Python打开窗口首选项的方法有:使用tkinter库、使用PyQt库、使用wxPython库。在这些方法中,tkinter是Python的标准GUI库,易于使用和学习,非常适合初学者。以下将详细介绍如何使用tkinter库来打开窗口首选项。
一、Tkinter库
Tkinter是Python的标准GUI(Graphical User Interface)库。它提供了一些基本的组件,允许我们创建简单的图形用户界面。下面是一个使用Tkinter库打开窗口首选项的示例:
import tkinter as tk
from tkinter import messagebox
def show_preferences():
prefs_window = tk.Toplevel(root)
prefs_window.title("Preferences")
prefs_window.geometry("300x200")
tk.Label(prefs_window, text="Preference 1:").pack(pady=10)
tk.Entry(prefs_window).pack(pady=10)
tk.Label(prefs_window, text="Preference 2:").pack(pady=10)
tk.Entry(prefs_window).pack(pady=10)
tk.Button(prefs_window, text="Save", command=lambda: messagebox.showinfo("Info", "Preferences Saved")).pack(pady=10)
root = tk.Tk()
root.title("Main Window")
root.geometry("400x300")
tk.Button(root, text="Open Preferences", command=show_preferences).pack(pady=50)
root.mainloop()
在这个示例中,我们创建了一个主窗口root
,并在其中添加了一个按钮。当点击按钮时,会创建并打开一个新的顶级窗口prefs_window
,这个窗口包含一些标签、输入框和一个保存按钮。
二、使用PyQt库
PyQt是一个功能强大的Python GUI库,适用于创建复杂的图形用户界面。以下是如何使用PyQt库打开窗口首选项的示例:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton, QDialog, QVBoxLayout, QLabel, QLineEdit, QMessageBox
class PreferencesDialog(QDialog):
def __init__(self):
super().__init__()
self.setWindowTitle("Preferences")
self.setGeometry(100, 100, 300, 200)
layout = QVBoxLayout()
layout.addWidget(QLabel("Preference 1:"))
layout.addWidget(QLineEdit())
layout.addWidget(QLabel("Preference 2:"))
layout.addWidget(QLineEdit())
save_button = QPushButton("Save")
save_button.clicked.connect(self.save_preferences)
layout.addWidget(save_button)
self.setLayout(layout)
def save_preferences(self):
QMessageBox.information(self, "Info", "Preferences Saved")
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("Main Window")
self.setGeometry(100, 100, 400, 300)
open_prefs_button = QPushButton("Open Preferences", self)
open_prefs_button.clicked.connect(self.show_preferences)
self.setCentralWidget(open_prefs_button)
def show_preferences(self):
prefs_dialog = PreferencesDialog()
prefs_dialog.exec_()
app = QApplication(sys.argv)
main_window = MainWindow()
main_window.show()
sys.exit(app.exec_())
在这个示例中,我们使用PyQt创建了一个主窗口MainWindow
,并在其中添加了一个按钮。当点击按钮时,会创建并打开一个新的对话窗口PreferencesDialog
,这个窗口包含一些标签、输入框和一个保存按钮。
三、使用wxPython库
wxPython是另一个流行的Python GUI库,功能强大且易于使用。以下是如何使用wxPython库打开窗口首选项的示例:
import wx
class PreferencesDialog(wx.Dialog):
def __init__(self, parent):
super().__init__(parent, title="Preferences", size=(300, 200))
panel = wx.Panel(self)
vbox = wx.BoxSizer(wx.VERTICAL)
vbox.Add(wx.StaticText(panel, label="Preference 1:"), flag=wx.ALL, border=10)
vbox.Add(wx.TextCtrl(panel), flag=wx.ALL | wx.EXPAND, border=10)
vbox.Add(wx.StaticText(panel, label="Preference 2:"), flag=wx.ALL, border=10)
vbox.Add(wx.TextCtrl(panel), flag=wx.ALL | wx.EXPAND, border=10)
save_button = wx.Button(panel, label="Save")
save_button.Bind(wx.EVT_BUTTON, self.save_preferences)
vbox.Add(save_button, flag=wx.ALL | wx.CENTER, border=10)
panel.SetSizer(vbox)
def save_preferences(self, event):
wx.MessageBox("Preferences Saved", "Info", wx.OK | wx.ICON_INFORMATION)
class MainWindow(wx.Frame):
def __init__(self):
super().__init__(None, title="Main Window", size=(400, 300))
panel = wx.Panel(self)
vbox = wx.BoxSizer(wx.VERTICAL)
open_prefs_button = wx.Button(panel, label="Open Preferences")
open_prefs_button.Bind(wx.EVT_BUTTON, self.show_preferences)
vbox.Add(open_prefs_button, flag=wx.ALL | wx.CENTER, border=50)
panel.SetSizer(vbox)
def show_preferences(self, event):
prefs_dialog = PreferencesDialog(self)
prefs_dialog.ShowModal()
prefs_dialog.Destroy()
app = wx.App(False)
main_window = MainWindow()
main_window.Show()
app.MainLoop()
在这个示例中,我们使用wxPython创建了一个主窗口MainWindow
,并在其中添加了一个按钮。当点击按钮时,会创建并打开一个新的对话窗口PreferencesDialog
,这个窗口包含一些标签、输入框和一个保存按钮。
结论
以上介绍了三种使用Python打开窗口首选项的方法:Tkinter、PyQt和wxPython。每种方法都有其优点和适用场景,具体选择哪种方法取决于项目需求和个人偏好。Tkinter适合初学者,PyQt适合需要复杂GUI的项目,wxPython则提供了灵活且强大的GUI开发能力。通过这些示例,您可以根据自己的需求选择合适的库来实现窗口首选项的功能。
相关问答FAQs:
如何在Python中打开窗口首选项设置?
在Python中,打开窗口首选项通常涉及使用图形用户界面(GUI)库,如Tkinter、PyQt或wxPython。以Tkinter为例,可以通过创建一个设置窗口,使用Toplevel
类来实现。具体步骤包括导入Tkinter库,创建一个新的窗口实例,并在窗口中添加所需的设置选项。
在Python GUI中,哪些库最适合创建窗口首选项?
有多个库可以用来创建窗口首选项,最常用的包括Tkinter、PyQt和wxPython。Tkinter是Python的标准GUI库,易于使用且适合小型项目。PyQt功能强大,适合需要复杂界面的应用,而wxPython则提供了本地化的外观和感觉,适合需要跨平台支持的项目。
如何在Python应用程序中保存用户的窗口首选项?
在Python应用程序中保存用户窗口首选项可以通过多种方法实现,例如使用配置文件(如JSON或INI文件)来存储用户的设置。用户在首选项窗口中进行更改后,可以将这些值写入文件中,并在应用启动时读取这些文件以恢复设置。此外,还可以使用数据库存储更复杂的首选项数据。
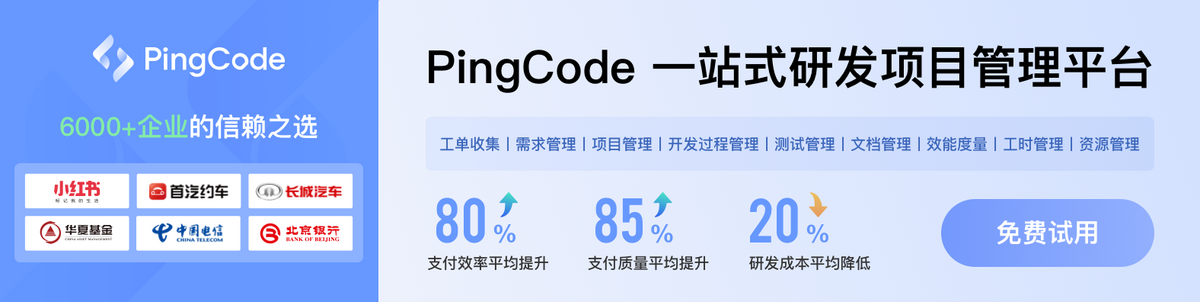