在Python中,可以使用装饰器、全局变量、类属性等方法来确保函数只执行一次。装饰器是一种常见且优雅的解决方案,因为它们可以将函数的行为进行修改,而不改变函数本身。以下详细描述如何使用装饰器来实现函数只执行一次的方法。
一、使用装饰器
装饰器是一种高级Python功能,可以在不改变函数本身的情况下修改其行为。通过使用装饰器,可以轻松实现函数只执行一次的要求。
def only_once(func):
has_run = False
def wrapper(*args, kwargs):
nonlocal has_run
if not has_run:
has_run = True
return func(*args, kwargs)
else:
print("This function has already been executed.")
return None
return wrapper
@only_once
def my_function():
print("Function is executed")
Testing the function
my_function() # Output: Function is executed
my_function() # Output: This function has already been executed.
装饰器的优点是简单、直观、易于维护,并且可以在不改变函数本身的情况下实现新的功能。
二、使用全局变量
除了装饰器,还可以使用全局变量来控制函数的执行次数。通过定义一个全局变量并在函数内部检查其状态,可以确保函数只执行一次。
has_run = False
def my_function():
global has_run
if not has_run:
has_run = True
print("Function is executed")
else:
print("This function has already been executed.")
Testing the function
my_function() # Output: Function is executed
my_function() # Output: This function has already been executed.
三、使用类属性
使用类属性也可以实现函数只执行一次的效果。通过将函数封装在类中,并使用类属性来记录函数的执行状态,可以确保函数只执行一次。
class MyClass:
has_run = False
@classmethod
def my_function(cls):
if not cls.has_run:
cls.has_run = True
print("Function is executed")
else:
print("This function has already been executed.")
Testing the function
MyClass.my_function() # Output: Function is executed
MyClass.my_function() # Output: This function has already been executed.
四、使用闭包
闭包是一种函数编程技术,可以捕获和保存其作用域中的局部变量。通过使用闭包,可以创建一个只执行一次的函数。
def only_once():
has_run = False
def inner_function():
nonlocal has_run
if not has_run:
has_run = True
print("Function is executed")
else:
print("This function has already been executed.")
return inner_function
Creating the function
my_function = only_once()
Testing the function
my_function() # Output: Function is executed
my_function() # Output: This function has already been executed.
五、使用单例模式
单例模式是一种设计模式,确保某个类只有一个实例,并提供一个全局访问点。通过使用单例模式,可以确保某个函数只执行一次。
class Singleton:
_instance = None
def __new__(cls, *args, kwargs):
if not cls._instance:
cls._instance = super(Singleton, cls).__new__(cls, *args, kwargs)
return cls._instance
def __init__(self):
if not hasattr(self, 'has_run'):
self.has_run = False
def my_function(self):
if not self.has_run:
self.has_run = True
print("Function is executed")
else:
print("This function has already been executed.")
Creating the singleton instance
singleton = Singleton()
Testing the function
singleton.my_function() # Output: Function is executed
singleton.my_function() # Output: This function has already been executed.
六、使用线程锁
在多线程环境中,可以使用线程锁(Lock)来确保函数只执行一次。线程锁是一种同步原语,用于防止多个线程同时访问共享资源。
import threading
lock = threading.Lock()
has_run = False
def my_function():
global has_run
with lock:
if not has_run:
has_run = True
print("Function is executed")
else:
print("This function has already been executed.")
Testing the function
my_function() # Output: Function is executed
my_function() # Output: This function has already been executed.
通过使用线程锁,可以确保在多线程环境中,函数只执行一次,避免竞争条件和数据不一致的问题。
七、使用函数属性
函数属性是Python函数的一个特性,可以为函数添加自定义属性。通过使用函数属性,可以控制函数的执行状态,确保函数只执行一次。
def my_function():
if not hasattr(my_function, 'has_run'):
my_function.has_run = False
if not my_function.has_run:
my_function.has_run = True
print("Function is executed")
else:
print("This function has already been executed.")
Testing the function
my_function() # Output: Function is executed
my_function() # Output: This function has already been executed.
八、使用缓存机制
使用缓存机制也可以实现函数只执行一次的效果。通过缓存函数的执行结果,可以确保函数只执行一次,并在后续调用中直接返回缓存结果。
from functools import lru_cache
@lru_cache(maxsize=1)
def my_function():
print("Function is executed")
return "Result"
Testing the function
my_function() # Output: Function is executed
my_function() # No output, result is returned from cache
总结
在Python中,有多种方法可以确保函数只执行一次,包括装饰器、全局变量、类属性、闭包、单例模式、线程锁、函数属性和缓存机制。每种方法都有其优点和适用场景,可以根据实际需求选择合适的解决方案。
使用装饰器是一种常见且优雅的解决方案,因为它们可以将函数的行为进行修改,而不改变函数本身。全局变量和类属性适用于简单的场景,而闭包和单例模式则适用于更复杂的需求。线程锁用于多线程环境,确保函数只执行一次。函数属性和缓存机制则提供了其他灵活的解决方案。
选择合适的方法来确保函数只执行一次,可以提高代码的可读性、可维护性和运行效率。
相关问答FAQs:
如何在Python中确保函数只被调用一次?
在Python中,可以使用装饰器来确保一个函数只执行一次。装饰器可以封装函数调用的逻辑,并通过状态变量来控制是否执行。例如,可以创建一个once
装饰器,在第一次调用时执行函数,之后返回一个已缓存的结果。
使用什么方法可以记录函数的执行状态?
可以利用Python中的闭包来记录函数的执行状态。通过定义一个内部函数并在外部函数中使用变量来保存状态,可以确保外部函数在执行后不再调用内部函数。这种方式简洁且易于维护。
是否有内置的库可以帮助实现函数只执行一次的功能?
Python的functools
模块提供了lru_cache
装饰器,可以缓存函数的返回值,从而避免重复计算。虽然它的主要用途是缓存结果,但也可以通过合适的参数设置来实现只执行一次的效果。此方法适用于需要重复调用但结果不变的场景。
在多线程环境中,如何确保函数只执行一次?
在多线程环境下,可以使用threading
模块中的锁(Lock)来确保函数只执行一次。通过在函数开始时获取锁,确保同一时间只有一个线程可以执行该函数,从而避免数据竞争和不一致的状态。确保在函数结束时释放锁,以便其他线程可以继续执行。
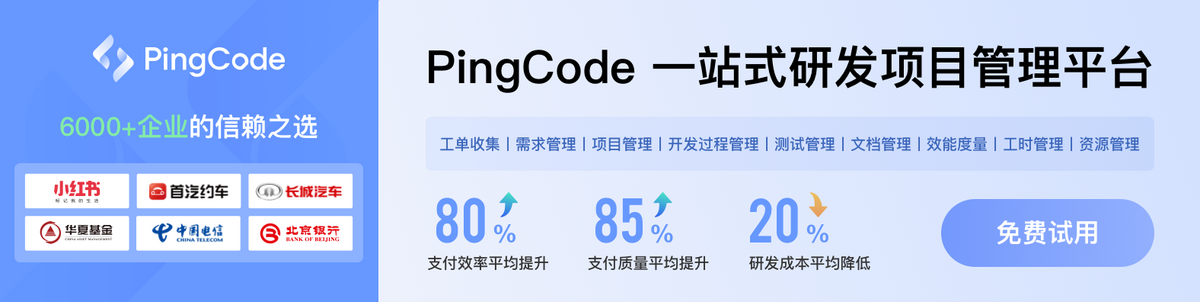