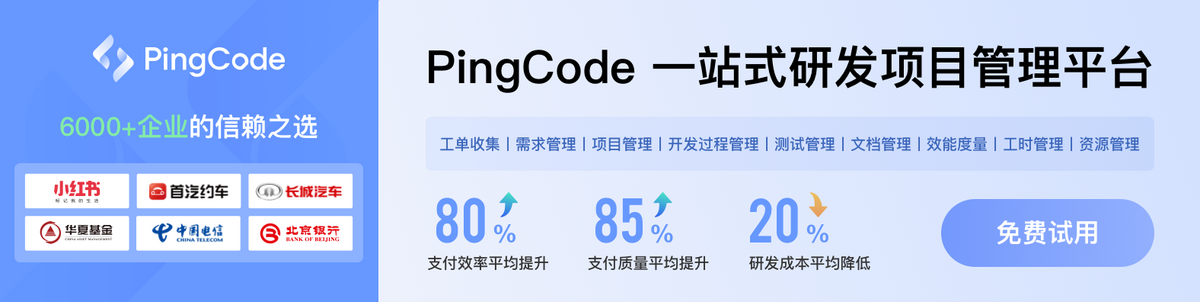