Python实现从FTP上下载文件的方法包括:使用ftplib库、使用urllib库、设置FTP连接、下载文件等。本文将详细探讨如何实现这些步骤,确保读者能够轻松掌握这一技巧。
一、使用ftplib库连接FTP服务器
ftplib是Python标准库中的一个模块,用于实现FTP协议。使用ftplib库,可以轻松地连接到FTP服务器并进行文件传输。以下是一个基本示例,展示了如何使用ftplib库连接到FTP服务器:
from ftplib import FTP
ftp = FTP('ftp.example.com') # 连接到FTP服务器
ftp.login(user='username', passwd='password') # 登录
二、浏览FTP目录
在连接到FTP服务器后,我们可以浏览FTP服务器上的目录。可以使用ftp.cwd()
方法更改当前目录,使用ftp.nlst()
方法列出目录中的文件和文件夹。以下是一个示例:
ftp.cwd('/path/to/directory') # 更改目录
files = ftp.nlst() # 列出目录中的文件和文件夹
print(files)
三、下载文件
使用ftplib库下载文件非常简单。我们可以使用ftp.retrbinary()
方法下载二进制文件,或者使用ftp.retrlines()
方法下载文本文件。以下是一个示例,展示了如何下载二进制文件:
local_filename = 'local_file.txt'
with open(local_filename, 'wb') as local_file:
ftp.retrbinary('RETR remote_file.txt', local_file.write)
四、处理FTP错误
在实际应用中,我们可能会遇到各种错误,例如连接超时、登录失败、文件不存在等。为了提高代码的健壮性,我们可以使用try-except语句来捕获和处理这些错误。以下是一个示例:
from ftplib import FTP, error_perm
try:
ftp = FTP('ftp.example.com')
ftp.login(user='username', passwd='password')
ftp.cwd('/path/to/directory')
files = ftp.nlst()
print(files)
local_filename = 'local_file.txt'
with open(local_filename, 'wb') as local_file:
ftp.retrbinary('RETR remote_file.txt', local_file.write)
except error_perm as e:
print(f'FTP error: {e}')
except Exception as e:
print(f'Error: {e}')
finally:
ftp.quit()
五、使用urllib库下载文件
除了ftplib库,Python还提供了另一个库urllib,用于处理URL,包括FTP。我们可以使用urllib库下载文件,以下是一个示例:
import urllib.request
url = 'ftp://username:password@ftp.example.com/path/to/remote_file.txt'
local_filename = 'local_file.txt'
urllib.request.urlretrieve(url, local_filename)
六、实现自动化下载
在实际应用中,我们可能需要自动化下载多个文件。可以使用循环来遍历文件列表,并依次下载每个文件。以下是一个示例,展示了如何实现自动化下载:
from ftplib import FTP
ftp = FTP('ftp.example.com')
ftp.login(user='username', passwd='password')
ftp.cwd('/path/to/directory')
files = ftp.nlst()
for file in files:
local_filename = file
with open(local_filename, 'wb') as local_file:
ftp.retrbinary(f'RETR {file}', local_file.write)
ftp.quit()
七、处理大文件下载
下载大文件时,我们可以使用分块下载的方式,以减少内存占用。以下是一个示例,展示了如何分块下载大文件:
chunk_size = 8192 # 定义块大小
def download_file(ftp, remote_file, local_file):
with open(local_file, 'wb') as local_file:
def callback(data):
local_file.write(data)
ftp.retrbinary(f'RETR {remote_file}', callback, blocksize=chunk_size)
ftp = FTP('ftp.example.com')
ftp.login(user='username', passwd='password')
ftp.cwd('/path/to/directory')
remote_file = 'remote_large_file.txt'
local_file = 'local_large_file.txt'
download_file(ftp, remote_file, local_file)
ftp.quit()
八、使用多线程下载文件
在下载大量文件时,我们可以使用多线程来提高下载速度。以下是一个示例,展示了如何使用多线程下载文件:
from ftplib import FTP
import threading
def download_file(ftp, remote_file, local_file):
with open(local_file, 'wb') as local_file:
ftp.retrbinary(f'RETR {remote_file}', local_file.write)
def ftp_thread(remote_file):
ftp = FTP('ftp.example.com')
ftp.login(user='username', passwd='password')
ftp.cwd('/path/to/directory')
local_file = remote_file
download_file(ftp, remote_file, local_file)
ftp.quit()
ftp = FTP('ftp.example.com')
ftp.login(user='username', passwd='password')
ftp.cwd('/path/to/directory')
files = ftp.nlst()
ftp.quit()
threads = []
for file in files:
thread = threading.Thread(target=ftp_thread, args=(file,))
thread.start()
threads.append(thread)
for thread in threads:
thread.join()
九、处理文件断点续传
在下载大文件时,如果连接中断,我们可以使用断点续传的方式继续下载。以下是一个示例,展示了如何实现断点续传:
import os
from ftplib import FTP
def download_file(ftp, remote_file, local_file):
if os.path.exists(local_file):
local_size = os.path.getsize(local_file)
ftp.sendcmd(f'REST {local_size}')
with open(local_file, 'ab') as local_file:
ftp.retrbinary(f'RETR {remote_file}', local_file.write, rest=local_size)
else:
with open(local_file, 'wb') as local_file:
ftp.retrbinary(f'RETR {remote_file}', local_file.write)
ftp = FTP('ftp.example.com')
ftp.login(user='username', passwd='password')
ftp.cwd('/path/to/directory')
remote_file = 'remote_large_file.txt'
local_file = 'local_large_file.txt'
download_file(ftp, remote_file, local_file)
ftp.quit()
十、总结
通过本文的介绍,我们学习了如何使用Python从FTP上下载文件。主要涉及的内容包括使用ftplib库、使用urllib库、设置FTP连接、浏览FTP目录、下载文件、处理FTP错误、实现自动化下载、处理大文件下载、使用多线程下载文件和处理文件断点续传。希望这些内容能帮助读者更好地掌握Python中与FTP相关的操作。
相关问答FAQs:
在使用Python从FTP服务器下载文件时,我应该使用哪个库?
Python中最常用的库是ftplib
,它是Python内置的库,可以方便地实现FTP操作,包括连接到FTP服务器、列出目录、下载文件等功能。您只需导入这个库并利用其提供的类和方法即可完成下载任务。
我需要提供哪些信息才能成功连接到FTP服务器?
要连接到FTP服务器,您通常需要提供服务器地址、用户名和密码。如果FTP服务器是公开的,您可能只需要地址和匿名用户名。确保在连接之前确认这些信息的正确性,以避免连接失败。
如何处理下载过程中的错误或异常情况?
在进行FTP下载时,可能会遇到多种异常情况,比如网络问题、文件不存在或权限不足等。使用Python的异常处理机制(try-except语句)可以有效捕获和处理这些错误,确保程序的健壮性。在捕获异常后,可以选择打印错误信息或进行其他处理,以便于后续的调试和改进。
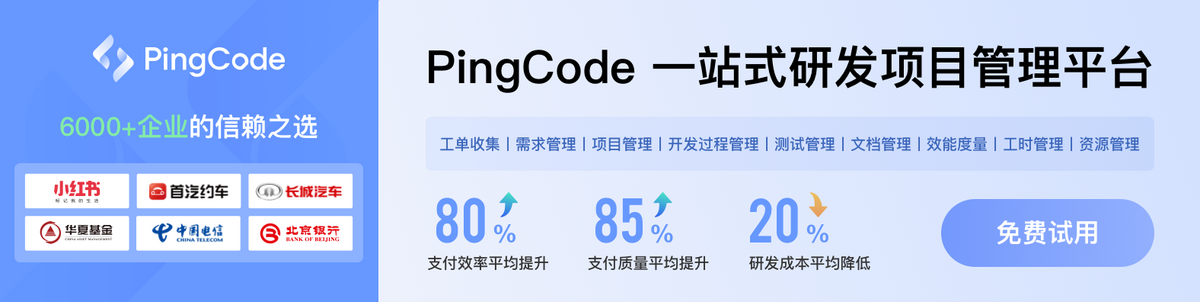