Python查看数据库表是否存在的方法包括:使用SQL语句、使用数据库模块提供的函数、通过捕获异常来判断。 在本文中,我们将详细描述这些方法,并提供示例代码以帮助你更好地理解和使用它们。
一、使用SQL语句
使用SQL语句是查看数据库表是否存在的常见方法之一。不同的数据库管理系统(DBMS)可能会有不同的SQL语句。以下是一些常见的DBMS及其相应的SQL语句:
1.1 MySQL
在MySQL中,可以使用SHOW TABLES
命令来查看数据库中的所有表,并检查指定的表是否存在:
import mysql.connector
def check_table_exists(cursor, table_name):
cursor.execute("SHOW TABLES LIKE %s", (table_name,))
result = cursor.fetchone()
return result is not None
示例
conn = mysql.connector.connect(user='yourusername', password='yourpassword', host='localhost', database='yourdatabase')
cursor = conn.cursor()
table_exists = check_table_exists(cursor, 'yourtable')
print(f"Table exists: {table_exists}")
1.2 PostgreSQL
在PostgreSQL中,可以查询information_schema.tables
视图来检查表是否存在:
import psycopg2
def check_table_exists(cursor, table_name):
cursor.execute("""
SELECT EXISTS (
SELECT 1
FROM information_schema.tables
WHERE table_name = %s
);
""", (table_name,))
result = cursor.fetchone()
return result[0]
示例
conn = psycopg2.connect("dbname=yourdatabase user=yourusername password=yourpassword host=localhost")
cursor = conn.cursor()
table_exists = check_table_exists(cursor, 'yourtable')
print(f"Table exists: {table_exists}")
1.3 SQLite
在SQLite中,可以查询sqlite_master
表来检查表是否存在:
import sqlite3
def check_table_exists(cursor, table_name):
cursor.execute("""
SELECT name
FROM sqlite_master
WHERE type='table' AND name=?
""", (table_name,))
result = cursor.fetchone()
return result is not None
示例
conn = sqlite3.connect('yourdatabase.db')
cursor = conn.cursor()
table_exists = check_table_exists(cursor, 'yourtable')
print(f"Table exists: {table_exists}")
二、使用数据库模块提供的函数
某些数据库模块提供了专门的函数来检查表是否存在。以下是一些示例:
2.1 SQLAlchemy
SQLAlchemy是一个功能强大的Python SQL工具包和对象关系映射器(ORM),它提供了一种简便的方法来检查表是否存在:
from sqlalchemy import create_engine, inspect
engine = create_engine('mysql+mysqlconnector://yourusername:yourpassword@localhost/yourdatabase')
inspector = inspect(engine)
def check_table_exists(inspector, table_name):
return inspector.has_table(table_name)
示例
table_exists = check_table_exists(inspector, 'yourtable')
print(f"Table exists: {table_exists}")
2.2 Django ORM
如果你使用Django框架,Django ORM提供了检查表是否存在的方法:
from django.db import connection
def check_table_exists(table_name):
with connection.cursor() as cursor:
cursor.execute("""
SELECT EXISTS (
SELECT 1
FROM information_schema.tables
WHERE table_name = %s
);
""", [table_name])
result = cursor.fetchone()
return result[0]
示例
table_exists = check_table_exists('yourtable')
print(f"Table exists: {table_exists}")
三、通过捕获异常来判断
在某些情况下,你可以尝试执行一个针对目标表的操作,并通过捕获异常来判断表是否存在。这种方法虽然不太优雅,但在某些情况下可能会很有用:
import sqlite3
def check_table_exists(conn, table_name):
try:
conn.execute(f"SELECT 1 FROM {table_name} LIMIT 1;")
return True
except sqlite3.OperationalError:
return False
示例
conn = sqlite3.connect('yourdatabase.db')
table_exists = check_table_exists(conn, 'yourtable')
print(f"Table exists: {table_exists}")
总结
通过使用SQL语句、数据库模块提供的函数或捕获异常来判断数据库表是否存在,可以有效地处理不同的数据库管理系统。选择合适的方法取决于你的具体需求和使用的DBMS。希望这篇文章能帮助你更好地理解和实现Python查看数据库表是否存在的方法。
相关问答FAQs:
如何使用Python连接到数据库?
要在Python中连接到数据库,您需要使用适当的数据库驱动程序,比如sqlite3
、mysql-connector-python
或psycopg2
等。通过这些库,您可以创建一个连接对象,并使用该对象执行SQL查询。确保您提供正确的数据库凭据和连接字符串,以便成功连接。
在Python中检查数据库表是否存在的最佳实践是什么?
在Python中检查表是否存在的一种常见方法是使用SQL查询。例如,您可以查询information_schema.tables
(对于MySQL)或pg_catalog.pg_tables
(对于PostgreSQL)来确认表的存在。通过执行查询并检查返回的结果,您可以决定表是否存在。
如果表不存在,我该如何处理?
当表不存在时,您可以选择创建该表或处理错误。使用Python的异常处理机制,可以捕获执行查询时的错误,并根据需要创建表。例如,您可以使用SQL的CREATE TABLE
语句来定义新表的结构,并通过连接对象执行该命令。根据业务需求,选择适合的处理方式。
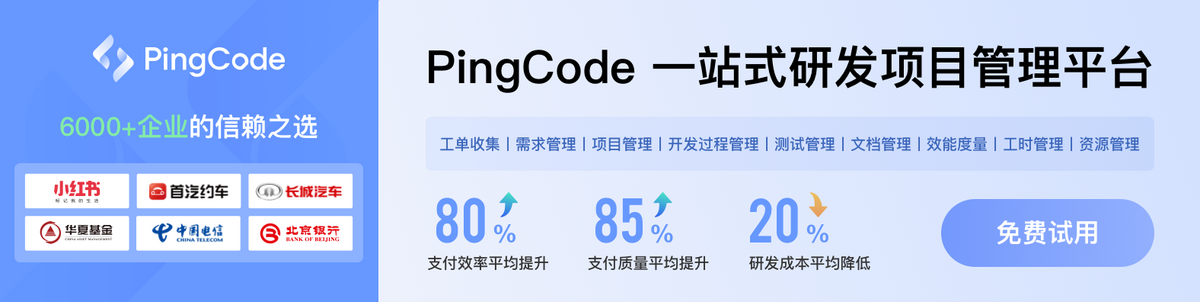