要将美元兑换为人民币,您可以使用Python编程语言通过调用在线API获取实时汇率,并进行计算。以下是一些关键步骤:使用API获取汇率、进行数据处理、进行汇率计算。
使用API获取汇率:这是最直接和简便的方法之一。通过调用一个提供实时外汇汇率的API,例如汇率API(Exchange Rate API),您可以获取最新的美元兑人民币汇率。下面我们详细描述如何实现这一点。
一、API获取汇率
要从API获取美元对人民币的实时汇率,首先需要选择一个可靠的API服务。目前有很多免费的和付费的API服务可以提供实时的汇率信息。以下是一些流行的API服务:
- ExchangeRate-API
- Open Exchange Rates
- XE Currency Data API
这里以ExchangeRate-API为例,描述如何使用它来获取美元对人民币的汇率。
1.1、注册并获取API密钥
首先,您需要注册一个ExchangeRate-API的账户,并获取一个API密钥。这个密钥用于验证您的请求。
1.2、发送API请求
在获取到API密钥后,您可以使用Python的requests库来发送API请求,并获取汇率数据。以下是一个示例代码:
import requests
def get_exchange_rate(api_key):
url = f"https://v6.exchangerate-api.com/v6/{api_key}/latest/USD"
response = requests.get(url)
data = response.json()
return data['conversion_rates']['CNY']
api_key = 'your_api_key_here'
usd_to_cny_rate = get_exchange_rate(api_key)
print(f"Current USD to CNY exchange rate: {usd_to_cny_rate}")
在这个示例中,我们定义了一个函数get_exchange_rate
,它发送一个API请求以获取美元对人民币的汇率。您需要将your_api_key_here
替换为您自己的API密钥。
二、数据处理与汇率计算
获取到汇率后,下一步就是进行数据处理和汇率计算。我们可以使用简单的数学运算来完成这一任务。
2.1、定义汇率计算函数
我们可以定义一个函数来将美元金额转换为人民币金额:
def convert_usd_to_cny(amount_usd, exchange_rate):
return amount_usd * exchange_rate
2.2、使用汇率进行转换
假设我们有一个美元金额为100美元,我们可以使用上述函数将其转换为人民币:
amount_usd = 100
amount_cny = convert_usd_to_cny(amount_usd, usd_to_cny_rate)
print(f"{amount_usd} USD is equal to {amount_cny} CNY")
三、处理异常情况
在实际应用中,您可能会遇到各种异常情况,例如API请求失败、网络连接错误等。因此,添加异常处理是非常重要的。以下是一个示例代码,展示了如何处理API请求失败的情况:
import requests
def get_exchange_rate(api_key):
try:
url = f"https://v6.exchangerate-api.com/v6/{api_key}/latest/USD"
response = requests.get(url)
response.raise_for_status()
data = response.json()
return data['conversion_rates']['CNY']
except requests.exceptions.RequestException as e:
print(f"Error fetching exchange rate: {e}")
return None
api_key = 'your_api_key_here'
usd_to_cny_rate = get_exchange_rate(api_key)
if usd_to_cny_rate:
amount_usd = 100
amount_cny = convert_usd_to_cny(amount_usd, usd_to_cny_rate)
print(f"{amount_usd} USD is equal to {amount_cny} CNY")
else:
print("Failed to fetch exchange rate.")
在这个示例中,我们使用了try
和except
块来捕获和处理API请求中的异常情况。如果API请求失败,函数将返回None
,并在控制台中打印错误消息。
四、扩展与优化
在实际应用中,您可能需要处理更多的情况,例如缓存汇率以减少API调用次数、处理不同的货币对、以及创建一个更友好的用户界面。以下是一些可能的扩展和优化建议:
4.1、缓存汇率
为了减少API调用次数,您可以将汇率缓存一段时间。例如,您可以将汇率存储在一个文件或内存中,并在一定时间(例如1小时)后更新汇率。
import time
import requests
cache = {}
cache_expiry = 3600 # Cache expiry time in seconds
def get_exchange_rate(api_key):
current_time = time.time()
if 'rate' in cache and (current_time - cache['timestamp'] < cache_expiry):
return cache['rate']
try:
url = f"https://v6.exchangerate-api.com/v6/{api_key}/latest/USD"
response = requests.get(url)
response.raise_for_status()
data = response.json()
cache['rate'] = data['conversion_rates']['CNY']
cache['timestamp'] = current_time
return cache['rate']
except requests.exceptions.RequestException as e:
print(f"Error fetching exchange rate: {e}")
return None
api_key = 'your_api_key_here'
usd_to_cny_rate = get_exchange_rate(api_key)
if usd_to_cny_rate:
amount_usd = 100
amount_cny = convert_usd_to_cny(amount_usd, usd_to_cny_rate)
print(f"{amount_usd} USD is equal to {amount_cny} CNY")
else:
print("Failed to fetch exchange rate.")
4.2、处理不同的货币对
您可以扩展程序以处理不同的货币对。为此,您可以修改get_exchange_rate
函数,使其接受两个参数:源货币和目标货币。
import requests
def get_exchange_rate(api_key, from_currency, to_currency):
try:
url = f"https://v6.exchangerate-api.com/v6/{api_key}/latest/{from_currency}"
response = requests.get(url)
response.raise_for_status()
data = response.json()
return data['conversion_rates'][to_currency]
except requests.exceptions.RequestException as e:
print(f"Error fetching exchange rate: {e}")
return None
api_key = 'your_api_key_here'
usd_to_cny_rate = get_exchange_rate(api_key, 'USD', 'CNY')
if usd_to_cny_rate:
amount_usd = 100
amount_cny = convert_usd_to_cny(amount_usd, usd_to_cny_rate)
print(f"{amount_usd} USD is equal to {amount_cny} CNY")
else:
print("Failed to fetch exchange rate.")
通过这种方式,您可以动态地获取不同货币对的汇率,并进行相应的转换。
4.3、创建用户界面
为了使程序更易于使用,您可以为其创建一个简单的图形用户界面(GUI)。您可以使用Tkinter库来创建一个基本的GUI应用程序。
import tkinter as tk
from tkinter import messagebox
import requests
def get_exchange_rate(api_key, from_currency, to_currency):
try:
url = f"https://v6.exchangerate-api.com/v6/{api_key}/latest/{from_currency}"
response = requests.get(url)
response.raise_for_status()
data = response.json()
return data['conversion_rates'][to_currency]
except requests.exceptions.RequestException as e:
messagebox.showerror("Error", f"Error fetching exchange rate: {e}")
return None
def convert_currency():
api_key = 'your_api_key_here'
from_currency = from_currency_var.get()
to_currency = to_currency_var.get()
amount = float(amount_var.get())
rate = get_exchange_rate(api_key, from_currency, to_currency)
if rate:
converted_amount = amount * rate
result_var.set(f"{amount} {from_currency} is equal to {converted_amount:.2f} {to_currency}")
app = tk.Tk()
app.title("Currency Converter")
tk.Label(app, text="From Currency:").grid(row=0, column=0)
from_currency_var = tk.StringVar(value='USD')
tk.Entry(app, textvariable=from_currency_var).grid(row=0, column=1)
tk.Label(app, text="To Currency:").grid(row=1, column=0)
to_currency_var = tk.StringVar(value='CNY')
tk.Entry(app, textvariable=to_currency_var).grid(row=1, column=1)
tk.Label(app, text="Amount:").grid(row=2, column=0)
amount_var = tk.StringVar(value='100')
tk.Entry(app, textvariable=amount_var).grid(row=2, column=1)
tk.Button(app, text="Convert", command=convert_currency).grid(row=3, column=0, columnspan=2)
result_var = tk.StringVar()
tk.Label(app, textvariable=result_var).grid(row=4, column=0, columnspan=2)
app.mainloop()
在这个示例中,我们使用Tkinter库创建了一个简单的GUI应用程序,用户可以输入源货币、目标货币和金额,然后点击“Convert”按钮进行汇率转换。转换结果会显示在应用程序中。
五、总结
通过本文的介绍,我们详细描述了如何使用Python将美元兑换为人民币。我们介绍了使用API获取实时汇率、进行数据处理和汇率计算的方法。同时,我们还讨论了如何处理异常情况,并提供了一些扩展和优化建议。希望这些内容对您有所帮助,让您能够更加高效地实现汇率转换功能。
相关问答FAQs:
如何使用Python获取美元对人民币的实时汇率?
可以通过多种API获取实时汇率信息,例如使用开放的金融数据API(如ExchangeRate-API、Open Exchange Rates等)。通过Python的requests库发送HTTP请求,您可以轻松获取最新的汇率数据,并进行解析和转换。
在Python中如何将美元金额转换为人民币?
您可以使用获取的实时汇率,将美元金额乘以当前的汇率值。示例代码如下:
usd_amount = 100 # 美元金额
exchange_rate = 6.5 # 假设当前汇率为6.5
rmb_amount = usd_amount * exchange_rate
print(f"{usd_amount} 美元等于 {rmb_amount} 人民币")
确保在实际应用中使用最新的汇率数据,以确保转换的准确性。
Python中有哪些库可以帮助处理货币转换?
除了requests库,您还可以使用pandas库来处理和分析汇率数据。通过将汇率数据存储在DataFrame中,您可以轻松进行各种转换和统计分析。此外,forex-python是一个专门用于货币转换的库,使用起来也非常方便,支持多种货币之间的转换。
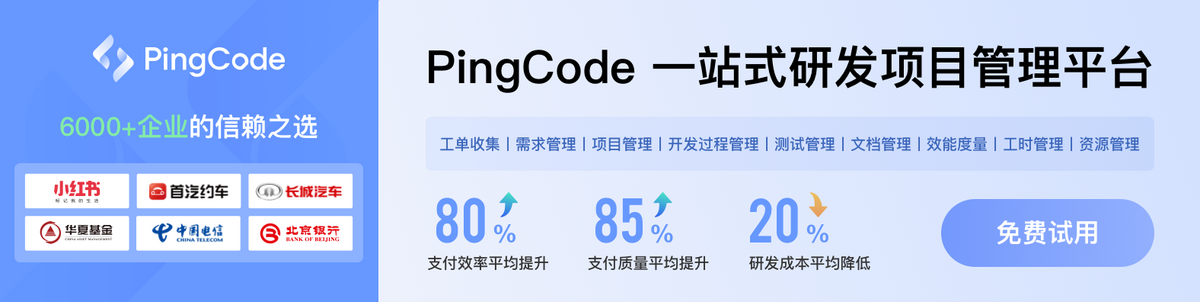