Python实现两个函数的调用可以通过以下几种方式:直接调用、嵌套调用、函数作为参数传递、函数作为返回值等。本文将详细介绍这些方法,并提供相关示例代码。
一、直接调用
在Python中,最简单的函数调用方式是直接调用。直接调用是指在一个函数中直接调用另一个函数。通过这种方式,可以实现两个函数的调用。
def func1():
print("This is function 1")
def func2():
print("This is function 2")
func1() # 直接调用func1
func2()
在上述代码中,func2
函数中直接调用了func1
函数。当执行func2
函数时,会首先打印"This is function 2",然后调用func1
函数,打印"This is function 1"。
二、嵌套调用
嵌套调用是指在一个函数中嵌套调用另一个函数。这种方式可以实现更复杂的函数调用。
def func1():
print("This is function 1")
def func2():
def func3():
print("This is function 3")
func1() # 嵌套调用func1
func3()
print("This is function 2")
func2()
在上述代码中,func3
函数嵌套在func2
函数中。在func3
函数中调用了func1
函数。当执行func2
函数时,会首先调用func3
函数,打印"This is function 3",然后调用func1
函数,打印"This is function 1",最后打印"This is function 2"。
三、函数作为参数传递
Python函数可以作为参数传递给另一个函数。这种方式可以实现更灵活的函数调用。
def func1():
print("This is function 1")
def func2(func):
print("This is function 2")
func() # 调用传递的函数
func2(func1)
在上述代码中,func2
函数接受一个函数作为参数。在调用func2
函数时,将func1
函数作为参数传递给func2
函数。在func2
函数中,通过调用传递的函数来实现函数的调用。
四、函数作为返回值
Python函数可以返回另一个函数。这种方式可以实现函数的链式调用。
def func1():
print("This is function 1")
def func2():
print("This is function 2")
return func1 # 返回func1函数
returned_func = func2()
returned_func() # 调用返回的函数
在上述代码中,func2
函数返回func1
函数。当执行func2
函数时,会打印"This is function 2",并返回func1
函数。然后,通过调用返回的func1
函数,实现函数的链式调用。
五、装饰器实现函数调用
装饰器是Python中的一种特殊函数,用于在不改变原函数的情况下,扩展其功能。通过装饰器,可以实现更灵活的函数调用。
def func1():
print("This is function 1")
def decorator(func):
def wrapper():
print("This is the decorator")
func() # 调用被装饰的函数
return wrapper
@decorator
def func2():
print("This is function 2")
func2()
在上述代码中,decorator
函数是一个装饰器,它接受一个函数作为参数,并返回一个包装函数。在包装函数中,首先打印"This is the decorator",然后调用被装饰的函数。通过使用@decorator
语法,将func2
函数装饰为decorator
函数。当执行func2
函数时,会首先打印"This is the decorator",然后调用func2
函数,打印"This is function 2"。
六、类方法调用
在面向对象编程中,可以通过类方法实现函数的调用。类方法可以直接调用类中的其他方法。
class MyClass:
def func1(self):
print("This is function 1")
def func2(self):
print("This is function 2")
self.func1() # 调用类中的func1方法
obj = MyClass()
obj.func2()
在上述代码中,MyClass
类中定义了两个方法func1
和func2
。在func2
方法中,通过self
关键字调用了func1
方法。当创建MyClass
类的实例并调用func2
方法时,会首先打印"This is function 2",然后调用func1
方法,打印"This is function 1"。
七、递归调用
递归调用是指函数在其定义中调用自身。通过递归调用,可以实现一些复杂的算法,如斐波那契数列、阶乘等。
def factorial(n):
if n == 1:
return 1
else:
return n * factorial(n - 1) # 递归调用自身
print(factorial(5))
在上述代码中,factorial
函数是一个递归函数,用于计算阶乘。当n
等于1时,返回1;否则,返回n
乘以factorial(n - 1)
。通过递归调用自身,实现了阶乘的计算。
八、生成器函数调用
生成器函数是Python中的一种特殊函数,通过yield
关键字返回一个生成器对象。生成器对象可以用于迭代,并在每次迭代时生成一个值。
def generator_func():
yield "This is function 1"
yield "This is function 2"
gen = generator_func()
for value in gen:
print(value)
在上述代码中,generator_func
是一个生成器函数,通过yield
关键字返回两个值。当调用generator_func
函数时,返回一个生成器对象。通过迭代生成器对象,可以依次获取生成器函数返回的值,并打印"This is function 1"和"This is function 2"。
总结
本文详细介绍了Python实现两个函数调用的多种方法,包括直接调用、嵌套调用、函数作为参数传递、函数作为返回值、装饰器实现函数调用、类方法调用、递归调用和生成器函数调用。每种方法都具有其独特的特点和应用场景,可以根据具体需求选择合适的实现方式。通过掌握这些方法,可以更灵活地实现函数的调用和组合,编写出更加高效和优雅的代码。
相关问答FAQs:
如何在Python中实现函数之间的调用?
在Python中,函数可以通过直接调用其他函数来实现相互之间的调用。只需在一个函数的内部使用另一个函数的名称并传递所需的参数。例如,您可以定义一个函数来计算平方,再定义另一个函数来计算立方,并在立方的函数中调用平方的函数。
在函数调用中如何传递参数?
参数传递是函数调用中的重要部分。在定义函数时,您可以指定参数,在调用时将实际值传递给这些参数。可以通过位置参数、关键字参数或可变参数等多种方式传递参数,以满足不同的需求。
如果一个函数需要返回多个值,应该如何实现?
在Python中,函数可以通过元组、列表或字典等数据结构返回多个值。您只需在函数中使用逗号分隔多个返回值,然后在调用函数时可以通过解包方式获取这些值。例如,您可以创建一个函数返回两个数的和与差,并在调用时将其分配给两个变量。
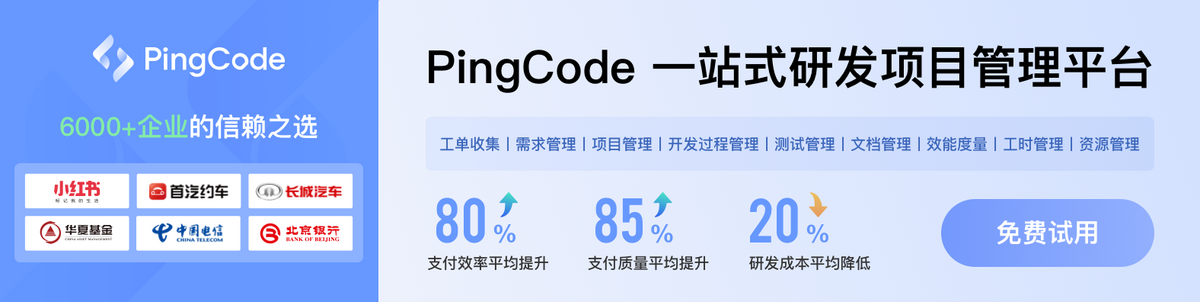