在Python中,可以通过使用Pillow库来将PNG格式的图像转换为JPG格式。 Pillow是Python Imaging Library (PIL) 的一个分支,提供了广泛的图像处理功能。可以通过安装Pillow库,并使用其提供的功能来轻松实现PNG到JPG的转换。具体步骤包括安装Pillow库、读取PNG文件、转换图像模式并保存为JPG格式。下面将详细描述这些步骤。
一、安装Pillow库
要在Python中处理图像,首先需要安装Pillow库。可以使用以下命令通过pip安装Pillow:
pip install pillow
这将安装最新版本的Pillow库,使其可用于图像处理任务。
二、读取PNG文件
安装Pillow库后,可以使用它来读取PNG文件。下面是如何使用Pillow读取PNG文件的示例代码:
from PIL import Image
打开PNG文件
png_image = Image.open('example.png')
在这段代码中,Image.open
函数用于打开指定路径的PNG文件,并将其加载为Pillow的Image对象。
三、转换图像模式
PNG图像通常支持透明度(即Alpha通道),而JPG格式不支持透明度。因此,在将PNG图像转换为JPG格式之前,需要将图像模式从RGBA(如果存在Alpha通道)转换为RGB。可以使用convert
方法来完成这一操作:
# 将图像模式从RGBA转换为RGB
rgb_image = png_image.convert('RGB')
在这段代码中,convert
方法用于将图像模式从RGBA转换为RGB,以确保生成的JPG图像没有Alpha通道。
四、保存为JPG格式
完成图像模式转换后,可以使用save
方法将图像保存为JPG格式。可以指定输出文件的路径和格式:
# 保存为JPG格式
rgb_image.save('example.jpg', 'JPEG')
在这段代码中,save
方法用于将图像保存为指定路径的JPG文件,并指定图像格式为JPEG。
五、完整示例代码
以下是将上述所有步骤整合在一起的完整示例代码:
from PIL import Image
打开PNG文件
png_image = Image.open('example.png')
将图像模式从RGBA转换为RGB
rgb_image = png_image.convert('RGB')
保存为JPG格式
rgb_image.save('example.jpg', 'JPEG')
通过运行这段代码,可以将名为example.png
的PNG文件转换为名为example.jpg
的JPG文件。
六、处理批量文件转换
如果需要处理多个PNG文件,可以使用循环遍历文件列表,并使用上述步骤逐个转换。以下是一个示例代码,用于批量转换指定目录下的所有PNG文件:
import os
from PIL import Image
def convert_png_to_jpg(directory):
for filename in os.listdir(directory):
if filename.endswith('.png'):
png_image = Image.open(os.path.join(directory, filename))
rgb_image = png_image.convert('RGB')
jpg_filename = os.path.splitext(filename)[0] + '.jpg'
rgb_image.save(os.path.join(directory, jpg_filename), 'JPEG')
print(f'Converted {filename} to {jpg_filename}')
指定目录路径
directory_path = 'path_to_directory'
convert_png_to_jpg(directory_path)
在这段代码中,os.listdir
函数用于列出指定目录中的所有文件,os.path.splitext
函数用于获取文件名的基本部分(不包括扩展名),并将其替换为.jpg
扩展名。convert_png_to_jpg
函数遍历目录中的所有PNG文件,并逐个进行转换。
七、处理错误和异常
在实际应用中,处理文件时可能会遇到各种错误和异常,例如文件不存在、文件格式不正确等。可以使用try-except块来捕获和处理这些异常:
import os
from PIL import Image
def convert_png_to_jpg_with_error_handling(directory):
for filename in os.listdir(directory):
if filename.endswith('.png'):
try:
png_image = Image.open(os.path.join(directory, filename))
rgb_image = png_image.convert('RGB')
jpg_filename = os.path.splitext(filename)[0] + '.jpg'
rgb_image.save(os.path.join(directory, jpg_filename), 'JPEG')
print(f'Converted {filename} to {jpg_filename}')
except Exception as e:
print(f'Failed to convert {filename}: {e}')
指定目录路径
directory_path = 'path_to_directory'
convert_png_to_jpg_with_error_handling(directory_path)
在这段代码中,try-except块用于捕获并处理在图像转换过程中可能发生的任何异常,并打印错误消息。
八、提高图像转换效率
在处理大量图像时,可以通过多线程或多进程来提高转换效率。以下是使用ThreadPoolExecutor进行并行转换的示例代码:
import os
from PIL import Image
from concurrent.futures import ThreadPoolExecutor
def convert_single_png_to_jpg(filename, directory):
try:
png_image = Image.open(os.path.join(directory, filename))
rgb_image = png_image.convert('RGB')
jpg_filename = os.path.splitext(filename)[0] + '.jpg'
rgb_image.save(os.path.join(directory, jpg_filename), 'JPEG')
print(f'Converted {filename} to {jpg_filename}')
except Exception as e:
print(f'Failed to convert {filename}: {e}')
def convert_png_to_jpg_parallel(directory):
with ThreadPoolExecutor() as executor:
png_files = [f for f in os.listdir(directory) if f.endswith('.png')]
executor.map(lambda f: convert_single_png_to_jpg(f, directory), png_files)
指定目录路径
directory_path = 'path_to_directory'
convert_png_to_jpg_parallel(directory_path)
在这段代码中,ThreadPoolExecutor
用于创建一个线程池,并通过executor.map
方法将转换任务分配给多个线程并行执行。这可以显著提高处理大量图像的效率。
通过上述步骤,可以轻松地在Python中将PNG格式的图像转换为JPG格式。希望这些详细的介绍和示例代码能够帮助你在实际应用中顺利实现图像格式的转换。
相关问答FAQs:
如何在Python中使用PIL库将PNG转换为JPG格式?
使用PIL(Pillow)库可以轻松将PNG文件转换为JPG格式。首先,确保已安装Pillow库。可以使用以下命令安装:
pip install Pillow
接下来,使用以下代码示例进行转换:
from PIL import Image
# 打开PNG文件
png_image = Image.open('image.png')
# 转换为RGB模式(JPG不支持透明度)
rgb_image = png_image.convert('RGB')
# 保存为JPG格式
rgb_image.save('image.jpg', 'JPEG')
该代码会将名为image.png
的文件转换为image.jpg
,并保留其颜色信息。
转换PNG为JPG时会影响图像质量吗?
在将PNG转换为JPG的过程中,确实可能会影响图像质量。PNG格式支持无损压缩和透明度,而JPG格式则是有损压缩并不支持透明度。为了尽量减少质量损失,在保存JPG文件时,可以调整质量参数,例如:
rgb_image.save('image.jpg', 'JPEG', quality=95)
这将生成较高质量的JPG文件,但文件大小也会相应增加。
如何批量将多个PNG文件转换为JPG格式?
如果需要批量转换多个PNG文件,可以使用循环来处理文件。以下是一个简单的示例:
import os
from PIL import Image
# 指定PNG文件目录
png_directory = 'path/to/png/files'
# 创建输出目录
output_directory = 'path/to/jpg/files'
os.makedirs(output_directory, exist_ok=True)
# 遍历目录中的所有PNG文件
for filename in os.listdir(png_directory):
if filename.endswith('.png'):
png_image = Image.open(os.path.join(png_directory, filename))
rgb_image = png_image.convert('RGB')
# 保存为JPG文件
jpg_filename = f"{os.path.splitext(filename)[0]}.jpg"
rgb_image.save(os.path.join(output_directory, jpg_filename), 'JPEG')
这段代码会将指定目录中所有的PNG文件转换为JPG格式,并保存在新的输出目录中。
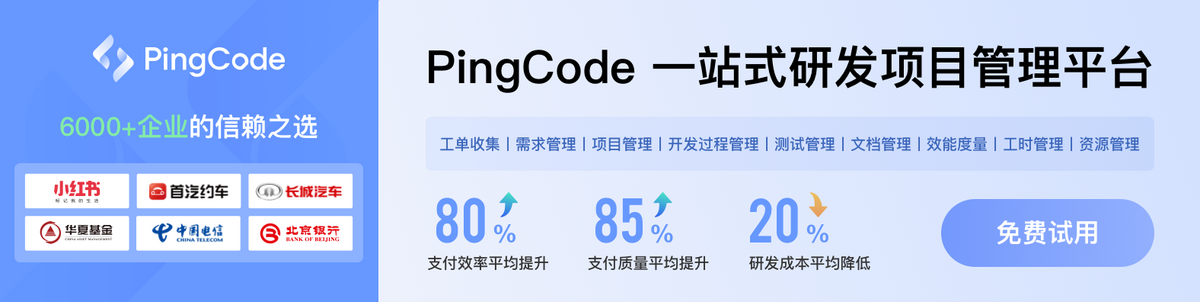