Python在字符串处理方面功能强大、灵活、易用。其中包括字符串切片、拼接、查找、替换、格式化等操作。使用Python的内置字符串操作函数和库,如re库,可以极大地方便字符串的处理。让我们详细探讨如何用Python完成各种字符串题目。
一、字符串基础操作
1. 字符串创建和赋值
在Python中,可以使用单引号、双引号或三引号创建字符串。三引号允许字符串跨行。
# 使用单引号
str1 = 'Hello, World!'
使用双引号
str2 = "Hello, Python!"
使用三引号
str3 = """Hello,
World!
Welcome to Python."""
2. 字符串拼接
字符串拼接是将多个字符串合并成一个字符串的操作。可以使用+
运算符或join
方法。
# 使用+运算符
str4 = str1 + " " + str2
print(str4) # 输出: Hello, World! Hello, Python!
使用join方法
str_list = [str1, str2]
str5 = " ".join(str_list)
print(str5) # 输出: Hello, World! Hello, Python!
3. 字符串切片
字符串切片是从字符串中提取子字符串的一种方法。使用切片操作符[]
。
str6 = "Hello, World!"
提取子字符串
substring = str6[0:5]
print(substring) # 输出: Hello
从字符串末尾开始计数
substring = str6[-6:]
print(substring) # 输出: World!
二、字符串查找和替换
1. 字符串查找
查找字符串中的子字符串,可以使用find
和index
方法。
str7 = "Hello, World!"
使用find方法
pos = str7.find("World")
print(pos) # 输出: 7
使用index方法
pos = str7.index("World")
print(pos) # 输出: 7
2. 字符串替换
替换字符串中的子字符串,可以使用replace
方法。
str8 = "Hello, World!"
使用replace方法
new_str = str8.replace("World", "Python")
print(new_str) # 输出: Hello, Python!
三、字符串格式化
1. 使用百分号(%)进行格式化
这种方法类似于C语言的printf。
name = "Alice"
age = 30
formatted_str = "Name: %s, Age: %d" % (name, age)
print(formatted_str) # 输出: Name: Alice, Age: 30
2. 使用str.format
方法
这种方法更强大,灵活性更高。
name = "Bob"
age = 25
formatted_str = "Name: {}, Age: {}".format(name, age)
print(formatted_str) # 输出: Name: Bob, Age: 25
3. 使用f字符串(f-strings)
这是Python 3.6及以后的版本提供的一种更简洁的格式化方法。
name = "Charlie"
age = 22
formatted_str = f"Name: {name}, Age: {age}"
print(formatted_str) # 输出: Name: Charlie, Age: 22
四、字符串高级操作
1. 正则表达式
正则表达式是用于匹配字符串的一种强大工具。在Python中,可以使用re
模块进行正则表达式操作。
import re
str9 = "Hello, World! Welcome to Python."
使用正则表达式查找
matches = re.findall(r'\b\w{5}\b', str9)
print(matches) # 输出: ['Hello', 'World']
使用正则表达式替换
new_str = re.sub(r'World', 'Universe', str9)
print(new_str) # 输出: Hello, Universe! Welcome to Python.
2. 字符串去空格和拆分
去除字符串两端的空格,可以使用strip
方法;拆分字符串为列表,可以使用split
方法。
str10 = " Hello, Python! "
去除两端空格
stripped_str = str10.strip()
print(stripped_str) # 输出: Hello, Python!
拆分字符串
words = stripped_str.split()
print(words) # 输出: ['Hello,', 'Python!']
五、字符串编码和解码
在处理非ASCII字符时,理解字符串的编码和解码非常重要。
# 编码
str11 = "你好,世界!"
encoded_str = str11.encode('utf-8')
print(encoded_str) # 输出: b'\xe4\xbd\xa0\xe5\xa5\xbd\xef\xbc\x8c\xe4\xb8\x96\xe7\x95\x8c\xef\xbc\x81'
解码
decoded_str = encoded_str.decode('utf-8')
print(decoded_str) # 输出: 你好,世界!
六、字符串常见面试题
1. 判断回文字符串
回文字符串是指正读和反读都相同的字符串。
def is_palindrome(s):
return s == s[::-1]
print(is_palindrome("radar")) # 输出: True
print(is_palindrome("hello")) # 输出: False
2. 统计字符串中每个字符的出现次数
from collections import Counter
def char_count(s):
return Counter(s)
print(char_count("hello world")) # 输出: Counter({'l': 3, 'o': 2, 'h': 1, 'e': 1, ' ': 1, 'w': 1, 'r': 1, 'd': 1})
3. 反转字符串中的单词
def reverse_words(s):
words = s.split()
return " ".join(reversed(words))
print(reverse_words("Hello World")) # 输出: World Hello
4. 删除字符串中的指定字符
def remove_char(s, char):
return s.replace(char, '')
print(remove_char("hello world", 'l')) # 输出: heo word
七、字符串处理的实际应用
1. 日志文件处理
在实际应用中,处理日志文件常常需要对字符串进行各种操作。
log = """INFO 2023-01-01 10:00:00 - User logged in
ERROR 2023-01-01 10:05:00 - Failed to connect to database
INFO 2023-01-01 10:10:00 - User logged out"""
提取所有错误日志
error_logs = [line for line in log.split('\n') if 'ERROR' in line]
print(error_logs) # 输出: ['ERROR 2023-01-01 10:05:00 - Failed to connect to database']
2. 网页数据提取
使用正则表达式从HTML中提取数据。
html = """
<html>
<head><title>Example</title></head>
<body>
<p class="content">Hello, World!</p>
<p class="content">Welcome to Python.</p>
</body>
</html>
"""
提取所有段落内容
content = re.findall(r'<p class="content">(.*?)</p>', html)
print(content) # 输出: ['Hello, World!', 'Welcome to Python.']
3. 文本数据清理
在处理自然语言数据时,通常需要对文本进行清理,包括去除标点符号、转换大小写等。
import string
text = "Hello, World! Welcome to Python."
去除标点符号
cleaned_text = text.translate(str.maketrans('', '', string.punctuation))
print(cleaned_text) # 输出: Hello World Welcome to Python
转换为小写
lower_text = cleaned_text.lower()
print(lower_text) # 输出: hello world welcome to python
八、字符串处理的性能优化
在处理大规模字符串数据时,性能优化显得尤为重要。
1. 使用生成器
生成器在处理大数据集时可以节省内存。
def read_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line.strip()
for line in read_large_file('large_file.txt'):
print(line)
2. 避免重复拼接
在循环中重复拼接字符串会导致性能问题。可以使用列表和join
方法来优化。
# 不推荐
result = ""
for i in range(1000):
result += str(i)
推荐
result = []
for i in range(1000):
result.append(str(i))
result = ''.join(result)
九、总结
Python提供了丰富的字符串处理功能,包括基本操作、查找替换、格式化、高级操作等。通过充分利用这些功能,可以高效地完成各种字符串处理任务。同时,在实际应用中,理解和掌握字符串处理的性能优化方法,可以提高代码的执行效率。在解决字符串相关问题时,合理选择合适的方法和工具,将事半功倍。
无论是基本的字符串操作,还是复杂的正则表达式处理,Python都提供了强大而灵活的解决方案。希望通过本文的介绍,能够帮助读者更好地理解和掌握Python中的字符串处理技术。
相关问答FAQs:
如何在Python中处理字符串的常见方法有哪些?
在Python中,字符串处理非常灵活且强大。常见的方法包括字符串拼接(使用+
运算符或join()
方法)、切片(通过索引获取子字符串)、查找(使用find()
或index()
方法)、替换(使用replace()
方法)和大小写转换(使用upper()
、lower()
、capitalize()
等方法)。这些方法可以帮助开发者高效地操作和处理字符串数据。
Python中有没有内置的字符串格式化功能?
Python提供了多种字符串格式化方法,包括使用f-string
(格式化字符串字面量)、str.format()
方法和百分号(%
)格式化。f-string
是Python 3.6及以上版本中引入的,使用方便且可读性高,允许直接在字符串中嵌入表达式。str.format()
则提供了更灵活的格式控制选项,适合复杂的字符串构建需求。
如何高效地处理大量字符串数据?
处理大量字符串数据时,可以考虑使用join()
方法进行拼接,因为它比直接使用+
运算符更高效。对于需要频繁修改的字符串,可以使用list
来存储各个部分,最后再使用join()
将它们合并。此外,使用正则表达式(re
模块)可以高效地进行复杂的字符串搜索和替换操作,适用于大规模文本处理。
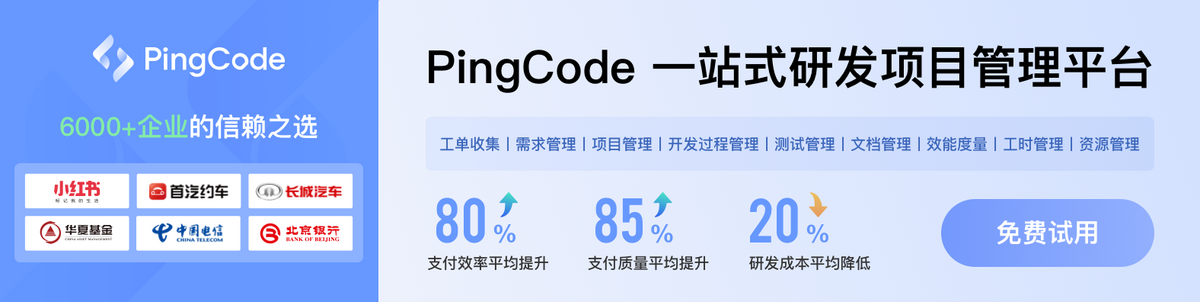