要在Python中创建动态可视化图,可以使用多个库,如matplotlib、Plotly、Bokeh等。本文将详细介绍如何使用这些库来实现动态可视化图。
一、MATPLOTLIB
Matplotlib是Python中最著名的绘图库之一,可以用来生成静态、动态和交互式的图表。为了创建动态可视化图,通常会使用matplotlib.animation模块。
1.1 安装Matplotlib
pip install matplotlib
1.2 基本示例
下面是一个使用Matplotlib创建简单动态图的示例:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
fig, ax = plt.subplots()
x = np.arange(0, 2*np.pi, 0.01)
line, = ax.plot(x, np.sin(x))
def update(frame):
line.set_ydata(np.sin(x + frame / 10.0))
return line,
ani = animation.FuncAnimation(fig, update, frames=100, blit=True)
plt.show()
在这段代码中,animation.FuncAnimation
函数用于创建动画,update
函数用于更新每一帧的数据。
1.3 高级示例
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
fig, ax = plt.subplots()
x = np.arange(0, 2 * np.pi, 0.01)
line, = ax.plot(x, np.sin(x))
def init():
ax.set_xlim(0, 2 * np.pi)
ax.set_ylim(-1.5, 1.5)
return line,
def update(frame):
line.set_ydata(np.sin(x + frame / 10.0))
return line,
ani = animation.FuncAnimation(fig, update, frames=100, init_func=init, blit=True)
plt.show()
在这个高级示例中,init
函数用于初始化图表,这样每次更新时图表的轴不会重新绘制。
二、PLOTLY
Plotly是一个强大的绘图库,可以生成交互式图表,适合于Web应用。使用Plotly创建动态图表可以使用户与图表进行更多的交互。
2.1 安装Plotly
pip install plotly
2.2 基本示例
下面是一个使用Plotly创建简单动态图的示例:
import plotly.graph_objs as go
import plotly.express as px
import numpy as np
x = np.linspace(0, 2*np.pi, 100)
y = np.sin(x)
fig = px.line(x=x, y=y)
fig.show()
2.3 动态更新示例
import plotly.graph_objs as go
from plotly.subplots import make_subplots
import numpy as np
import pandas as pd
x = np.linspace(0, 2*np.pi, 100)
frames = [go.Frame(data=[go.Scatter(x=x, y=np.sin(x + i / 10.0))]) for i in range(100)]
fig = make_subplots(rows=1, cols=1)
fig.add_trace(go.Scatter(x=x, y=np.sin(x)))
fig.frames = frames
fig.update_layout(updatemenus=[{
'buttons': [
{
'args': [None, {'frame': {'duration': 50, 'redraw': True}, 'fromcurrent': True}],
'label': 'Play',
'method': 'animate'
}
]
}])
fig.show()
2.4 高级示例
import plotly.graph_objs as go
from plotly.subplots import make_subplots
import numpy as np
x = np.linspace(0, 2*np.pi, 100)
frames = [go.Frame(data=[go.Scatter(x=x, y=np.sin(x + i / 10.0))]) for i in range(100)]
fig = make_subplots(rows=1, cols=1)
fig.add_trace(go.Scatter(x=x, y=np.sin(x)))
fig.frames = frames
fig.update_layout(
updatemenus=[{
'buttons': [
{
'args': [None, {'frame': {'duration': 50, 'redraw': True}, 'fromcurrent': True}],
'label': 'Play',
'method': 'animate'
}
]
}],
sliders=[{
'steps': [
{
'args': [
[i],
{
'frame': {'duration': 50, 'redraw': True},
'mode': 'immediate',
'transition': {'duration': 0}
}
],
'label': str(i),
'method': 'animate'
}
for i in range(100)
]
}]
)
fig.show()
在这个高级示例中,添加了一个滑动条,使得用户可以手动控制动画的进度。
三、BOKEH
Bokeh是一个交互式可视化库,可以生成高质量的图表,并且支持Web应用。
3.1 安装Bokeh
pip install bokeh
3.2 基本示例
下面是一个使用Bokeh创建简单动态图的示例:
from bokeh.plotting import figure, show
from bokeh.models import ColumnDataSource
from bokeh.io import curdoc
from bokeh.layouts import column
from bokeh.driving import linear
source = ColumnDataSource(data=dict(x=[], y=[]))
p = figure()
p.line('x', 'y', source=source)
@linear()
def update(step):
new_data = dict(x=[step], y=[step % 10])
source.stream(new_data, rollover=200)
curdoc().add_periodic_callback(update, 100)
show(p)
3.3 高级示例
from bokeh.plotting import figure, show
from bokeh.models import ColumnDataSource
from bokeh.io import curdoc
from bokeh.layouts import column
from bokeh.driving import linear
import numpy as np
source = ColumnDataSource(data=dict(x=[], y=[]))
p = figure()
p.line('x', 'y', source=source)
@linear()
def update(step):
new_data = dict(x=[step], y=[np.sin(step / 10.0)])
source.stream(new_data, rollover=200)
curdoc().add_periodic_callback(update, 100)
show(p)
在这个高级示例中,生成的动态图表会显示一个正弦波随时间变化的动态过程。
四、总结
通过本文的介绍,您应该已经了解了如何使用Matplotlib、Plotly和Bokeh来创建动态可视化图。每个库都有其独特的优势和应用场景:
- Matplotlib:适合生成静态图表和简单的动画,适用于科学研究和数据分析。
- Plotly:适合生成交互式图表,适用于Web应用和数据展示。
- Bokeh:适合生成高质量的交互式图表,适用于Web应用和实时数据展示。
无论您选择哪个库,都可以根据具体需求和场景来创建符合要求的动态可视化图。希望本文对您有所帮助。
相关问答FAQs:
动态可视化图在Python中有哪些常用库?
在Python中,创建动态可视化图的常用库包括Matplotlib、Plotly和Bokeh。Matplotlib提供了基础的动画支持,适合简单的动态图表。Plotly则支持交互式和实时更新的图表,适合用于网页和数据展示。Bokeh专注于大规模数据集的交互式可视化,适合构建复杂的动态应用。
如何使用Matplotlib创建简单的动态图?
要使用Matplotlib创建动态图,可以使用FuncAnimation
模块。首先,您需要定义一个更新函数,该函数会在每一帧中更新数据。接着,通过FuncAnimation
将此函数与图表结合起来,指定更新的间隔时间和总帧数。例如,可以创建一个简单的正弦波动画,通过不断更新其数据点来实现动态效果。
在Python中动态可视化图表的性能如何优化?
为了优化动态可视化图表的性能,可以考虑以下几点:减少每帧更新的数据量,例如只更新必要的数据点,使用更高效的绘图库如Plotly或Bokeh,或者利用多线程技术来分担数据处理的负担。同时,选择合适的图表类型和渲染方式也能显著提升性能,例如使用WebGL进行图形渲染。
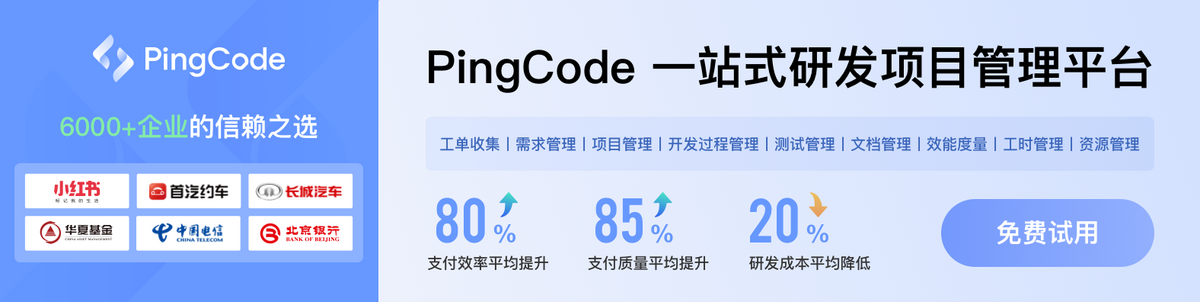