使用Python创建窗口文件的方法包括安装所需的库、编写基本的窗口代码、添加组件、处理事件等。下面将详细介绍其中一个方法,即使用Tkinter库创建窗口文件。
安装Tkinter库
Tkinter 是Python的标准GUI(图形用户界面)库,不需要单独安装。只需确保你已经安装了Python。
一、导入Tkinter库
在你的Python脚本中,首先需要导入Tkinter库。
import tkinter as tk
from tkinter import ttk
二、创建主窗口
接下来,需要创建主窗口。主窗口是所有其他组件的父窗口。
root = tk.Tk()
root.title("My Tkinter Window")
root.geometry("800x600")
三、添加组件
在主窗口中添加各种组件,例如标签、按钮、输入框等。
# 创建标签
label = tk.Label(root, text="Hello, Tkinter!")
label.pack(pady=20)
创建按钮
button = tk.Button(root, text="Click Me!", command=lambda: print("Button Clicked!"))
button.pack(pady=20)
创建输入框
entry = tk.Entry(root)
entry.pack(pady=20)
四、处理事件
事件处理是指在用户与GUI组件交互时执行某些操作。例如,按钮点击事件。
def on_button_click():
print("Button was clicked!")
button = tk.Button(root, text="Click Me!", command=on_button_click)
button.pack(pady=20)
五、主事件循环
主事件循环是GUI应用程序的核心。它等待用户与应用程序交互,并处理这些事件。
root.mainloop()
完整代码示例:
import tkinter as tk
from tkinter import ttk
def on_button_click():
print("Button was clicked!")
创建主窗口
root = tk.Tk()
root.title("My Tkinter Window")
root.geometry("800x600")
创建标签
label = tk.Label(root, text="Hello, Tkinter!")
label.pack(pady=20)
创建按钮
button = tk.Button(root, text="Click Me!", command=on_button_click)
button.pack(pady=20)
创建输入框
entry = tk.Entry(root)
entry.pack(pady=20)
运行主事件循环
root.mainloop()
六、进一步扩展
以上代码展示了如何创建一个简单的窗口应用程序。你可以根据需要进一步扩展,例如添加更多组件、定义更多事件处理函数、使用布局管理器等。
使用布局管理器
Tkinter 提供了三种布局管理器:pack、grid 和 place。你可以根据需要选择合适的布局管理器来组织组件。
pack布局:
pack布局管理器根据组件的顺序和方向(上下、左右)排列组件。
label1 = tk.Label(root, text="Label 1")
label1.pack(side="top", fill="x")
label2 = tk.Label(root, text="Label 2")
label2.pack(side="top", fill="x")
grid布局:
grid布局管理器允许你使用网格来组织组件。每个组件放置在特定的行和列中。
label1 = tk.Label(root, text="Label 1")
label1.grid(row=0, column=0)
label2 = tk.Label(root, text="Label 2")
label2.grid(row=1, column=0)
place布局:
place布局管理器允许你使用绝对位置来放置组件。
label1 = tk.Label(root, text="Label 1")
label1.place(x=50, y=50)
label2 = tk.Label(root, text="Label 2")
label2.place(x=150, y=100)
处理更多事件
除了按钮点击事件,你还可以处理其他事件,例如键盘输入、鼠标点击等。
键盘输入事件:
def on_key_press(event):
print(f"Key pressed: {event.keysym}")
root.bind("<KeyPress>", on_key_press)
鼠标点击事件:
def on_mouse_click(event):
print(f"Mouse clicked at: ({event.x}, {event.y})")
root.bind("<Button-1>", on_mouse_click)
使用ttk模块
ttk模块提供了Tkinter的主题小部件,可以让你的应用程序看起来更加现代。
# 创建ttk按钮
ttk_button = ttk.Button(root, text="TTK Button", command=on_button_click)
ttk_button.pack(pady=20)
创建菜单
你可以在Tkinter应用程序中创建菜单,为用户提供更多的操作选项。
# 创建菜单
menu_bar = tk.Menu(root)
创建文件菜单
file_menu = tk.Menu(menu_bar, tearoff=0)
file_menu.add_command(label="Open")
file_menu.add_command(label="Save")
file_menu.add_separator()
file_menu.add_command(label="Exit", command=root.quit)
menu_bar.add_cascade(label="File", menu=file_menu)
添加菜单到主窗口
root.config(menu=menu_bar)
总结
通过以上步骤,你已经了解了如何使用Python和Tkinter库创建一个简单的窗口应用程序。你可以根据需要进一步扩展和自定义你的应用程序,例如添加更多组件、处理更多事件、使用布局管理器、创建菜单等。希望这篇文章对你有所帮助,祝你在Python编程中取得更多进展!
相关问答FAQs:
如何使用Python创建一个简单的GUI窗口?
可以使用Python的Tkinter库来创建一个简单的GUI窗口。Tkinter是Python的标准GUI库,提供了创建窗口和各种控件的功能。只需导入Tkinter,创建一个Tk对象,然后调用mainloop()方法即可显示窗口。示例代码如下:
import tkinter as tk
root = tk.Tk()
root.title("我的窗口")
root.geometry("300x200")
root.mainloop()
在Python窗口中如何添加按钮和文本框?
可以通过Tkinter库中的Button和Entry控件来实现按钮和文本框的添加。使用Button控件可以创建按钮,使用Entry控件可以创建文本框。通过定义相应的回调函数,可以使按钮执行特定的操作,例如获取文本框中的内容。示例代码如下:
import tkinter as tk
def on_button_click():
print(entry.get())
root = tk.Tk()
root.title("我的窗口")
root.geometry("300x200")
entry = tk.Entry(root)
entry.pack(pady=10)
button = tk.Button(root, text="点击我", command=on_button_click)
button.pack(pady=10)
root.mainloop()
如何定制窗口的外观和布局?
可以通过Tkinter提供的各种属性和布局管理器来定制窗口的外观和布局。例如,可以使用pack、grid或place方法来安排控件的位置,使用configure方法来设置控件的颜色、字体和大小等属性。借助这些工具,可以创建出更符合用户需求的界面设计。使用示例:
import tkinter as tk
root = tk.Tk()
root.title("定制窗口")
root.geometry("400x300")
label = tk.Label(root, text="欢迎使用我的程序", font=("Arial", 14))
label.pack(pady=20)
button = tk.Button(root, text="关闭", command=root.quit, bg="red", fg="white")
button.pack(pady=10)
root.mainloop()
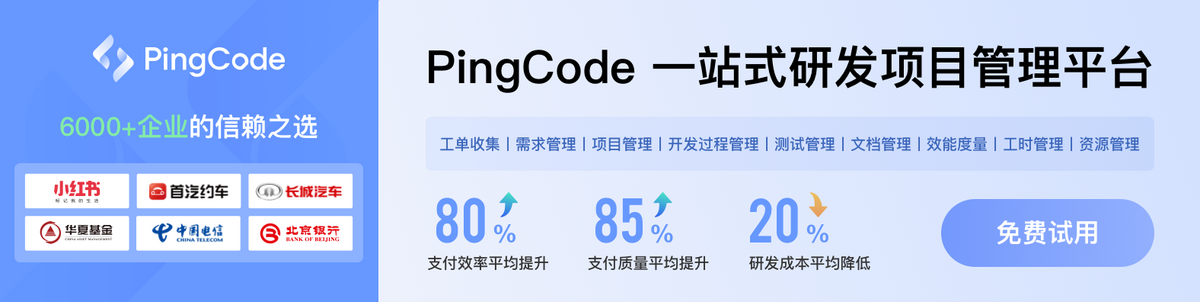