使用Python进行网页爬虫以取出网页中的所有文本,可以通过以下步骤:发送HTTP请求获取网页内容、使用HTML解析库解析网页、遍历所有文本节点并提取文本内容。 使用BeautifulSoup库解析HTML内容并提取网页文本是最常见的方法之一。以下是详细描述如何使用BeautifulSoup库来取出网页中的所有文本。
Python爬虫的基本步骤包括:发送HTTP请求获取网页内容、解析HTML文档、提取所需数据。常用的HTTP请求库是requests,解析HTML文档常用的是BeautifulSoup。下面是具体步骤和代码示例。
一、安装必要的库
在开始编写爬虫之前,确保已经安装了requests和BeautifulSoup库。可以使用以下命令安装这些库:
pip install requests
pip install beautifulsoup4
二、发送HTTP请求获取网页内容
使用requests库发送HTTP请求获取网页内容,并检查请求是否成功。
import requests
url = 'https://example.com' # 替换为目标网页的URL
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
else:
print(f"Failed to retrieve webpage. Status code: {response.status_code}")
三、使用BeautifulSoup解析HTML
将获取的HTML内容传递给BeautifulSoup进行解析,并指定解析器(通常使用'lxml'或'html.parser')。
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
四、提取网页中的所有文本
使用BeautifulSoup的.get_text()
方法,可以提取网页中的所有文本。这个方法会递归地获取所有文本节点并拼接成一个字符串。
page_text = soup.get_text(separator='\n', strip=True)
print(page_text)
五、处理和清理文本
提取的文本可能包含多余的空行或无用信息,可以进一步清理和处理文本。
cleaned_text = "\n".join([line.strip() for line in page_text.splitlines() if line.strip()])
print(cleaned_text)
六、保存提取的文本
将提取并清理后的文本保存到一个文件中,便于后续分析和处理。
with open('extracted_text.txt', 'w', encoding='utf-8') as f:
f.write(cleaned_text)
详细代码示例
以下是完整的代码示例,从发送请求到保存提取的文本:
import requests
from bs4 import BeautifulSoup
def fetch_webpage(url):
response = requests.get(url)
if response.status_code == 200:
return response.text
else:
print(f"Failed to retrieve webpage. Status code: {response.status_code}")
return None
def extract_text(html_content):
soup = BeautifulSoup(html_content, 'html.parser')
page_text = soup.get_text(separator='\n', strip=True)
cleaned_text = "\n".join([line.strip() for line in page_text.splitlines() if line.strip()])
return cleaned_text
def save_text_to_file(text, filename):
with open(filename, 'w', encoding='utf-8') as f:
f.write(text)
if __name__ == '__main__':
url = 'https://example.com' # 替换为目标网页的URL
html_content = fetch_webpage(url)
if html_content:
extracted_text = extract_text(html_content)
save_text_to_file(extracted_text, 'extracted_text.txt')
print("Text extracted and saved successfully.")
七、处理动态网页
有些网页内容是通过JavaScript动态加载的,requests库无法直接获取动态内容。可以使用Selenium库来处理动态网页。
- 安装Selenium和浏览器驱动程序:
pip install selenium
- 使用Selenium获取动态网页内容:
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
配置Selenium使用的浏览器驱动(以Chrome为例)
options = webdriver.ChromeOptions()
options.add_argument('--headless') # 无头模式,不打开浏览器窗口
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()), options=options)
访问网页
url = 'https://example.com' # 替换为目标网页的URL
driver.get(url)
获取网页内容
html_content = driver.page_source
关闭浏览器
driver.quit()
解析和提取文本
soup = BeautifulSoup(html_content, 'html.parser')
page_text = soup.get_text(separator='\n', strip=True)
cleaned_text = "\n".join([line.strip() for line in page_text.splitlines() if line.strip()])
print(cleaned_text)
使用Selenium获取动态网页内容后,可以继续使用BeautifulSoup解析和提取文本。
八、处理多页爬取
有些网页内容分布在多个页面,需要处理分页逻辑。可以通过循环请求分页URL来获取所有页面的内容。
base_url = 'https://example.com/page/' # 替换为分页的基础URL
all_text = []
for page_num in range(1, 6): # 假设需要爬取前5页
url = f"{base_url}{page_num}"
html_content = fetch_webpage(url)
if html_content:
extracted_text = extract_text(html_content)
all_text.append(extracted_text)
合并所有页面的文本
final_text = "\n".join(all_text)
print(final_text)
九、处理反爬虫机制
有些网站会使用反爬虫机制来阻止频繁的自动化请求。可以通过以下方法来减少被阻止的风险:
- 设置请求头部信息:模拟浏览器请求,增加随机等待时间。
- 使用代理IP:轮换使用多个代理IP。
- 控制请求频率:避免频繁请求,增加请求间隔时间。
import time
import random
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.36'
}
def fetch_webpage_with_headers(url):
response = requests.get(url, headers=headers)
if response.status_code == 200:
return response.text
else:
print(f"Failed to retrieve webpage. Status code: {response.status_code}")
return None
def fetch_webpage_with_delay(url):
html_content = fetch_webpage_with_headers(url)
time.sleep(random.uniform(1, 3)) # 随机等待1到3秒
return html_content
示例:获取多页内容
for page_num in range(1, 6):
url = f"{base_url}{page_num}"
html_content = fetch_webpage_with_delay(url)
if html_content:
extracted_text = extract_text(html_content)
all_text.append(extracted_text)
final_text = "\n".join(all_text)
print(final_text)
通过这些方法,可以有效地减少被网站阻止的风险,并提高爬虫的成功率。
十、总结
使用Python爬虫提取网页中的所有文本可以通过requests和BeautifulSoup库来实现。对于动态网页,可以使用Selenium库。处理多页爬取和反爬虫机制是提高爬虫成功率的重要手段。通过合理设置请求头部信息、使用代理IP和控制请求频率,可以有效减少被阻止的风险。通过这些方法,可以顺利提取网页中的所有文本。
相关问答FAQs:
如何使用Python爬虫提取网页中的文本数据?
要提取网页中的文本数据,可以使用Python的requests库获取网页内容,再利用BeautifulSoup库解析HTML。通过BeautifulSoup的find_all()方法,可以获取网页中所有的文本节点。示例代码如下:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
text = soup.get_text()
print(text)
是否需要处理网页中的HTML标签?
在提取文本时,HTML标签可能会干扰数据的整洁性。使用BeautifulSoup的.get_text()方法可以自动去除标签,提取纯文本。如果需要更细致的处理,可以通过指定特定的标签(如p、h1等)来提取相关部分的文本。
如何处理提取到的文本数据?
提取到的文本数据可能包含多余的空格和换行符。可以使用Python的字符串方法如strip()和replace()来清理这些多余的字符。此外,利用正则表达式可以进一步过滤文本内容,以满足特定的需求,比如去除特定的符号或格式化文本。
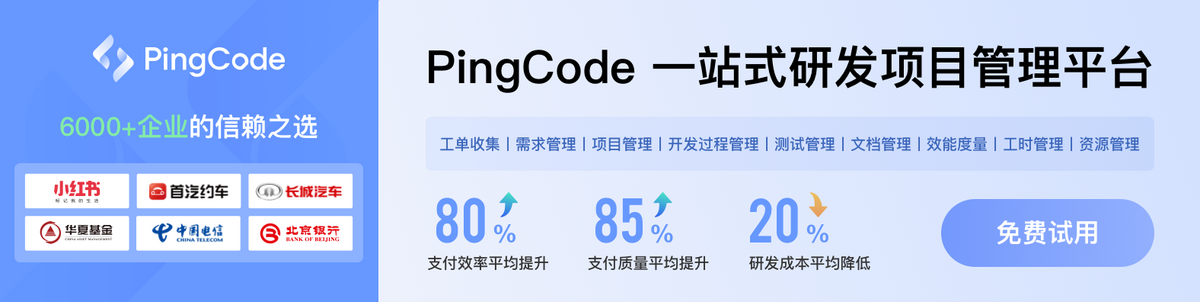