用Python快速遍历所有URL
在Python中,快速遍历所有URL可以通过多种方式实现,常见的方法包括使用requests库、BeautifulSoup库、多线程或异步编程。其中,多线程或异步编程可以显著提高遍历速度。下面详细介绍一种结合requests库和多线程的方法。
一、使用requests库和BeautifulSoup库
使用requests库和BeautifulSoup库可以方便地请求URL和解析网页内容。首先,你需要安装这两个库:
pip install requests
pip install beautifulsoup4
然后,可以通过以下代码示例来遍历URL并解析网页内容:
import requests
from bs4 import BeautifulSoup
def fetch_url(url):
response = requests.get(url)
return response.text
def parse_content(html):
soup = BeautifulSoup(html, 'html.parser')
return soup
def main():
urls = ['https://example.com', 'https://example.org'] # 要遍历的URL列表
for url in urls:
html = fetch_url(url)
soup = parse_content(html)
print(soup.title.string) # 示例:打印页面标题
if __name__ == "__main__":
main()
二、使用多线程提高遍历速度
为了提高遍历速度,可以使用多线程。Python的concurrent.futures
模块提供了高层次的接口来并行执行任务。
import requests
from bs4 import BeautifulSoup
from concurrent.futures import ThreadPoolExecutor
def fetch_url(url):
response = requests.get(url)
return response.text
def parse_content(html):
soup = BeautifulSoup(html, 'html.parser')
return soup
def process_url(url):
html = fetch_url(url)
soup = parse_content(html)
print(soup.title.string) # 示例:打印页面标题
def main():
urls = ['https://example.com', 'https://example.org'] # 要遍历的URL列表
with ThreadPoolExecutor(max_workers=10) as executor:
executor.map(process_url, urls)
if __name__ == "__main__":
main()
三、使用异步编程
异步编程可以进一步提升性能,尤其是在处理大量IO操作时。可以使用aiohttp
库和asyncio
库来实现异步遍历。
首先,安装aiohttp库:
pip install aiohttp
然后,使用以下代码示例:
import aiohttp
import asyncio
from bs4 import BeautifulSoup
async def fetch_url(session, url):
async with session.get(url) as response:
return await response.text()
async def parse_content(html):
soup = BeautifulSoup(html, 'html.parser')
return soup
async def process_url(session, url):
html = await fetch_url(session, url)
soup = await parse_content(html)
print(soup.title.string) # 示例:打印页面标题
async def main():
urls = ['https://example.com', 'https://example.org'] # 要遍历的URL列表
async with aiohttp.ClientSession() as session:
tasks = [process_url(session, url) for url in urls]
await asyncio.gather(*tasks)
if __name__ == "__main__":
asyncio.run(main())
四、总结
通过上述方法,可以快速遍历所有URL并解析网页内容。使用requests库和BeautifulSoup库可以方便地请求和解析网页内容,而通过多线程或异步编程可以显著提高遍历速度。选择适合自己需求的方法,实现高效的URL遍历。
在实际应用中,还可以根据需求进一步优化代码,例如增加异常处理、限制并发请求数、设置请求超时时间等,以提高程序的健壮性和稳定性。
相关问答FAQs:
如何使用Python遍历大量URL的最佳方法是什么?
在Python中,可以使用多线程或异步IO来快速遍历大量URL。使用requests
库结合concurrent.futures
模块的ThreadPoolExecutor
可以高效地发起并发请求。此外,aiohttp
库也提供了异步请求的功能,可以更有效地处理IO密集型操作。选择合适的方法可以显著提高遍历速度。
在遍历URL时,如何处理可能出现的错误或异常?
在遍历URL时,网络请求可能会因为多种原因失败,比如连接超时、404错误等。可以使用try-except
语句来捕获这些异常,并在出现错误时记录日志或重试请求。设定合理的重试次数和间隔时间,可以提高成功率,同时避免对目标服务器造成过大压力。
遍历URL后,如何高效地处理和存储获取到的数据?
在获取到数据后,可以使用Python的pandas
库将数据存储到DataFrame中,方便后续的数据处理和分析。也可以将数据输出到CSV或JSON文件中,以便于后续使用。此外,使用数据库(如SQLite或MongoDB)存储数据也是一个不错的选择,这样可以更方便地进行查询和管理。
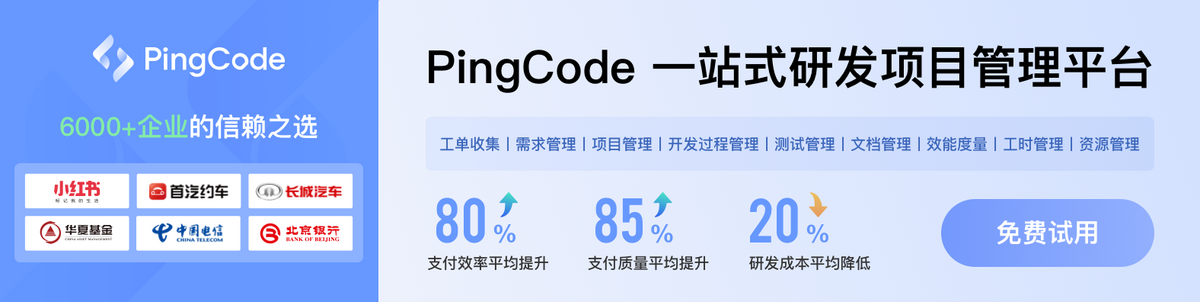